- Tina Chang 6
- NEWBIE
- 85 Points
- Member since 2017
- Salesforce Specialist
-
ChatterFeed
-
1Best Answers
-
0Likes Received
-
0Likes Given
-
8Questions
-
20Replies
Don't see standard SalesForce header and sidebar elements in Trailhead "Use Static Resources" unit
I am working on the Trailhead "Use Static Resources" unit. I see this in the instrutions - "Click Preview to open a preview of your page that you can look at while you make changes. A new window should open, showing the standard Salesforce page header and sidebar elements, and a short message from jQuery." But I don't see the standard Salesforce page header and sidebare elements, I just see a bare browser window with just the little bit of jQuery ouput from the exercise. I have noticed the same results in other training units. Is there some setup that I am missing? Thanks.
-
- PR Canegaly
- April 06, 2018
- Like
- 0
"System.AssertException: Assertion Failed" error in my test class
Hello, there! I've checked all similar posts but still wasn't able to solve the problem. This is a pretty simple trigger from Trailhead:
trigger RestrictContactByName on Contact (before insert, before update) { //check contacts prior to insert or update for invalid data For (Contact c : Trigger.New) { if(c.LastName == 'INVALIDNAME') { //invalidname is invalid c.AddError('The Last Name "'+c.LastName+'" is not allowed for DML.'); } } }This is my test class. The test class can get me 100% code coverage and the UI operation all works beautifully. The issue is the test result returned "System.AssertException: Assertion Failed" error at Line 15. Can anyone please explain to me why this is happening? Is it about Database opertion or DML statements? Thank you so much!
@isTest private class TestRestrictContactByName { @isTest static void TestLastNameIsvalid() { // Test data setup // Create a contact with a valid Last Name, and then try to insert/update it. Contact c = new Contact (FirstName='Kathy',LastName='Smith',Department='Technology'); insert c; // Perform test Test.startTest(); Database.SaveResult result = Database.insert(c, false); Test.stopTest(); // Verify // In this case the insert/update should have been processed. System.assert(result.isSuccess()); } @isTest static void TestLastNameIsNotInvalid() { // Test data setup // Create a contact with a Last Name of 'INVALIDNAME', and then try to insert/update it. Contact c2 = new Contact (FirstName='Joe',LastName='INVALIDNAME',Department='Finance'); insert c2; // Perform test Test.startTest(); Database.SaveResult result = Database.insert(c2, false); Test.stopTest(); // Verify // In this case the deletion should have been stopped by the trigger, // so verify that we got back an error. System.assert(!result.isSuccess()); System.assert(result.getErrors().size() > 0); System.assertEquals('The Last Name "INVALIDNAME" is not allowed for DML.', result.getErrors()[0].getMessage()); } }
-
- Tina Chang 6
- May 10, 2018
- Like
- 0
How to Write Test Class for Accounts with or without Opportunities?
Hello, I wrote the following test class for my Apex code "LastModifiedOppty" but it didn't work with code coverage 0%. Does anyone know how to fix this? My apologies I have just started the coding portion of my Salesforce knowledge base, so any advice would be much appreciated! Thank you!
@isTest private class LastModifiedOpptyTest { @isTest static void createAccountWithOpp() { // Accounts with Opportunities Account accts = new Account(); accts.Name = 'testAccount'; Insert accts; Opportunity opp = new Opportunity(); opp.Name = 'testOpportunity'; opp.StageName = 'Prospecting'; opp.CloseDate = Date.today(); opp.AccountId = accts.Id; opp.Amount = 100000; Insert opp; Test.startTest(); System.assertEquals(1, accts.Opportunities.Size()); Test.stopTest(); } @isTest static void createAccountWithoutOpp() { // Accounts without Opportunities Account accts2 = new Account(); accts2.Name = 'testAccount2'; Insert accts2; Test.startTest(); System.assertEquals(0, accts2.Opportunities.Size()); Test.stopTest(); } }Here's my Apex class "LastModifiedOppty":
public class LastModifiedOppty { public static void updateLastOpptyIdField() { // Retrieve the last modified opportunity's ID for each account. Account[] accountList = [SELECT Id, (SELECT Id, Amount from Opportunities ORDER BY LastModifiedDate DESC LIMIT 1) FROM Account]; // to access the opportunity related to individual account and pass the Id value on to the Last_Opportunity_ID__c field. // if the account does not have any opportunities, pass the value 'Without Oppty' to the Last_Opportunity_ID__c field. for(Account acct : accountList){ if(acct.Opportunities.size()>0){ Opportunity relatedOpp = acct.Opportunities[0]; acct.Last_Opportunity_ID__c = relatedOpp.Id; acct.Last_Opportunity_Amount__c = relatedOpp.Amount; } else if(acct.Opportunities.size()==0){ acct.Last_Opportunity_ID__c = 'Without Oppty'; } } update accountList; } }
-
- Tina Chang 6
- May 08, 2018
- Like
- 0
How to Write a Test Class for SOQL and Account Field Update?
Hello, I've just started to learn about Apex and I'm having difficulty writing a test class for the following Apex class.
The following Apex class has code to retrieve Account records and logic to iterate over a list of Account records and update the Description field. I learned this Apex class through Trailhead and wanted to write a test class for it but couldn't figure out how.
The following Apex class has code to retrieve Account records and logic to iterate over a list of Account records and update the Description field. I learned this Apex class through Trailhead and wanted to write a test class for it but couldn't figure out how.
public class OlderAccountsUtility { public static void updateOlderAccounts() { // Get the 5 oldest accounts Account[] oldAccounts = [SELECT Id, Description FROM Account ORDER BY CreatedDate ASC LIMIT 5]; // loop through them and update the Description field for (Account acct : oldAccounts) { acct.Description = 'Heritage Account'; } // save the change you made update oldAccounts; } }This is what I have written. Can anyone show me how to fix this Apex test unit so it works? Any help would be much appreciated!
@isTest private class OlderAccountsUtilityTest { @isTest static void createAccount() { List <Account> accts = new List<Account>(); for(integer i = 0; i<200; i++) { Account a = new Account(Name='testAccount'+'i'); accts.add(a); } insert accts; Test.StartTest(); List<Account> accList = [SELECT Id, Description FROM Account ORDER BY CreatedDate ASC LIMIT 5]; for (Account acct : accList) { acct.Description = 'Heritage Account'; } Test.StopTest(); System.AssertEquals( database.countquery('SELECT COUNT() FROM Account WHERE Description' = 'Heritage Account'), 5); } }
-
- Tina Chang 6
- March 13, 2018
- Like
- 0
Why mass uploads cancel out scores calculated by Apex code?
Hello, all,
We recently implemented this Apex code "Account Grading Method" (attached below) in our system. When an account record is created or edited, a process in the Process Builder calls the Apex code to calculate the account grade. It calculates the score of an account based on the input of a group of fields called Account Intelligence fields and returns the result to a number field called "Account Overall Grade".
The problem is whenever we mass update accounts using an upload (like API Data Loader) or via nightly API calls, the score of the Account Overall Grade get zeroed out, and they need to be re-triggered individually. The account fields we mass updated were not even related to the Account Intelligence fields. When we tried to mass update accounts in list view, the score zeroed out as well. For example, we took an account list view and selected a bunch of records to do a mass in-line editing of one of the Account Intelligence fields, the "Account Overall Grade" field zeroed out.
Any idea on how we can continue to be able to mass update accounts without this happening, or what the issue is? The person who developed this account grading process and the code had left. Any help would be much much appreciated!
My apologies this is a lot of code. Thank you for your patience.
Account Grading Method
We recently implemented this Apex code "Account Grading Method" (attached below) in our system. When an account record is created or edited, a process in the Process Builder calls the Apex code to calculate the account grade. It calculates the score of an account based on the input of a group of fields called Account Intelligence fields and returns the result to a number field called "Account Overall Grade".
The problem is whenever we mass update accounts using an upload (like API Data Loader) or via nightly API calls, the score of the Account Overall Grade get zeroed out, and they need to be re-triggered individually. The account fields we mass updated were not even related to the Account Intelligence fields. When we tried to mass update accounts in list view, the score zeroed out as well. For example, we took an account list view and selected a bunch of records to do a mass in-line editing of one of the Account Intelligence fields, the "Account Overall Grade" field zeroed out.
Any idea on how we can continue to be able to mass update accounts without this happening, or what the issue is? The person who developed this account grading process and the code had left. Any help would be much much appreciated!
My apologies this is a lot of code. Thank you for your patience.
