You need to sign in to do that
Don't have an account?

Trigger Handler for the following:
Hi there, I am relatively new to apex coding and need to write a trigger handler for the following Trigger. I understand the general logic and reason for why I need to do it, but unsure on how exacty it needs to be done. i.e. do I leave my definition of the ID sets in the trigger and move everything else to the class? Do I need to pass anything from the trigger to the class when I call it (specially if the id sets are defined/declared in the trigger)? Your help is greatly appreciated!
trigger AgreementHasAttachment on ContentDocumentLink (after insert) {
Set<Id> setParentId = new Set<Id>();
Set<Id> setGrandParentId = new Set<Id>();
for (ContentDocumentLink cdl : trigger.new) {
setParentId.add(cdl.LinkedEntityId);
}
List<Agreement__c> Agreementlst = new List<Agreement__c>();
Agreementlst = [select Id, HasAttachment__c, MDU_Referral_Contact__c from Agreement__c where Id IN :setParentId];
For (Agreement__c a : Agreementlst)
{
a.HasAttachment__c = True;
setGrandParentId.add(a.MDU_Referral_Contact__c);
}
List<Contact> Contlst = new List<Contact>();
Contlst = [Select Id, Contract_Uploaded__c from Contact where Id IN :setGrandParentId];
For (Contact c : Contlst){
c.Contract_Uploaded__c = true;
}
if(Agreementlst.size() > 0) update Agreementlst;
if(Contlst.size() > 0) update Contlst;
}
trigger AgreementHasAttachment on ContentDocumentLink (after insert) {
Set<Id> setParentId = new Set<Id>();
Set<Id> setGrandParentId = new Set<Id>();
for (ContentDocumentLink cdl : trigger.new) {
setParentId.add(cdl.LinkedEntityId);
}
List<Agreement__c> Agreementlst = new List<Agreement__c>();
Agreementlst = [select Id, HasAttachment__c, MDU_Referral_Contact__c from Agreement__c where Id IN :setParentId];
For (Agreement__c a : Agreementlst)
{
a.HasAttachment__c = True;
setGrandParentId.add(a.MDU_Referral_Contact__c);
}
List<Contact> Contlst = new List<Contact>();
Contlst = [Select Id, Contract_Uploaded__c from Contact where Id IN :setGrandParentId];
For (Contact c : Contlst){
c.Contract_Uploaded__c = true;
}
if(Agreementlst.size() > 0) update Agreementlst;
if(Contlst.size() > 0) update Contlst;
}
If you want to move the entire trigger code in the handler class, you can do the following.
Step 1: write your trigger with below code.
trigger AgreementHasAttachment on ContentDocumentLink (after insert) {
new AgreementHasAttachmentHandler.run();
}
Step 2: create the class AgreementHasAttachmentHandler
public class AgreementHasAttachmentHandler extends TriggerHandler{
// variables
private LIst<ContentDocumentLink> triggerNew = (ContentDocumentLink[]) Trigger.new;
// constructor
public AgreementHasAttachmentHandler(){}
// override trigger methods
public override void afterInsert(){
for(ContentDocumentLink cdl : triggerNew){
}
}
}
Let me know if this is the soluition helps you.
Trigger.
Class
Please mark this as best answer if it resolves your query.
Thanks.
I noticed that the declaration of the id sets (setParentId and setGrandParentId) is not visible anywhere . Is this not required or did you miss it?