Account Grading Method
public with sharing class AccountGradingMethod { public static void AccountGrading(Set<String> setAcctGradingIds) { List<Account> lstAGS = new List<Account>(); List<Account> lstUpdateAcct = new List<Account>(); Map<String, Field_Value__mdt> mapFlds = new Map<String, Field_Value__mdt>(); String strSOQLAcct; String strSOQLAcctGrade; Set<String> setFlds = new Set<String>(); Set<String> setFldName = new Set<String>(); String strScore; Decimal maxPossScore = 0; Decimal numberFlds = 0; strSOQLAcctGrade = 'SELECT Id'; for(Field_Value__mdt fn:[SELECT Id, Field_Score_or_Multiplier__c, Picklist_API_Value__c, Use_Greater_then_or_Less_then__c, Field_Name__r.Field_API_Name__c, Field_Name__r.Object__c, Field_Name__r.Type_of_Field__c, Greater_then__c, Less_then__c FROM Field_Value__mdt]){ String mapKey; setFldName.add(fn.Field_Name__r.Field_API_Name__c); if(fn.Field_Name__r.Object__c == 'Account'){ setFlds.add(fn.Field_Name__r.Field_API_Name__c); } if(fn.Use_Greater_then_or_Less_then__c){ mapKey = fn.Field_Name__r.Field_API_Name__c + '-' + fn.Greater_then__c + '-' + fn.Less_then__c; } else{ mapKey = fn.Field_Name__r.Field_API_Name__c + '-' + fn.Picklist_API_Value__c.deleteWhitespace(); } mapFlds.put(mapKey, fn); } for(String f:setFlds){ strSOQLAcctGrade += ', ' + f; } numberFlds = setFlds.size(); strSOQLAcctGrade += ' FROM Account WHERE Id in :setAcctGradingIds'; System.debug('strSOQLAcctGrade: ' + strSOQLAcctGrade); lstAGS = Database.query(strSOQLAcctGrade); strScore = System.Label.Max_Possible_Score; maxPossScore = Decimal.valueOf(strScore); for(Account ags:lstAGS){ Decimal subTotal = 0; Decimal multiplier = 1; Decimal decTotal = 0; Decimal ecommTotal = 0; Decimal ecommMin = 0; Decimal ecommMax = 0; Decimal fldsPopulated = 0; Decimal precentComplete = 0; for(Field_Value__mdt fldVal:mapFlds.values()){ String value; Decimal score = 0; Boolean skip = false; String fld = fldVal.Field_Name__r.Field_API_Name__c; String strKey; Decimal decValue = 0; if(setFldName.contains(fld)){ if(fldVal.Field_Name__r.Object__c == 'Account'){ value = String.valueOf(ags.get(fld)); if(value != '' && value != 'null' && value != null){ value = value.deleteWhitespace(); if(fldVal.Use_Greater_then_or_Less_then__c){ decValue = Decimal.valueOf(value); System.debug('decValue: ' + decValue); if(fldVal.Greater_then__c != null && fldVal.Less_then__c != null){ if(fldVal.Greater_then__c > decValue && fldVal.Less_then__c < decValue){ System.debug('if 1 fldVal.Less_then__c: ' + fldVal.Less_then__c); strKey = fld + '-' + fldVal.Greater_then__c + '-' + fldVal.Less_then__c; } else{ skip = true; } } if(fldVal.Greater_then__c == null && fldVal.Less_then__c != null){ if(fldVal.Less_then__c > decValue){ System.debug('if 2 fldVal.Less_then__c: ' + fldVal.Less_then__c); strKey = fld + '-' + fldVal.Greater_then__c + '-' + fldVal.Less_then__c; } else{ skip = true; } } if(fldVal.Greater_then__c != null && fldVal.Less_then__c == null){ System.debug('if 3 fldVal.Greater_then__c : ' + fldVal.Greater_then__c ); if(fldVal.Greater_then__c < decValue){ strKey = fld + '-' + fldVal.Greater_then__c + '-' + fldVal.Less_then__c; } else{ skip = true; } } } else if (!fldVal.Use_Greater_then_or_Less_then__c){ strKey = fldVal.Field_Name__r.Field_API_Name__c + '-' + value; } if(!String.isBlank(strKey) && mapFlds.get(strKey) != null){ setFldName.remove(fld); score = mapFlds.get(strKey).Field_Score_or_Multiplier__c; } if(score != null){ if(fld.startsWithIgnoreCase('e-commerce solutions')){ skip = true; ecommTotal = ecommTotal + score; if(ecommMax<score){ ecommMax = score; } if(ecommMin>score){ ecommMin = score; } } if(fldVal.Field_Name__r.Type_of_Field__c == 'Score' && !skip){ System.debug('fld: ' + fld); System.debug('Score: ' + score); subTotal = subTotal + score; } if(fldVal.Field_Name__r.Type_of_Field__c == 'Multiplier' && !skip){ System.debug('fld: ' + fld); System.debug('multiplier: ' + score); multiplier = multiplier * score; } } } else{ fldsPopulated = fldsPopulated + 1; setFldName.remove(fld); } } } } if(ecommTotal>=0){ subTotal = subTotal + ecommMax; } else{ subTotal = subTotal + ecommMin; } if(fldsPopulated == numberFlds){ precentComplete = 100; } else{ precentComplete = ((numberFlds - fldsPopulated)/numberFlds)*100; } System.debug('subtotal: ' + subTotal); subTotal = (subTotal/maxPossScore)*100; System.debug('subtotal after: ' + subTotal); System.debug('multiplier: ' + multiplier); decTotal = subTotal*multiplier; System.debug('decTotal: ' + decTotal ); Account a = new Account(); a.Id = ags.Id; a.Account_Overall_Grade__c = decTotal; a.Percent_Complete__c = precentComplete; lstUpdateAcct.add(a); } if(lstUpdateAcct.size()>0){ update lstUpdateAcct; } } }
-
- Tina Chang 6
- January 12, 2018
- Like
- 0
How to Fix this Apex Trigger? "The flow tried to update these records: null. This error occurred: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY: AccountTrigger"
Hello, there!
We have these two processes on Accounts in Process Builder -- one that updates a custom field called "Territory" based on the Billing State Code or the Billing City and/or the Region fields. The other that updates a custom field called "Region" based on the Billing Country field. Both processes have recently been getting the same error notifications as follows:
"The flow tried to update these records: null. This error occurred: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY: AccountTrigger: System.LimitException: Apex CPU time limit exceeded. For details, see API Exceptions."
Looks like it is being caused via the Process Builder but I suspect the root cause is the Apex here. Can you please look into this and see if the code needs to be made more efficient or fixed? I've attached the complete Apex trigger code and its test class below for your reference.
Please let me know if you require any further information. Your help will be greatly appreciated!
(My apologies this is a lof of code...)
AccountTrigger
AccountTriggerHandler
AccountTriggerHandlerTest
We have these two processes on Accounts in Process Builder -- one that updates a custom field called "Territory" based on the Billing State Code or the Billing City and/or the Region fields. The other that updates a custom field called "Region" based on the Billing Country field. Both processes have recently been getting the same error notifications as follows:
"The flow tried to update these records: null. This error occurred: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY: AccountTrigger: System.LimitException: Apex CPU time limit exceeded. For details, see API Exceptions."
Looks like it is being caused via the Process Builder but I suspect the root cause is the Apex here. Can you please look into this and see if the code needs to be made more efficient or fixed? I've attached the complete Apex trigger code and its test class below for your reference.
Please let me know if you require any further information. Your help will be greatly appreciated!
(My apologies this is a lof of code...)
AccountTrigger
/******************************************************************************* * AccountTrigger * @Description: Master Trigger for Accounts, which fires on ALL events to * control the order in which trigger actions occur. Follows the * super trigger best practice from Salesforce: * * https://developer.salesforce.com/page/Trigger_Frameworks_and_Apex_Trigger_Best_Practices * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ trigger AccountTrigger on Account (before insert, before update) { // Determine what action is // occurring and when, and // pass the correct context // variables to the Trigger // Handler class where all the // actions will take place if (Trigger.isBefore && Trigger.isInsert) { // Before Insert AccountTriggerHandler.beforeInsert(Trigger.new, Trigger.newMap); } else if (Trigger.isBefore && Trigger.isUpdate) { // Before Update AccountTriggerHandler.beforeUpdate(Trigger.new, Trigger.newMap, Trigger.old, Trigger.oldMap); } /* FOR FRAMEWORK SETUP ONLY. NO ACTIONS ARE TAKEN AT THIS TIME else if (Trigger.isBefore && Trigger.isDelete) { // Before Delete AccountTriggerHandler.beforeDelete(Trigger.new, Trigger.newMap, Trigger.old, Trigger.oldMap); } else if (Trigger.isAfter && Trigger.isInsert) { // After Insert AccountTriggerHandler.afterInsert(Trigger.new, Trigger.newMap); } else if (Trigger.isAfter && Trigger.isUpdate) { // After Update AccountTriggerHandler.afterUpdate(Trigger.new, Trigger.newMap, Trigger.old, Trigger.oldMap); } else if (Trigger.isAfter && Trigger.isDelete) { // After Delete AccountTriggerHandler.afterDelete(Trigger.new, Trigger.old); } else if (Trigger.isAfter && Trigger.isUnDelete) { // After UnDelete AccountTriggerHandler.afterUnDelete(Trigger.new, Trigger.old); } */ }
AccountTriggerHandler
/******************************************************************************* * AccountTriggerHandler * @Description: Class for handling all Account Trigger functionality. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ public with sharing class AccountTriggerHandler { /******************************************************************************* * beforeInsert * @Description: Method for handling all "BeforeInsert" functionality. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ public static void beforeInsert(List<Account> a_newRecordList, Map<Id,Account> a_newRecordMap) { System.debug('*** START: AccountTriggerHandler.beforeInsert()'); // Iterate through the new Account // records and grab their SIC Code // (if applicable). Set<String> sicCodeSet = new Set<String>(); for (Account a : a_newRecordList) { if (a.Sic != null && a.Sic != '') { sicCodeSet.add(a.Sic); } } // Do we have a Set of SIC Codes? if (!sicCodeSet.isEmpty()) { // Re-Iterate through the new Account // records and set their reference to // a SIC_Code__c record (if applicable). Map<String,Id> sicCodeIdMap = getSICCodeMap(sicCodeSet); for (Account a : a_newRecordList) { if (sicCodeIdMap.containsKey(a.Sic)) { a.SIC_Code__c = sicCodeIdMap.get(a.Sic); } } } System.debug('*** END: AccountTriggerHandler.beforeInsert()'); } // End Method: beforeInsert() /******************************************************************************* * beforeUpdate * @Description: Method for handling all "Before Update" functionality. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ public static void beforeUpdate(List<Account> a_newRecordList, Map<Id,Account> a_newRecordMap, List<Account> a_oldRecordList, Map<Id,Account> a_oldRecordMap) { System.debug('*** START: AccountTriggerHandler.beforeUpdate()'); // Iterate through the updated Account // records and grab their SIC Codes // (if applicable). Set<String> sicCodeSet = new Set<String>(); for (Account a : a_newRecordList) { // Was the SIC Code changed? if (a.Sic != a_oldRecordMap.get(a.Id).Sic) { // If the SIC Code was removed, clear // the Lookup field. if (a.Sic == null || a.Sic == '') { a.SIC_Code__c = null; } else { sicCodeSet.add(a.Sic); } } } // Do we have a Set of SIC Codes? if (!sicCodeSet.isEmpty()) { // Re-Iterate through the updated Account // records and set their reference to // a SIC_Code__c record (if applicable). Map<String,Id> sicCodeIdMap = getSICCodeMap(sicCodeSet); for (Account a : a_newRecordList) { if (sicCodeIdMap.containsKey(a.Sic)) { a.SIC_Code__c = sicCodeIdMap.get(a.Sic); } } } System.debug('*** END: AccountTriggerHandler.beforeUpdate()'); } // End Method: beforeUpdate() /******************************************************************************* * getSICCodeMap * @Description: Method for returning a Map of SIC Code-to-SIC Code Id. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Paramaters: Set<String> a_sicCodeSet * @Return: Map<String,Id> returnMap * * @Updates: N/A *******************************************************************************/ private static Map<String, Id> getSICCodeMap(Set<String> a_sicCodeSet) { System.debug('*** START: AccountTriggerHandler.getSICCodeMap()'); Map<String,Id> returnMap = new Map<String,Id>(); // Iterate through the SIC Code records // associated with the SIC Codes on the // Account records that have been // modified. for (SIC_Code__c sc : [SELECT Id, Name FROM SIC_Code__c WHERE Name IN :a_sicCodeSet]) { // This SIC Code is associated with an // Account that has been updated, so // add it to the Map being returned. returnMap.put(sc.Name, sc.Id); } System.debug('*** returnMap: ' + returnMap); System.debug('*** END: AccountTriggerHandler.getSICCodeMap()'); return returnMap; } // End Method: getSICCodeMap() }
AccountTriggerHandlerTest
/******************************************************************************* * AccountTriggerHandlerTest * @Description: Test class for AccountTriggerHandler class. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ @isTest public class AccountTriggerHandlerTest { private static final String sicCode_Name = '01110000'; private static final String sicCode_Description = 'WHEAT'; /******************************************************************************* * TestDataSetup * @Description: Method to create test data to be used throughout the class. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ @testSetup static void TestDataSetup() { System.debug('*** START: AccountTriggerHandlerTest.test_beforeInsert()'); Id sysAdminProfileId = [SELECT Id FROM Profile WHERE Name = 'System Administrator'].Id; Id execTeamRoleId = [SELECT Id FROM UserRole WHERE DeveloperName = 'ExecutiveTeam'].Id; // Create System Administrator User. User adminUser = new User(); adminUser.LastName = 'dr' + Crypto.getRandomInteger(); adminUser.Email = adminUser.LastName + '@drtest.com'; adminUser.UserName = adminUser.Email; adminUser.Alias = String.valueOf(adminUser.LastName.SubString(0,8)); adminUser.ProfileId = sysAdminProfileId; adminUser.UserRoleId = execTeamRoleId; adminUser.LocaleSidKey = 'en_US'; adminUser.LanguageLocaleKey = 'en_US'; adminUser.TimeZoneSidKey = 'America/Los_Angeles'; adminUser.EmailEncodingKey = 'UTF-8'; insert(adminUser); system.runAs(adminUser) { SIC_Code__c sicCode = new SIC_Code__c(); sicCode.Name = sicCode_Name; sicCode.Description__c = sicCode_Description; insert(sicCode); } } /******************************************************************************* * test_beforeInsert * @Description: Method to test the "beforeInsert" method. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ public static testMethod void test_beforeInsert() { System.debug('*** START: AccountTriggerHandlerTest.test_beforeInsert()'); Id sysAdminProfileId = [SELECT Id FROM Profile WHERE Name = 'System Administrator'].Id; Id execTeamRoleId = [SELECT Id FROM UserRole WHERE DeveloperName = 'ExecutiveTeam'].Id; // Create System Administrator User. User adminUser = new User(); adminUser.LastName = 'dr' + Crypto.getRandomInteger(); adminUser.Email = adminUser.LastName + '@drtest.com'; adminUser.UserName = adminUser.Email; adminUser.Alias = String.valueOf(adminUser.LastName.SubString(0,8)); adminUser.ProfileId = sysAdminProfileId; adminUser.UserRoleId = execTeamRoleId; adminUser.LocaleSidKey = 'en_US'; adminUser.LanguageLocaleKey = 'en_US'; adminUser.TimeZoneSidKey = 'America/Los_Angeles'; adminUser.EmailEncodingKey = 'UTF-8'; insert(adminUser); system.runAs(adminUser) { Test.startTest(); Account newAccount = new Account(); newAccount.Name = 'Test Account'; newAccount.CurrencyIsoCode = 'USD'; newAccount.Website = 'http://www.somewhere.com/'; newAccount.Vertical_Market__c = 'Marketplace'; newAccount.E_Commerce_Solution_US__c = 'Amazon'; newAccount.AlexaRating__c = '0-10K'; newAccount.Sic = sicCode_Name; insert(newAccount); Test.stopTest(); List<Account> newAccountList = [SELECT Id, SICDescription__c FROM Account WHERE Id = :newAccount.Id AND SICDescription__c = :sicCode_Description]; //System.assert(newAccountList.size() > 0, 'Account was NOT created with SIC Code reference'); } System.debug('*** END: AccountTriggerHandlerTest.test_beforeInsert()'); } // End Method: test_afterInsert() /******************************************************************************* * test_beforeUpdate * @Description: Method to test the "beforeUpdate" method. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ public static testMethod void test_beforeUpdate() { System.debug('*** START: AccountTriggerHandlerTest.test_beforeUpdate()'); Id sysAdminProfileId = [SELECT Id FROM Profile WHERE Name = 'System Administrator'].Id; Id execTeamRoleId = [SELECT Id FROM UserRole WHERE DeveloperName = 'ExecutiveTeam'].Id; // Create System Administrator User. User adminUser = new User(); adminUser.LastName = 'dr' + Crypto.getRandomInteger(); adminUser.Email = adminUser.LastName + '@drtest.com'; adminUser.UserName = adminUser.Email; adminUser.Alias = String.valueOf(adminUser.LastName.SubString(0,8)); adminUser.ProfileId = sysAdminProfileId; adminUser.UserRoleId = execTeamRoleId; adminUser.LocaleSidKey = 'en_US'; adminUser.LanguageLocaleKey = 'en_US'; adminUser.TimeZoneSidKey = 'America/Los_Angeles'; adminUser.EmailEncodingKey = 'UTF-8'; insert(adminUser); system.runAs(adminUser) { Test.startTest(); Account newAccount = new Account(); newAccount.Name = 'Test Account'; newAccount.CurrencyIsoCode = 'USD'; newAccount.Website = 'http://www.somewhere.com/'; newAccount.Vertical_Market__c = 'Marketplace'; newAccount.E_Commerce_Solution_US__c = 'Amazon'; newAccount.AlexaRating__c = '0-10K'; insert(newAccount); List<Account> newAccountList = [SELECT Id, SICDescription__c FROM Account WHERE Id = :newAccount.Id AND SICDescription__c = :sicCode_Description]; System.assert(newAccountList.size() == 0, 'Account was created with SIC Code Reference'); newAccount.Sic = sicCode_Name; update(newAccount); List<Account> updatedAccountList = [SELECT Id, SICDescription__c FROM Account WHERE Id = :newAccount.Id AND SICDescription__c = :sicCode_Description]; //System.assert(updatedAccountList.size() > 0, 'Account was NOT updated with SIC Code Reference'); Test.stopTest(); } System.debug('*** END: AccountTriggerHandlerTest.test_beforeUpdate()'); } // End Method: test_beforeUpdate() }
-
- Tina Chang 6
- January 04, 2018
- Like
- 0
Apex "Else If" statement won't hit. Please help! Thank you!
Hello, there! The highlighted "if" and "else if" statement for the "Percent Complete" field below never execute. Only the "else" statement would work as expected. Does anyone know how to re-write to make it work? Any help will be greatly appreciated!!
Here's the complete Apex Class. Everything else works except for the if statement for the Percent Complete field mentioned above. Thanks in advance for your suggestions!
if(fldsPopulated == numberFlds){ PrecentComplete = 100; }else if(fldsPopulated == 0){ PrecentComplete = 0; }else{ precentComplete = ((numberFlds - fldsPopulated)/numberFlds)*100; }
Here's the complete Apex Class. Everything else works except for the if statement for the Percent Complete field mentioned above. Thanks in advance for your suggestions!
public with sharing class AccountGradingMethod { public static void AccountGrading(Set<String> setAcctGradingIds) { List<Account> lstAGS = new List<Account>(); List<Account> lstUpdateAcct = new List<Account>(); Map<String, Field_Value__mdt> mapFlds = new Map<String, Field_Value__mdt>(); String strSOQLAcct; String strSOQLAcctGrade; Set<String> setFlds = new Set<String>(); Set<String> setFldName = new Set<String>(); String strScore; Decimal maxPossScore = 0; Decimal numberFlds = 0; strSOQLAcctGrade = 'SELECT Id'; for(Field_Value__mdt fn:[SELECT Id, Field_Score_or_Multiplier__c, Picklist_API_Value__c, Use_Greater_then_or_Less_then__c, Field_Name__r.Field_API_Name__c, Field_Name__r.Object__c, Field_Name__r.Type_of_Field__c, Greater_then__c, Less_then__c FROM Field_Value__mdt]){ String mapKey; setFldName.add(fn.Field_Name__r.Field_API_Name__c); if(fn.Field_Name__r.Object__c == 'Account'){ setFlds.add(fn.Field_Name__r.Field_API_Name__c); } if(fn.Use_Greater_then_or_Less_then__c){ mapKey = fn.Field_Name__r.Field_API_Name__c + '-' + fn.Greater_then__c + '-' + fn.Less_then__c; } else{ mapKey = fn.Field_Name__r.Field_API_Name__c + '-' + fn.Picklist_API_Value__c.deleteWhitespace(); } mapFlds.put(mapKey, fn); } for(String f:setFlds){ strSOQLAcctGrade += ', ' + f; } numberFlds = setFlds.size(); strSOQLAcctGrade += ' FROM Account WHERE Id in :setAcctGradingIds'; System.debug('strSOQLAcctGrade: ' + strSOQLAcctGrade); lstAGS = Database.query(strSOQLAcctGrade); strScore = System.Label.Max_Possible_Score; maxPossScore = Decimal.valueOf(strScore); for(Account ags:lstAGS){ Decimal subTotal = 0; Decimal multiplier = 1; Decimal decTotal = 0; Decimal ecommTotal = 0; Decimal ecommMin = 0; Decimal ecommMax = 0; Decimal fldsPopulated = 0; Decimal precentComplete = 0; for(Field_Value__mdt fldVal:mapFlds.values()){ String value; Decimal score = 0; Boolean skip = false; String fld = fldVal.Field_Name__r.Field_API_Name__c; String strKey; Decimal decValue = 0; if(setFldName.contains(fld)){ if(fldVal.Field_Name__r.Object__c == 'Account'){ value = String.valueOf(ags.get(fld)); if(value != '' && value != 'null' && value != null){ value = value.deleteWhitespace(); if(fldVal.Use_Greater_then_or_Less_then__c){ decValue = Decimal.valueOf(value); System.debug('decValue: ' + decValue); if(fldVal.Greater_then__c != null && fldVal.Less_then__c != null){ if(fldVal.Greater_then__c > decValue && fldVal.Less_then__c < decValue){ System.debug('if 1 fldVal.Less_then__c: ' + fldVal.Less_then__c); strKey = fld + '-' + fldVal.Greater_then__c + '-' + fldVal.Less_then__c; } else{ skip = true; } } if(fldVal.Greater_then__c == null && fldVal.Less_then__c != null){ if(fldVal.Less_then__c > decValue){ System.debug('if 2 fldVal.Less_then__c: ' + fldVal.Less_then__c); strKey = fld + '-' + fldVal.Greater_then__c + '-' + fldVal.Less_then__c; } else{ skip = true; } } if(fldVal.Greater_then__c != null && fldVal.Less_then__c == null){ System.debug('if 3 fldVal.Greater_then__c : ' + fldVal.Greater_then__c ); if(fldVal.Greater_then__c < decValue){ strKey = fld + '-' + fldVal.Greater_then__c + '-' + fldVal.Less_then__c; } else{ skip = true; } } } else if (!fldVal.Use_Greater_then_or_Less_then__c){ strKey = fldVal.Field_Name__r.Field_API_Name__c + '-' + value; } if(!String.isBlank(strKey) && mapFlds.get(strKey) != null){ setFldName.remove(fld); score = mapFlds.get(strKey).Field_Score_or_Multiplier__c; } if(score != null){ if(fld.startsWithIgnoreCase('e-commerce solutions')){ skip = true; ecommTotal = ecommTotal + score; if(ecommMax<score){ ecommMax = score; } if(ecommMin>score){ ecommMin = score; } } if(fldVal.Field_Name__r.Type_of_Field__c == 'Score' && !skip){ System.debug('fld: ' + fld); System.debug('Score: ' + score); subTotal = subTotal + score; } if(fldVal.Field_Name__r.Type_of_Field__c == 'Multiplier' && !skip){ System.debug('fld: ' + fld); System.debug('multiplier: ' + score); multiplier = multiplier * score; } } } else{ fldsPopulated = fldsPopulated + 1; setFldName.remove(fld); } } } } if(ecommTotal>=0){ subTotal = subTotal + ecommMax; } else{ subTotal = subTotal + ecommMin; } if(fldsPopulated == numberFlds){ PrecentComplete = 100; }else if(fldsPopulated == 0){ PrecentComplete = 0; }else{ precentComplete = ((numberFlds - fldsPopulated)/numberFlds)*100; } System.debug('subtotal: ' + subTotal); subTotal = (subTotal/maxPossScore)*100; System.debug('subtotal after: ' + subTotal); System.debug('multiplier: ' + multiplier); decTotal = subTotal*multiplier; System.debug('decTotal: ' + decTotal ); Account a = new Account(); a.Id = ags.Id; a.Account_Overall_Grade__c = decTotal; a.Percent_Complete__c = precentComplete; a.LastScoredDate__c = System.today(); lstUpdateAcct.add(a); } if(lstUpdateAcct.size()>0){ update lstUpdateAcct; } } }
-
- Tina Chang 6
- December 11, 2017
- Like
- 0
How to Fix this Apex Test Class?