Hi Ankit, I am having trouble compiling the code. Please see below errors:
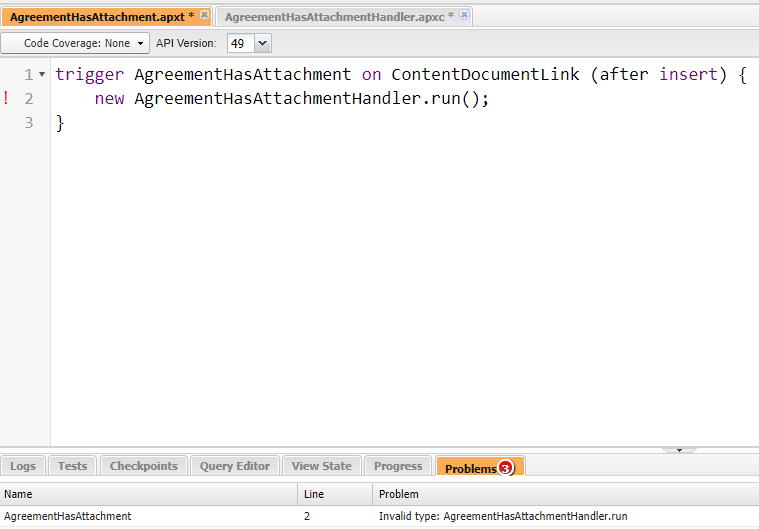
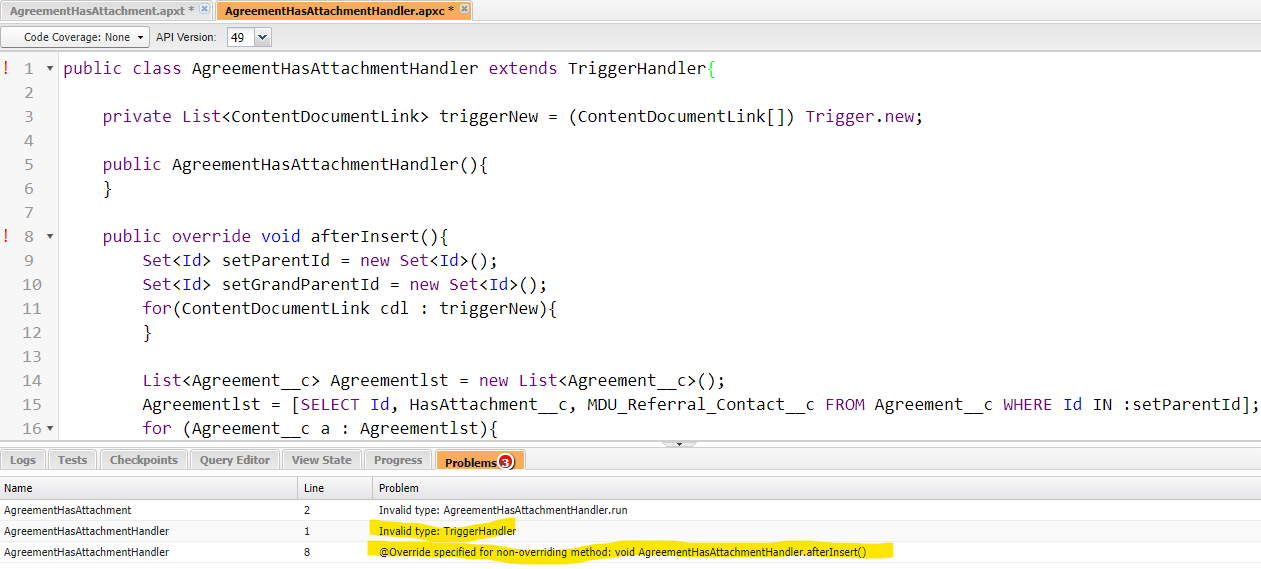
Trigger error:
Class error:
I have missed the parenthesis in the trigger code which I have provided.
Please update the trigger and it will work.
Sorry Ankit, but it is still not compiling
Error on Trigger: Method does not exist or incorrect signature: void run() from the type AgreementHasAttachmentHandler (line2)
trigger AgreementHasAttachment on ContentDocumentLink (after insert) {
new AgreementHasAttachmentHandler().run();
}
-----------------------
Error on Class: @Override specified for non-overriding method: void AgreementHasAttachmentHandler.afterInsert() (Line 8)
public class AgreementHasAttachmentHandler {
private List<ContentDocumentLink> triggerNew = (ContentDocumentLink[]) Trigger.new;
public AgreementHasAttachmentHandler(){
}
public override void afterInsert(){
for(ContentDocumentLink cdl : triggerNew){
}
List<Agreement__c> Agreementlst = new List<Agreement__c>();
Agreementlst = [SELECT Id, HasAttachment__c, MDU_Referral_Contact__c FROM Agreement__c WHERE Id IN :setParentId];
for (Agreement__c a : Agreementlst){
a.HasAttachment__c = true;
setGrandParentId.add(a.MDU_Referral_Contact__c);
}
List<Contact> Contlst = new List<Contact>();
Contlst = [SELECT Id, Contract_Uploaded__c FROM Contact WHERE Id IN :setGrandParentId];
for (Contact c : Contlst){
c.Contract_Uploaded__c = true;
}
if(Agreementlst.size() > 0) UPDATE Agreementlst;
if(Contlst.size() > 0) UPDATE Contlst;
}
}
I have commented code inside trigger since I do not have custom object "Agreement" in my org.
Can you add below class and compile your trigger ?