Hello! I have to deactivate a Trigger as it is blocking a deployment.
I deactivated the Trigger in the sandbox, created a Change Set, included the Trigger in the change set and deployed the same into the Production, but it wasn't able to pass the Apex Test.
This is the error message that I got:
I know the problem is with this code "System.assert(false);" but I am not a developer so I don't know how to fix it. I have searched around for solutions but it is way beyond my knowledge.
Can anyone kindly help and let me know how to fix this Apex Test Class? It would be much much appreciated!
The Apex Trigger
The Apex Test Class
Tina
Sincerely,
Tina
I deactivated the Trigger in the sandbox, created a Change Set, included the Trigger in the change set and deployed the same into the Production, but it wasn't able to pass the Apex Test.
This is the error message that I got:
- System.Exception: Assertion Failed
- Stack Trace: Class.test_updatecontactrolecount.testcreateopptywithconditionandrole: line 38, column 1
I know the problem is with this code "System.assert(false);" but I am not a developer so I don't know how to fix it. I have searched around for solutions but it is way beyond my knowledge.
Can anyone kindly help and let me know how to fix this Apex Test Class? It would be much much appreciated!
The Apex Trigger
trigger updatecontactrolecount on Opportunity (before insert, before update) { Boolean isPrimary; Integer iCount; Map<String, Opportunity> oppty_con = new Map<String, Opportunity>();//check if the contact role is needed and add it to the oppty_con map for (Integer i = 0; i < Trigger.new.size(); i++) { oppty_con.put(Trigger.new[i].id, Trigger.new[i]); } isPrimary = False; for (List<OpportunityContactRole> oppcntctrle :[select OpportunityId from OpportunityContactRole where (OpportunityContactRole.IsPrimary = True and OpportunityContactRole.OpportunityId in :oppty_con.keySet())]) { if (oppcntctrle .Size() >0) { isPrimary = True; } } iCount = 0; for (List<OpportunityContactRole> oppcntctrle2 : [select OpportunityId from OpportunityContactRole where (OpportunityContactRole.OpportunityId in :oppty_con.keySet())])//Query for Contact Roles { if (oppcntctrle2 .Size()>0) { iCount= oppcntctrle2 .Size(); } } for (Opportunity Oppty : system.trigger.new) //Check if roles exist in the map or contact role isn't required { Oppty.Number_of_Contacts_Roles_Assigned__c = iCount; Oppty.Primary_Contact_Assigned__c =isPrimary; } }
The Apex Test Class
public class test_updatecontactrolecount { public static testMethod void testcreateopptywithconditionandrole() { //Insert Opportunities try { Opportunity Oppty = new Opportunity(Name='Oppty_test1',StageName='Stage 1 - Unqualified Prospect',Type ='New Business', CloseDate=System.Today()); insert Oppty; // insert contact Contact[] cont = new Contact[] { new Contact(LastName = 'testcontact1'), new Contact(LastName = 'testcontact2') }; insert cont; // insert contact role OpportunityContactRole [] ocr = new OpportunityContactRole[] { new OpportunityContactRole(Role ='Business User',OpportunityId=Oppty.id ,Contactid = cont[0].id ,Isprimary = True), new OpportunityContactRole(Role ='Business User',OpportunityId=Oppty.id ,Contactid = cont[0].id ,Isprimary = False) }; insert ocr; Oppty.StageName = 'Stage 3 - Eval Request'; //Update opportunity Test.StartTest(); update Oppty; Test.StopTest(); Oppty =[SELECT Number_of_Contacts_Roles_Assigned__c,Primary_Contact_Assigned__c FROM Opportunity WHERE Id = :Oppty.Id]; system.assert (Oppty.Number_of_Contacts_Roles_Assigned__c == 2); system.assert (Oppty.Primary_Contact_Assigned__c == True); } catch (System.DmlException e) { System.assert(false); } } }Sincerely,
Tina
Sincerely,
Tina
-
- Tina Chang 6
- September 08, 2017
- Like
- 0
After embedding a Flow in Visualforce Page, how to make a Visualforce Page form display only for certain screen of Flow?
Hello, everyone! I had a flow embedded in a Visualforce Page. And then I created a apex form for users to upload the files. My question is: is it possible to display this Upload an Attachment form area for only the 1st Intro Screen in the flow session? That is, I don't want the users to see the Upload an Attachment section during the flow session except for the 1st Intro Screen - STEP 1 as captured in the image below. Is there a way for me to hide that section for the rest of the steps? Any suggestions and help would be greatly appreciated!! :)
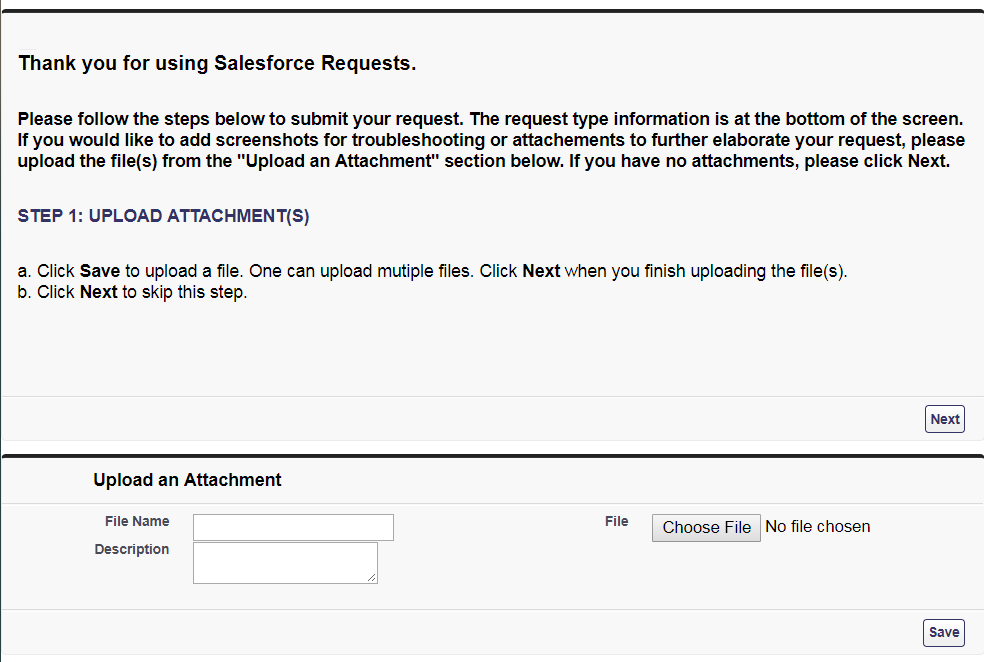
The Visualforce Page:
Following is the Apex Class: AttachmentUploadController
The Visualforce Page:
<apex:page sidebar="false" showHeader="false" title="Salesforce Requests" controller="AttachmentUploadController"> <flow:interview name="Salesforce_Request_System" finishLocation="{!URLFOR('/home/home.jsp')}" buttonLocation="bottom" buttonStyle="color:#333366; background-color:#fed; border:1px solid;"/> <apex:form enctype="multipart/form-data"> <apex:pageMessages /> <apex:pageBlock title="Upload an Attachment" > <apex:pageBlockButtons location="bottom"> <apex:commandButton action="{!upload}" value="Save" style="color:#333366; background-color:#fed; border:1px solid;"/> </apex:pageBlockButtons> <apex:pageBlockSection showHeader="false" columns="2" id="block1"> <apex:pageBlockSectionItem > <apex:outputLabel value="File Name" for="fileName"/> <apex:inputText value="{!attachment.name}" id="fileName"/> </apex:pageBlockSectionItem> <apex:pageBlockSectionItem > <apex:outputLabel value="File" for="file"/> <apex:inputFile value="{!attachment.body}" filename="{!attachment.name}" id="file"/> </apex:pageBlockSectionItem> <apex:pageBlockSectionItem > <apex:outputLabel value="Description" for="description"/> <apex:inputTextarea value="{!attachment.description}" id="description"/> </apex:pageBlockSectionItem> </apex:pageBlockSection> </apex:pageBlock> </apex:form> </apex:page>
Following is the Apex Class: AttachmentUploadController
public with sharing class AttachmentUploadController { public Attachment attachment { get { if (attachment == null) attachment = new Attachment(); return attachment; } set; } public PageReference upload() { attachment.OwnerId = UserInfo.getUserId(); attachment.ParentId = '500S0000008lP0o'; attachment.IsPrivate = true; try { insert attachment; } catch (DMLException e) { ApexPages.addMessage(new ApexPages.message(ApexPages.severity.ERROR,'Error uploading attachment')); return null; } finally { attachment = new Attachment(); } ApexPages.addMessage(new ApexPages.message(ApexPages.severity.INFO,'Attachment uploaded successfully')); return null; } }
-
- Tina Chang 6
- July 20, 2017
- Like
- 0
"System.AssertException: Assertion Failed" error in my test class
Hello, there! I've checked all similar posts but still wasn't able to solve the problem. This is a pretty simple trigger from Trailhead:
trigger RestrictContactByName on Contact (before insert, before update) { //check contacts prior to insert or update for invalid data For (Contact c : Trigger.New) { if(c.LastName == 'INVALIDNAME') { //invalidname is invalid c.AddError('The Last Name "'+c.LastName+'" is not allowed for DML.'); } } }This is my test class. The test class can get me 100% code coverage and the UI operation all works beautifully. The issue is the test result returned "System.AssertException: Assertion Failed" error at Line 15. Can anyone please explain to me why this is happening? Is it about Database opertion or DML statements? Thank you so much!
@isTest private class TestRestrictContactByName { @isTest static void TestLastNameIsvalid() { // Test data setup // Create a contact with a valid Last Name, and then try to insert/update it. Contact c = new Contact (FirstName='Kathy',LastName='Smith',Department='Technology'); insert c; // Perform test Test.startTest(); Database.SaveResult result = Database.insert(c, false); Test.stopTest(); // Verify // In this case the insert/update should have been processed. System.assert(result.isSuccess()); } @isTest static void TestLastNameIsNotInvalid() { // Test data setup // Create a contact with a Last Name of 'INVALIDNAME', and then try to insert/update it. Contact c2 = new Contact (FirstName='Joe',LastName='INVALIDNAME',Department='Finance'); insert c2; // Perform test Test.startTest(); Database.SaveResult result = Database.insert(c2, false); Test.stopTest(); // Verify // In this case the deletion should have been stopped by the trigger, // so verify that we got back an error. System.assert(!result.isSuccess()); System.assert(result.getErrors().size() > 0); System.assertEquals('The Last Name "INVALIDNAME" is not allowed for DML.', result.getErrors()[0].getMessage()); } }
- Tina Chang 6
- May 10, 2018
- Like
- 0
How to Write Test Class for Accounts with or without Opportunities?
Hello, I wrote the following test class for my Apex code "LastModifiedOppty" but it didn't work with code coverage 0%. Does anyone know how to fix this? My apologies I have just started the coding portion of my Salesforce knowledge base, so any advice would be much appreciated! Thank you!
@isTest private class LastModifiedOpptyTest { @isTest static void createAccountWithOpp() { // Accounts with Opportunities Account accts = new Account(); accts.Name = 'testAccount'; Insert accts; Opportunity opp = new Opportunity(); opp.Name = 'testOpportunity'; opp.StageName = 'Prospecting'; opp.CloseDate = Date.today(); opp.AccountId = accts.Id; opp.Amount = 100000; Insert opp; Test.startTest(); System.assertEquals(1, accts.Opportunities.Size()); Test.stopTest(); } @isTest static void createAccountWithoutOpp() { // Accounts without Opportunities Account accts2 = new Account(); accts2.Name = 'testAccount2'; Insert accts2; Test.startTest(); System.assertEquals(0, accts2.Opportunities.Size()); Test.stopTest(); } }Here's my Apex class "LastModifiedOppty":
public class LastModifiedOppty { public static void updateLastOpptyIdField() { // Retrieve the last modified opportunity's ID for each account. Account[] accountList = [SELECT Id, (SELECT Id, Amount from Opportunities ORDER BY LastModifiedDate DESC LIMIT 1) FROM Account]; // to access the opportunity related to individual account and pass the Id value on to the Last_Opportunity_ID__c field. // if the account does not have any opportunities, pass the value 'Without Oppty' to the Last_Opportunity_ID__c field. for(Account acct : accountList){ if(acct.Opportunities.size()>0){ Opportunity relatedOpp = acct.Opportunities[0]; acct.Last_Opportunity_ID__c = relatedOpp.Id; acct.Last_Opportunity_Amount__c = relatedOpp.Amount; } else if(acct.Opportunities.size()==0){ acct.Last_Opportunity_ID__c = 'Without Oppty'; } } update accountList; } }
- Tina Chang 6
- May 08, 2018
- Like
- 0
Don't see standard SalesForce header and sidebar elements in Trailhead "Use Static Resources" unit
I am working on the Trailhead "Use Static Resources" unit. I see this in the instrutions - "Click Preview to open a preview of your page that you can look at while you make changes. A new window should open, showing the standard Salesforce page header and sidebar elements, and a short message from jQuery." But I don't see the standard Salesforce page header and sidebare elements, I just see a bare browser window with just the little bit of jQuery ouput from the exercise. I have noticed the same results in other training units. Is there some setup that I am missing? Thanks.
- PR Canegaly
- April 06, 2018
- Like
- 0
How to Write a Test Class for SOQL and Account Field Update?
Hello, I've just started to learn about Apex and I'm having difficulty writing a test class for the following Apex class.
The following Apex class has code to retrieve Account records and logic to iterate over a list of Account records and update the Description field. I learned this Apex class through Trailhead and wanted to write a test class for it but couldn't figure out how.
The following Apex class has code to retrieve Account records and logic to iterate over a list of Account records and update the Description field. I learned this Apex class through Trailhead and wanted to write a test class for it but couldn't figure out how.
public class OlderAccountsUtility { public static void updateOlderAccounts() { // Get the 5 oldest accounts Account[] oldAccounts = [SELECT Id, Description FROM Account ORDER BY CreatedDate ASC LIMIT 5]; // loop through them and update the Description field for (Account acct : oldAccounts) { acct.Description = 'Heritage Account'; } // save the change you made update oldAccounts; } }This is what I have written. Can anyone show me how to fix this Apex test unit so it works? Any help would be much appreciated!
@isTest private class OlderAccountsUtilityTest { @isTest static void createAccount() { List <Account> accts = new List<Account>(); for(integer i = 0; i<200; i++) { Account a = new Account(Name='testAccount'+'i'); accts.add(a); } insert accts; Test.StartTest(); List<Account> accList = [SELECT Id, Description FROM Account ORDER BY CreatedDate ASC LIMIT 5]; for (Account acct : accList) { acct.Description = 'Heritage Account'; } Test.StopTest(); System.AssertEquals( database.countquery('SELECT COUNT() FROM Account WHERE Description' = 'Heritage Account'), 5); } }
- Tina Chang 6
- March 13, 2018
- Like
- 0
Why mass uploads cancel out scores calculated by Apex code?
Hello, all,
We recently implemented this Apex code "Account Grading Method" (attached below) in our system. When an account record is created or edited, a process in the Process Builder calls the Apex code to calculate the account grade. It calculates the score of an account based on the input of a group of fields called Account Intelligence fields and returns the result to a number field called "Account Overall Grade".
The problem is whenever we mass update accounts using an upload (like API Data Loader) or via nightly API calls, the score of the Account Overall Grade get zeroed out, and they need to be re-triggered individually. The account fields we mass updated were not even related to the Account Intelligence fields. When we tried to mass update accounts in list view, the score zeroed out as well. For example, we took an account list view and selected a bunch of records to do a mass in-line editing of one of the Account Intelligence fields, the "Account Overall Grade" field zeroed out.
Any idea on how we can continue to be able to mass update accounts without this happening, or what the issue is? The person who developed this account grading process and the code had left. Any help would be much much appreciated!
My apologies this is a lot of code. Thank you for your patience.
Account Grading Method
We recently implemented this Apex code "Account Grading Method" (attached below) in our system. When an account record is created or edited, a process in the Process Builder calls the Apex code to calculate the account grade. It calculates the score of an account based on the input of a group of fields called Account Intelligence fields and returns the result to a number field called "Account Overall Grade".
The problem is whenever we mass update accounts using an upload (like API Data Loader) or via nightly API calls, the score of the Account Overall Grade get zeroed out, and they need to be re-triggered individually. The account fields we mass updated were not even related to the Account Intelligence fields. When we tried to mass update accounts in list view, the score zeroed out as well. For example, we took an account list view and selected a bunch of records to do a mass in-line editing of one of the Account Intelligence fields, the "Account Overall Grade" field zeroed out.
Any idea on how we can continue to be able to mass update accounts without this happening, or what the issue is? The person who developed this account grading process and the code had left. Any help would be much much appreciated!
My apologies this is a lot of code. Thank you for your patience.
Account Grading Method
public with sharing class AccountGradingMethod { public static void AccountGrading(Set<String> setAcctGradingIds) { List<Account> lstAGS = new List<Account>(); List<Account> lstUpdateAcct = new List<Account>(); Map<String, Field_Value__mdt> mapFlds = new Map<String, Field_Value__mdt>(); String strSOQLAcct; String strSOQLAcctGrade; Set<String> setFlds = new Set<String>(); Set<String> setFldName = new Set<String>(); String strScore; Decimal maxPossScore = 0; Decimal numberFlds = 0; strSOQLAcctGrade = 'SELECT Id'; for(Field_Value__mdt fn:[SELECT Id, Field_Score_or_Multiplier__c, Picklist_API_Value__c, Use_Greater_then_or_Less_then__c, Field_Name__r.Field_API_Name__c, Field_Name__r.Object__c, Field_Name__r.Type_of_Field__c, Greater_then__c, Less_then__c FROM Field_Value__mdt]){ String mapKey; setFldName.add(fn.Field_Name__r.Field_API_Name__c); if(fn.Field_Name__r.Object__c == 'Account'){ setFlds.add(fn.Field_Name__r.Field_API_Name__c); } if(fn.Use_Greater_then_or_Less_then__c){ mapKey = fn.Field_Name__r.Field_API_Name__c + '-' + fn.Greater_then__c + '-' + fn.Less_then__c; } else{ mapKey = fn.Field_Name__r.Field_API_Name__c + '-' + fn.Picklist_API_Value__c.deleteWhitespace(); } mapFlds.put(mapKey, fn); } for(String f:setFlds){ strSOQLAcctGrade += ', ' + f; } numberFlds = setFlds.size(); strSOQLAcctGrade += ' FROM Account WHERE Id in :setAcctGradingIds'; System.debug('strSOQLAcctGrade: ' + strSOQLAcctGrade); lstAGS = Database.query(strSOQLAcctGrade); strScore = System.Label.Max_Possible_Score; maxPossScore = Decimal.valueOf(strScore); for(Account ags:lstAGS){ Decimal subTotal = 0; Decimal multiplier = 1; Decimal decTotal = 0; Decimal ecommTotal = 0; Decimal ecommMin = 0; Decimal ecommMax = 0; Decimal fldsPopulated = 0; Decimal precentComplete = 0; for(Field_Value__mdt fldVal:mapFlds.values()){ String value; Decimal score = 0; Boolean skip = false; String fld = fldVal.Field_Name__r.Field_API_Name__c; String strKey; Decimal decValue = 0; if(setFldName.contains(fld)){ if(fldVal.Field_Name__r.Object__c == 'Account'){ value = String.valueOf(ags.get(fld)); if(value != '' && value != 'null' && value != null){ value = value.deleteWhitespace(); if(fldVal.Use_Greater_then_or_Less_then__c){ decValue = Decimal.valueOf(value); System.debug('decValue: ' + decValue); if(fldVal.Greater_then__c != null && fldVal.Less_then__c != null){ if(fldVal.Greater_then__c > decValue && fldVal.Less_then__c < decValue){ System.debug('if 1 fldVal.Less_then__c: ' + fldVal.Less_then__c); strKey = fld + '-' + fldVal.Greater_then__c + '-' + fldVal.Less_then__c; } else{ skip = true; } } if(fldVal.Greater_then__c == null && fldVal.Less_then__c != null){ if(fldVal.Less_then__c > decValue){ System.debug('if 2 fldVal.Less_then__c: ' + fldVal.Less_then__c); strKey = fld + '-' + fldVal.Greater_then__c + '-' + fldVal.Less_then__c; } else{ skip = true; } } if(fldVal.Greater_then__c != null && fldVal.Less_then__c == null){ System.debug('if 3 fldVal.Greater_then__c : ' + fldVal.Greater_then__c ); if(fldVal.Greater_then__c < decValue){ strKey = fld + '-' + fldVal.Greater_then__c + '-' + fldVal.Less_then__c; } else{ skip = true; } } } else if (!fldVal.Use_Greater_then_or_Less_then__c){ strKey = fldVal.Field_Name__r.Field_API_Name__c + '-' + value; } if(!String.isBlank(strKey) && mapFlds.get(strKey) != null){ setFldName.remove(fld); score = mapFlds.get(strKey).Field_Score_or_Multiplier__c; } if(score != null){ if(fld.startsWithIgnoreCase('e-commerce solutions')){ skip = true; ecommTotal = ecommTotal + score; if(ecommMax<score){ ecommMax = score; } if(ecommMin>score){ ecommMin = score; } } if(fldVal.Field_Name__r.Type_of_Field__c == 'Score' && !skip){ System.debug('fld: ' + fld); System.debug('Score: ' + score); subTotal = subTotal + score; } if(fldVal.Field_Name__r.Type_of_Field__c == 'Multiplier' && !skip){ System.debug('fld: ' + fld); System.debug('multiplier: ' + score); multiplier = multiplier * score; } } } else{ fldsPopulated = fldsPopulated + 1; setFldName.remove(fld); } } } } if(ecommTotal>=0){ subTotal = subTotal + ecommMax; } else{ subTotal = subTotal + ecommMin; } if(fldsPopulated == numberFlds){ precentComplete = 100; } else{ precentComplete = ((numberFlds - fldsPopulated)/numberFlds)*100; } System.debug('subtotal: ' + subTotal); subTotal = (subTotal/maxPossScore)*100; System.debug('subtotal after: ' + subTotal); System.debug('multiplier: ' + multiplier); decTotal = subTotal*multiplier; System.debug('decTotal: ' + decTotal ); Account a = new Account(); a.Id = ags.Id; a.Account_Overall_Grade__c = decTotal; a.Percent_Complete__c = precentComplete; lstUpdateAcct.add(a); } if(lstUpdateAcct.size()>0){ update lstUpdateAcct; } } }
- Tina Chang 6
- January 12, 2018
- Like
- 0
How to Fix this Apex Trigger? "The flow tried to update these records: null. This error occurred: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY: AccountTrigger"
Hello, there!
We have these two processes on Accounts in Process Builder -- one that updates a custom field called "Territory" based on the Billing State Code or the Billing City and/or the Region fields. The other that updates a custom field called "Region" based on the Billing Country field. Both processes have recently been getting the same error notifications as follows:
"The flow tried to update these records: null. This error occurred: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY: AccountTrigger: System.LimitException: Apex CPU time limit exceeded. For details, see API Exceptions."
Looks like it is being caused via the Process Builder but I suspect the root cause is the Apex here. Can you please look into this and see if the code needs to be made more efficient or fixed? I've attached the complete Apex trigger code and its test class below for your reference.
Please let me know if you require any further information. Your help will be greatly appreciated!
(My apologies this is a lof of code...)
AccountTrigger
AccountTriggerHandler
AccountTriggerHandlerTest
We have these two processes on Accounts in Process Builder -- one that updates a custom field called "Territory" based on the Billing State Code or the Billing City and/or the Region fields. The other that updates a custom field called "Region" based on the Billing Country field. Both processes have recently been getting the same error notifications as follows:
"The flow tried to update these records: null. This error occurred: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY: AccountTrigger: System.LimitException: Apex CPU time limit exceeded. For details, see API Exceptions."
Looks like it is being caused via the Process Builder but I suspect the root cause is the Apex here. Can you please look into this and see if the code needs to be made more efficient or fixed? I've attached the complete Apex trigger code and its test class below for your reference.
Please let me know if you require any further information. Your help will be greatly appreciated!
(My apologies this is a lof of code...)
AccountTrigger
/******************************************************************************* * AccountTrigger * @Description: Master Trigger for Accounts, which fires on ALL events to * control the order in which trigger actions occur. Follows the * super trigger best practice from Salesforce: * * https://developer.salesforce.com/page/Trigger_Frameworks_and_Apex_Trigger_Best_Practices * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ trigger AccountTrigger on Account (before insert, before update) { // Determine what action is // occurring and when, and // pass the correct context // variables to the Trigger // Handler class where all the // actions will take place if (Trigger.isBefore && Trigger.isInsert) { // Before Insert AccountTriggerHandler.beforeInsert(Trigger.new, Trigger.newMap); } else if (Trigger.isBefore && Trigger.isUpdate) { // Before Update AccountTriggerHandler.beforeUpdate(Trigger.new, Trigger.newMap, Trigger.old, Trigger.oldMap); } /* FOR FRAMEWORK SETUP ONLY. NO ACTIONS ARE TAKEN AT THIS TIME else if (Trigger.isBefore && Trigger.isDelete) { // Before Delete AccountTriggerHandler.beforeDelete(Trigger.new, Trigger.newMap, Trigger.old, Trigger.oldMap); } else if (Trigger.isAfter && Trigger.isInsert) { // After Insert AccountTriggerHandler.afterInsert(Trigger.new, Trigger.newMap); } else if (Trigger.isAfter && Trigger.isUpdate) { // After Update AccountTriggerHandler.afterUpdate(Trigger.new, Trigger.newMap, Trigger.old, Trigger.oldMap); } else if (Trigger.isAfter && Trigger.isDelete) { // After Delete AccountTriggerHandler.afterDelete(Trigger.new, Trigger.old); } else if (Trigger.isAfter && Trigger.isUnDelete) { // After UnDelete AccountTriggerHandler.afterUnDelete(Trigger.new, Trigger.old); } */ }
AccountTriggerHandler
/******************************************************************************* * AccountTriggerHandler * @Description: Class for handling all Account Trigger functionality. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ public with sharing class AccountTriggerHandler { /******************************************************************************* * beforeInsert * @Description: Method for handling all "BeforeInsert" functionality. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ public static void beforeInsert(List<Account> a_newRecordList, Map<Id,Account> a_newRecordMap) { System.debug('*** START: AccountTriggerHandler.beforeInsert()'); // Iterate through the new Account // records and grab their SIC Code // (if applicable). Set<String> sicCodeSet = new Set<String>(); for (Account a : a_newRecordList) { if (a.Sic != null && a.Sic != '') { sicCodeSet.add(a.Sic); } } // Do we have a Set of SIC Codes? if (!sicCodeSet.isEmpty()) { // Re-Iterate through the new Account // records and set their reference to // a SIC_Code__c record (if applicable). Map<String,Id> sicCodeIdMap = getSICCodeMap(sicCodeSet); for (Account a : a_newRecordList) { if (sicCodeIdMap.containsKey(a.Sic)) { a.SIC_Code__c = sicCodeIdMap.get(a.Sic); } } } System.debug('*** END: AccountTriggerHandler.beforeInsert()'); } // End Method: beforeInsert() /******************************************************************************* * beforeUpdate * @Description: Method for handling all "Before Update" functionality. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ public static void beforeUpdate(List<Account> a_newRecordList, Map<Id,Account> a_newRecordMap, List<Account> a_oldRecordList, Map<Id,Account> a_oldRecordMap) { System.debug('*** START: AccountTriggerHandler.beforeUpdate()'); // Iterate through the updated Account // records and grab their SIC Codes // (if applicable). Set<String> sicCodeSet = new Set<String>(); for (Account a : a_newRecordList) { // Was the SIC Code changed? if (a.Sic != a_oldRecordMap.get(a.Id).Sic) { // If the SIC Code was removed, clear // the Lookup field. if (a.Sic == null || a.Sic == '') { a.SIC_Code__c = null; } else { sicCodeSet.add(a.Sic); } } } // Do we have a Set of SIC Codes? if (!sicCodeSet.isEmpty()) { // Re-Iterate through the updated Account // records and set their reference to // a SIC_Code__c record (if applicable). Map<String,Id> sicCodeIdMap = getSICCodeMap(sicCodeSet); for (Account a : a_newRecordList) { if (sicCodeIdMap.containsKey(a.Sic)) { a.SIC_Code__c = sicCodeIdMap.get(a.Sic); } } } System.debug('*** END: AccountTriggerHandler.beforeUpdate()'); } // End Method: beforeUpdate() /******************************************************************************* * getSICCodeMap * @Description: Method for returning a Map of SIC Code-to-SIC Code Id. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Paramaters: Set<String> a_sicCodeSet * @Return: Map<String,Id> returnMap * * @Updates: N/A *******************************************************************************/ private static Map<String, Id> getSICCodeMap(Set<String> a_sicCodeSet) { System.debug('*** START: AccountTriggerHandler.getSICCodeMap()'); Map<String,Id> returnMap = new Map<String,Id>(); // Iterate through the SIC Code records // associated with the SIC Codes on the // Account records that have been // modified. for (SIC_Code__c sc : [SELECT Id, Name FROM SIC_Code__c WHERE Name IN :a_sicCodeSet]) { // This SIC Code is associated with an // Account that has been updated, so // add it to the Map being returned. returnMap.put(sc.Name, sc.Id); } System.debug('*** returnMap: ' + returnMap); System.debug('*** END: AccountTriggerHandler.getSICCodeMap()'); return returnMap; } // End Method: getSICCodeMap() }
AccountTriggerHandlerTest
/******************************************************************************* * AccountTriggerHandlerTest * @Description: Test class for AccountTriggerHandler class. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ @isTest public class AccountTriggerHandlerTest { private static final String sicCode_Name = '01110000'; private static final String sicCode_Description = 'WHEAT'; /******************************************************************************* * TestDataSetup * @Description: Method to create test data to be used throughout the class. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ @testSetup static void TestDataSetup() { System.debug('*** START: AccountTriggerHandlerTest.test_beforeInsert()'); Id sysAdminProfileId = [SELECT Id FROM Profile WHERE Name = 'System Administrator'].Id; Id execTeamRoleId = [SELECT Id FROM UserRole WHERE DeveloperName = 'ExecutiveTeam'].Id; // Create System Administrator User. User adminUser = new User(); adminUser.LastName = 'dr' + Crypto.getRandomInteger(); adminUser.Email = adminUser.LastName + '@drtest.com'; adminUser.UserName = adminUser.Email; adminUser.Alias = String.valueOf(adminUser.LastName.SubString(0,8)); adminUser.ProfileId = sysAdminProfileId; adminUser.UserRoleId = execTeamRoleId; adminUser.LocaleSidKey = 'en_US'; adminUser.LanguageLocaleKey = 'en_US'; adminUser.TimeZoneSidKey = 'America/Los_Angeles'; adminUser.EmailEncodingKey = 'UTF-8'; insert(adminUser); system.runAs(adminUser) { SIC_Code__c sicCode = new SIC_Code__c(); sicCode.Name = sicCode_Name; sicCode.Description__c = sicCode_Description; insert(sicCode); } } /******************************************************************************* * test_beforeInsert * @Description: Method to test the "beforeInsert" method. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ public static testMethod void test_beforeInsert() { System.debug('*** START: AccountTriggerHandlerTest.test_beforeInsert()'); Id sysAdminProfileId = [SELECT Id FROM Profile WHERE Name = 'System Administrator'].Id; Id execTeamRoleId = [SELECT Id FROM UserRole WHERE DeveloperName = 'ExecutiveTeam'].Id; // Create System Administrator User. User adminUser = new User(); adminUser.LastName = 'dr' + Crypto.getRandomInteger(); adminUser.Email = adminUser.LastName + '@drtest.com'; adminUser.UserName = adminUser.Email; adminUser.Alias = String.valueOf(adminUser.LastName.SubString(0,8)); adminUser.ProfileId = sysAdminProfileId; adminUser.UserRoleId = execTeamRoleId; adminUser.LocaleSidKey = 'en_US'; adminUser.LanguageLocaleKey = 'en_US'; adminUser.TimeZoneSidKey = 'America/Los_Angeles'; adminUser.EmailEncodingKey = 'UTF-8'; insert(adminUser); system.runAs(adminUser) { Test.startTest(); Account newAccount = new Account(); newAccount.Name = 'Test Account'; newAccount.CurrencyIsoCode = 'USD'; newAccount.Website = 'http://www.somewhere.com/'; newAccount.Vertical_Market__c = 'Marketplace'; newAccount.E_Commerce_Solution_US__c = 'Amazon'; newAccount.AlexaRating__c = '0-10K'; newAccount.Sic = sicCode_Name; insert(newAccount); Test.stopTest(); List<Account> newAccountList = [SELECT Id, SICDescription__c FROM Account WHERE Id = :newAccount.Id AND SICDescription__c = :sicCode_Description]; //System.assert(newAccountList.size() > 0, 'Account was NOT created with SIC Code reference'); } System.debug('*** END: AccountTriggerHandlerTest.test_beforeInsert()'); } // End Method: test_afterInsert() /******************************************************************************* * test_beforeUpdate * @Description: Method to test the "beforeUpdate" method. * * @Date: 12/15/2015 * @Author: Jason Flippen (Demand Chain Systems) * * @Updates: N/A *******************************************************************************/ public static testMethod void test_beforeUpdate() { System.debug('*** START: AccountTriggerHandlerTest.test_beforeUpdate()'); Id sysAdminProfileId = [SELECT Id FROM Profile WHERE Name = 'System Administrator'].Id; Id execTeamRoleId = [SELECT Id FROM UserRole WHERE DeveloperName = 'ExecutiveTeam'].Id; // Create System Administrator User. User adminUser = new User(); adminUser.LastName = 'dr' + Crypto.getRandomInteger(); adminUser.Email = adminUser.LastName + '@drtest.com'; adminUser.UserName = adminUser.Email; adminUser.Alias = String.valueOf(adminUser.LastName.SubString(0,8)); adminUser.ProfileId = sysAdminProfileId; adminUser.UserRoleId = execTeamRoleId; adminUser.LocaleSidKey = 'en_US'; adminUser.LanguageLocaleKey = 'en_US'; adminUser.TimeZoneSidKey = 'America/Los_Angeles'; adminUser.EmailEncodingKey = 'UTF-8'; insert(adminUser); system.runAs(adminUser) { Test.startTest(); Account newAccount = new Account(); newAccount.Name = 'Test Account'; newAccount.CurrencyIsoCode = 'USD'; newAccount.Website = 'http://www.somewhere.com/'; newAccount.Vertical_Market__c = 'Marketplace'; newAccount.E_Commerce_Solution_US__c = 'Amazon'; newAccount.AlexaRating__c = '0-10K'; insert(newAccount); List<Account> newAccountList = [SELECT Id, SICDescription__c FROM Account WHERE Id = :newAccount.Id AND SICDescription__c = :sicCode_Description]; System.assert(newAccountList.size() == 0, 'Account was created with SIC Code Reference'); newAccount.Sic = sicCode_Name; update(newAccount); List<Account> updatedAccountList = [SELECT Id, SICDescription__c FROM Account WHERE Id = :newAccount.Id AND SICDescription__c = :sicCode_Description]; //System.assert(updatedAccountList.size() > 0, 'Account was NOT updated with SIC Code Reference'); Test.stopTest(); } System.debug('*** END: AccountTriggerHandlerTest.test_beforeUpdate()'); } // End Method: test_beforeUpdate() }
- Tina Chang 6
- January 04, 2018
- Like
- 0
Apex "Else If" statement won't hit. Please help! Thank you!
Hello, there! The highlighted "if" and "else if" statement for the "Percent Complete" field below never execute. Only the "else" statement would work as expected. Does anyone know how to re-write to make it work? Any help will be greatly appreciated!!
Here's the complete Apex Class. Everything else works except for the if statement for the Percent Complete field mentioned above. Thanks in advance for your suggestions!
if(fldsPopulated == numberFlds){ PrecentComplete = 100; }else if(fldsPopulated == 0){ PrecentComplete = 0; }else{ precentComplete = ((numberFlds - fldsPopulated)/numberFlds)*100; }
Here's the complete Apex Class. Everything else works except for the if statement for the Percent Complete field mentioned above. Thanks in advance for your suggestions!
public with sharing class AccountGradingMethod { public static void AccountGrading(Set<String> setAcctGradingIds) { List<Account> lstAGS = new List<Account>(); List<Account> lstUpdateAcct = new List<Account>(); Map<String, Field_Value__mdt> mapFlds = new Map<String, Field_Value__mdt>(); String strSOQLAcct; String strSOQLAcctGrade; Set<String> setFlds = new Set<String>(); Set<String> setFldName = new Set<String>(); String strScore; Decimal maxPossScore = 0; Decimal numberFlds = 0; strSOQLAcctGrade = 'SELECT Id'; for(Field_Value__mdt fn:[SELECT Id, Field_Score_or_Multiplier__c, Picklist_API_Value__c, Use_Greater_then_or_Less_then__c, Field_Name__r.Field_API_Name__c, Field_Name__r.Object__c, Field_Name__r.Type_of_Field__c, Greater_then__c, Less_then__c FROM Field_Value__mdt]){ String mapKey; setFldName.add(fn.Field_Name__r.Field_API_Name__c); if(fn.Field_Name__r.Object__c == 'Account'){ setFlds.add(fn.Field_Name__r.Field_API_Name__c); } if(fn.Use_Greater_then_or_Less_then__c){ mapKey = fn.Field_Name__r.Field_API_Name__c + '-' + fn.Greater_then__c + '-' + fn.Less_then__c; } else{ mapKey = fn.Field_Name__r.Field_API_Name__c + '-' + fn.Picklist_API_Value__c.deleteWhitespace(); } mapFlds.put(mapKey, fn); } for(String f:setFlds){ strSOQLAcctGrade += ', ' + f; } numberFlds = setFlds.size(); strSOQLAcctGrade += ' FROM Account WHERE Id in :setAcctGradingIds'; System.debug('strSOQLAcctGrade: ' + strSOQLAcctGrade); lstAGS = Database.query(strSOQLAcctGrade); strScore = System.Label.Max_Possible_Score; maxPossScore = Decimal.valueOf(strScore); for(Account ags:lstAGS){ Decimal subTotal = 0; Decimal multiplier = 1; Decimal decTotal = 0; Decimal ecommTotal = 0; Decimal ecommMin = 0; Decimal ecommMax = 0; Decimal fldsPopulated = 0; Decimal precentComplete = 0; for(Field_Value__mdt fldVal:mapFlds.values()){ String value; Decimal score = 0; Boolean skip = false; String fld = fldVal.Field_Name__r.Field_API_Name__c; String strKey; Decimal decValue = 0; if(setFldName.contains(fld)){ if(fldVal.Field_Name__r.Object__c == 'Account'){ value = String.valueOf(ags.get(fld)); if(value != '' && value != 'null' && value != null){ value = value.deleteWhitespace(); if(fldVal.Use_Greater_then_or_Less_then__c){ decValue = Decimal.valueOf(value); System.debug('decValue: ' + decValue); if(fldVal.Greater_then__c != null && fldVal.Less_then__c != null){ if(fldVal.Greater_then__c > decValue && fldVal.Less_then__c < decValue){ System.debug('if 1 fldVal.Less_then__c: ' + fldVal.Less_then__c); strKey = fld + '-' + fldVal.Greater_then__c + '-' + fldVal.Less_then__c; } else{ skip = true; } } if(fldVal.Greater_then__c == null && fldVal.Less_then__c != null){ if(fldVal.Less_then__c > decValue){ System.debug('if 2 fldVal.Less_then__c: ' + fldVal.Less_then__c); strKey = fld + '-' + fldVal.Greater_then__c + '-' + fldVal.Less_then__c; } else{ skip = true; } } if(fldVal.Greater_then__c != null && fldVal.Less_then__c == null){ System.debug('if 3 fldVal.Greater_then__c : ' + fldVal.Greater_then__c ); if(fldVal.Greater_then__c < decValue){ strKey = fld + '-' + fldVal.Greater_then__c + '-' + fldVal.Less_then__c; } else{ skip = true; } } } else if (!fldVal.Use_Greater_then_or_Less_then__c){ strKey = fldVal.Field_Name__r.Field_API_Name__c + '-' + value; } if(!String.isBlank(strKey) && mapFlds.get(strKey) != null){ setFldName.remove(fld); score = mapFlds.get(strKey).Field_Score_or_Multiplier__c; } if(score != null){ if(fld.startsWithIgnoreCase('e-commerce solutions')){ skip = true; ecommTotal = ecommTotal + score; if(ecommMax<score){ ecommMax = score; } if(ecommMin>score){ ecommMin = score; } } if(fldVal.Field_Name__r.Type_of_Field__c == 'Score' && !skip){ System.debug('fld: ' + fld); System.debug('Score: ' + score); subTotal = subTotal + score; } if(fldVal.Field_Name__r.Type_of_Field__c == 'Multiplier' && !skip){ System.debug('fld: ' + fld); System.debug('multiplier: ' + score); multiplier = multiplier * score; } } } else{ fldsPopulated = fldsPopulated + 1; setFldName.remove(fld); } } } } if(ecommTotal>=0){ subTotal = subTotal + ecommMax; } else{ subTotal = subTotal + ecommMin; } if(fldsPopulated == numberFlds){ PrecentComplete = 100; }else if(fldsPopulated == 0){ PrecentComplete = 0; }else{ precentComplete = ((numberFlds - fldsPopulated)/numberFlds)*100; } System.debug('subtotal: ' + subTotal); subTotal = (subTotal/maxPossScore)*100; System.debug('subtotal after: ' + subTotal); System.debug('multiplier: ' + multiplier); decTotal = subTotal*multiplier; System.debug('decTotal: ' + decTotal ); Account a = new Account(); a.Id = ags.Id; a.Account_Overall_Grade__c = decTotal; a.Percent_Complete__c = precentComplete; a.LastScoredDate__c = System.today(); lstUpdateAcct.add(a); } if(lstUpdateAcct.size()>0){ update lstUpdateAcct; } } }
- Tina Chang 6
- December 11, 2017
- Like
- 0
How to Fix this Apex Test Class?
Hello! I have to deactivate a Trigger as it is blocking a deployment.
I deactivated the Trigger in the sandbox, created a Change Set, included the Trigger in the change set and deployed the same into the Production, but it wasn't able to pass the Apex Test.
This is the error message that I got:
I know the problem is with this code "System.assert(false);" but I am not a developer so I don't know how to fix it. I have searched around for solutions but it is way beyond my knowledge.
Can anyone kindly help and let me know how to fix this Apex Test Class? It would be much much appreciated!
The Apex Trigger
The Apex Test Class
Tina
Sincerely,
Tina
I deactivated the Trigger in the sandbox, created a Change Set, included the Trigger in the change set and deployed the same into the Production, but it wasn't able to pass the Apex Test.
This is the error message that I got:
- System.Exception: Assertion Failed
- Stack Trace: Class.test_updatecontactrolecount.testcreateopptywithconditionandrole: line 38, column 1
I know the problem is with this code "System.assert(false);" but I am not a developer so I don't know how to fix it. I have searched around for solutions but it is way beyond my knowledge.
Can anyone kindly help and let me know how to fix this Apex Test Class? It would be much much appreciated!
The Apex Trigger
trigger updatecontactrolecount on Opportunity (before insert, before update) { Boolean isPrimary; Integer iCount; Map<String, Opportunity> oppty_con = new Map<String, Opportunity>();//check if the contact role is needed and add it to the oppty_con map for (Integer i = 0; i < Trigger.new.size(); i++) { oppty_con.put(Trigger.new[i].id, Trigger.new[i]); } isPrimary = False; for (List<OpportunityContactRole> oppcntctrle :[select OpportunityId from OpportunityContactRole where (OpportunityContactRole.IsPrimary = True and OpportunityContactRole.OpportunityId in :oppty_con.keySet())]) { if (oppcntctrle .Size() >0) { isPrimary = True; } } iCount = 0; for (List<OpportunityContactRole> oppcntctrle2 : [select OpportunityId from OpportunityContactRole where (OpportunityContactRole.OpportunityId in :oppty_con.keySet())])//Query for Contact Roles { if (oppcntctrle2 .Size()>0) { iCount= oppcntctrle2 .Size(); } } for (Opportunity Oppty : system.trigger.new) //Check if roles exist in the map or contact role isn't required { Oppty.Number_of_Contacts_Roles_Assigned__c = iCount; Oppty.Primary_Contact_Assigned__c =isPrimary; } }
The Apex Test Class
public class test_updatecontactrolecount { public static testMethod void testcreateopptywithconditionandrole() { //Insert Opportunities try { Opportunity Oppty = new Opportunity(Name='Oppty_test1',StageName='Stage 1 - Unqualified Prospect',Type ='New Business', CloseDate=System.Today()); insert Oppty; // insert contact Contact[] cont = new Contact[] { new Contact(LastName = 'testcontact1'), new Contact(LastName = 'testcontact2') }; insert cont; // insert contact role OpportunityContactRole [] ocr = new OpportunityContactRole[] { new OpportunityContactRole(Role ='Business User',OpportunityId=Oppty.id ,Contactid = cont[0].id ,Isprimary = True), new OpportunityContactRole(Role ='Business User',OpportunityId=Oppty.id ,Contactid = cont[0].id ,Isprimary = False) }; insert ocr; Oppty.StageName = 'Stage 3 - Eval Request'; //Update opportunity Test.StartTest(); update Oppty; Test.StopTest(); Oppty =[SELECT Number_of_Contacts_Roles_Assigned__c,Primary_Contact_Assigned__c FROM Opportunity WHERE Id = :Oppty.Id]; system.assert (Oppty.Number_of_Contacts_Roles_Assigned__c == 2); system.assert (Oppty.Primary_Contact_Assigned__c == True); } catch (System.DmlException e) { System.assert(false); } } }Sincerely,
Tina
Sincerely,
Tina
- Tina Chang 6
- September 08, 2017
- Like
- 0
After embedding a Flow in Visualforce Page, how to make a Visualforce Page form display only for certain screen of Flow?
Hello, everyone! I had a flow embedded in a Visualforce Page. And then I created a apex form for users to upload the files. My question is: is it possible to display this Upload an Attachment form area for only the 1st Intro Screen in the flow session? That is, I don't want the users to see the Upload an Attachment section during the flow session except for the 1st Intro Screen - STEP 1 as captured in the image below. Is there a way for me to hide that section for the rest of the steps? Any suggestions and help would be greatly appreciated!! :)
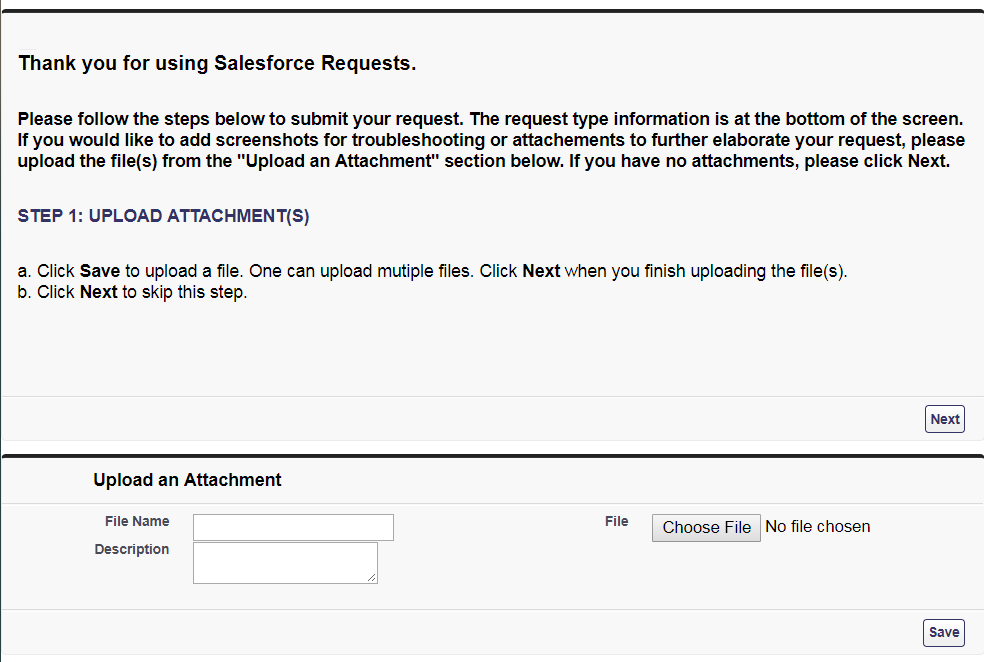
The Visualforce Page:
Following is the Apex Class: AttachmentUploadController
The Visualforce Page:
<apex:page sidebar="false" showHeader="false" title="Salesforce Requests" controller="AttachmentUploadController"> <flow:interview name="Salesforce_Request_System" finishLocation="{!URLFOR('/home/home.jsp')}" buttonLocation="bottom" buttonStyle="color:#333366; background-color:#fed; border:1px solid;"/> <apex:form enctype="multipart/form-data"> <apex:pageMessages /> <apex:pageBlock title="Upload an Attachment" > <apex:pageBlockButtons location="bottom"> <apex:commandButton action="{!upload}" value="Save" style="color:#333366; background-color:#fed; border:1px solid;"/> </apex:pageBlockButtons> <apex:pageBlockSection showHeader="false" columns="2" id="block1"> <apex:pageBlockSectionItem > <apex:outputLabel value="File Name" for="fileName"/> <apex:inputText value="{!attachment.name}" id="fileName"/> </apex:pageBlockSectionItem> <apex:pageBlockSectionItem > <apex:outputLabel value="File" for="file"/> <apex:inputFile value="{!attachment.body}" filename="{!attachment.name}" id="file"/> </apex:pageBlockSectionItem> <apex:pageBlockSectionItem > <apex:outputLabel value="Description" for="description"/> <apex:inputTextarea value="{!attachment.description}" id="description"/> </apex:pageBlockSectionItem> </apex:pageBlockSection> </apex:pageBlock> </apex:form> </apex:page>
Following is the Apex Class: AttachmentUploadController
public with sharing class AttachmentUploadController { public Attachment attachment { get { if (attachment == null) attachment = new Attachment(); return attachment; } set; } public PageReference upload() { attachment.OwnerId = UserInfo.getUserId(); attachment.ParentId = '500S0000008lP0o'; attachment.IsPrivate = true; try { insert attachment; } catch (DMLException e) { ApexPages.addMessage(new ApexPages.message(ApexPages.severity.ERROR,'Error uploading attachment')); return null; } finally { attachment = new Attachment(); } ApexPages.addMessage(new ApexPages.message(ApexPages.severity.INFO,'Attachment uploaded successfully')); return null; } }
- Tina Chang 6
- July 20, 2017
- Like
- 0
Trailhead: Coverage 100% but challenge failed
I have this very simple class..
When I run the Test class from the Developer Console I get 100% of coverage on the RestrictContactByName class but, when I check the challenge on the trailhead it returns the error:
Challenge not yet complete... here's what's wrong: The 'RestrictContactByName' class did not achieve 100% code coverage via your test methods
Has someone had my same issue?
trigger RestrictContactByName on Contact (before insert, before update) { //check contacts prior to insert or update for invalid data For (Contact c : Trigger.New) { if(c.LastName == 'INVALIDNAME') { //invalidname is invalid c.AddError('The Last Name "'+c.LastName+'" is not allowed for DML'); } } }.. and the corresponding Test Class:
@isTest private class TestRestrictContactByName { @isTest static void metodoTest() { List listaContatti = new List(); Contact c1 = new Contact(FirstName='Francesco', LastName='Riggio'); Contact c2 = new Contact(LastName = 'INVALIDNAME'); listaContatti.add(c1); listaContatti.add(c2); //insert listaContatti; // Perform test Test.startTest(); Database.SaveResult [] result = Database.insert(listaContatti, false); Test.stopTest(); c1.LastName = 'INVALIDNAME'; update c1; } }
When I run the Test class from the Developer Console I get 100% of coverage on the RestrictContactByName class but, when I check the challenge on the trailhead it returns the error:
Challenge not yet complete... here's what's wrong: The 'RestrictContactByName' class did not achieve 100% code coverage via your test methods
Has someone had my same issue?
- Andrea Ianni
- June 24, 2015
- Like
- 0