You need to sign in to do that
Don't have an account?

How to add Custom Objects to an App after creation of the objects?
Hi,
I am following the book "Developement with the Force.com platform" by JasonOuelette.
When creating the Custom Objects, I did not check the box to be visible in a tab, and so I could not add this object to the Custom App I was creating. Now I have a created fields and relationships in the custom object. How do I add this Object to the Custom App?
thanks
Svidya
Commercial Real Estate App
Hi out there,
I am new on this amazing platform and I want to build a Commercial Real Estate App on top force.com. I know there are a couple in the AppExchange but they are too extensive for my needs.
I know all the functionality I want to include.
My question is if I can use the Lightning to do this or if I need to write code?
Best Regards,
- Jörgen Ekelund
I am new on this amazing platform and I want to build a Commercial Real Estate App on top force.com. I know there are a couple in the AppExchange but they are too extensive for my needs.
I know all the functionality I want to include.
My question is if I can use the Lightning to do this or if I need to write code?
Best Regards,
- Jörgen Ekelund


Hello!
Welcome to the Salesforce Platform! The answer to your question is difficult because you do not give details as to the functionality you are looking for. There are many, many processes that can be developed for Salesforce using 'point and click' functionality (no code). These processes can be put together for a fully functioning app, on both the Salesforce Classic interface and the new Lightning Interface. It is recommended that you go through the Trailhead tutorials for much more information on how design and use those. Whether or not you have to write code is completely dependent on 'how' you want your App to work. Before determining whether you 'need to write code', create the Business documentation and Use Cases for your app (describing how its supposed to operate from a high-level). If you are not sure how to go about writing it, then might I suggest writing it in the format that Salesforce has used to provide 'test cases' that need to be solved using Salesforce. One such example can be found at the new 'Supebadge' trailheads. An example is here (https://developer.salesforce.com/trailhead/en/super_badges/superbadge_apex" target="_blank) (See the 'Use Case' section on that page). After you do that, you will have a much clearer picture of the different 'components' and 'features' your app will have, and then you can do your research into each of these to determine the level of effort and tools on the platform you will need to create such an app.
Hapy trails!
Welcome to the Salesforce Platform! The answer to your question is difficult because you do not give details as to the functionality you are looking for. There are many, many processes that can be developed for Salesforce using 'point and click' functionality (no code). These processes can be put together for a fully functioning app, on both the Salesforce Classic interface and the new Lightning Interface. It is recommended that you go through the Trailhead tutorials for much more information on how design and use those. Whether or not you have to write code is completely dependent on 'how' you want your App to work. Before determining whether you 'need to write code', create the Business documentation and Use Cases for your app (describing how its supposed to operate from a high-level). If you are not sure how to go about writing it, then might I suggest writing it in the format that Salesforce has used to provide 'test cases' that need to be solved using Salesforce. One such example can be found at the new 'Supebadge' trailheads. An example is here (https://developer.salesforce.com/trailhead/en/super_badges/superbadge_apex" target="_blank) (See the 'Use Case' section on that page). After you do that, you will have a much clearer picture of the different 'components' and 'features' your app will have, and then you can do your research into each of these to determine the level of effort and tools on the platform you will need to create such an app.
Hapy trails!

how to prevent record update or editing based on a condition
If picklist field status = VOID, then user cannot update or edit record.
I keep running into a solution where a validation rule or flow prevents user from updating picklist to void. This is not the requirement. The requirement is once the status is already changed to VOID and the record is saved, then no further updates or edits can be made.
I keep running into a solution where a validation rule or flow prevents user from updating picklist to void. This is not the requirement. The requirement is once the status is already changed to VOID and the record is saved, then no further updates or edits can be made.




Hi Ahon,
You can try below validation rule.
Important Update - last chance
Heads up — this is your last chance to get your Trailblazer account set up and connected to your forums discussions on this site.Please take these steps before November 30, 2023, 11:59 p.m. PT to ensure your contributions follow you to the Trailblazer Community:
We know that moving platforms can be a hassle, so we created a special forums users group, the Migration Support Hub, in the Trailblazer Community where you can find other forums users and get training videos, the latest information, and assistance.We want to thank you for all of your time, energy, and contributions made here in the Salesforce Discussion Forums.
We’ll see you in the Trailblazer Community!
Thanks,
You can try below validation rule.
AND( NOT(ISNEW()), ISPICKVAL(PRIORVALUE(status),"VOID") )Please mark as Best Answer if above information was helpful.
Important Update - last chance
Heads up — this is your last chance to get your Trailblazer account set up and connected to your forums discussions on this site.Please take these steps before November 30, 2023, 11:59 p.m. PT to ensure your contributions follow you to the Trailblazer Community:
- If you’re not already a member of the Trailblazer Community, sign up for a Trailblazer account using the same login email address associated with your Developer Discussion Forums account. This is crucial.
- If you already have a Trailblazer account, and it’s using a different email address from your Developer Discussion Forums account, we recommend that you log in to the Trailblazer Community and connect your forums email address to your Trailblazer account.
We know that moving platforms can be a hassle, so we created a special forums users group, the Migration Support Hub, in the Trailblazer Community where you can find other forums users and get training videos, the latest information, and assistance.We want to thank you for all of your time, energy, and contributions made here in the Salesforce Discussion Forums.
We’ll see you in the Trailblazer Community!
Thanks,
I need help with a trigger
the trigger should be on account to check the assets and if ALL Assets where product family = "bla bla " and their status was "Canceled" the trigger should update bla bla Account stage (API Name = Account_Status__c ) to "Cancel".


Can you try the below
trigger AccountUpdateFromAssetProduct on Asset (after insert, after update) { List<Asset> assetList = Trigger.new; List<Id> idAccountList = new List<Id>(); for(Asset asset : assetList) { if(Trigger.isUpdate && asset.Product2.Family == Trigger.oldMap.get(asset.Id).Product2.Family && asset.Status == Trigger.oldMap.get(asset.Id).Status) continue; if(asset.AccountId == null) continue; idAccountList.add(asset.AccountId); } if(idAccountList.isEmpty()) return; List<Account> acctList = [SELECT Id, (SELECT Id FROM Assets WHERE Product2.Family = 'bla bla' AND Status = 'Canceled') FROM Account LIMIT 50]; for(Account acct : acctList) { if(acct.Assets.isEmpty()) continue; acct.Account_Status__c = 'Cancel'; } update acctList; }

tutorial to integrate Web service API using rest
Hello,
I am looking for a tutorial to integrate a wed service using rest method.
I have webservice which sends XML request and get XML response.
I am looking for tutorial to integrate a simple exsisitng web service, later i will modify for me.
thanks
I am looking for a tutorial to integrate a wed service using rest method.
I have webservice which sends XML request and get XML response.
I am looking for tutorial to integrate a simple exsisitng web service, later i will modify for me.
thanks

Hi Sandrine,
You just check below links it wil help for sure !!
https://developer.salesforce.com/page/Creating_REST_APIs_using_Apex_REST
https://developer.salesforce.com/blogs/developer-relations/2015/07/using-apex-to-integrate-salesforce.html
Also you can check the link posted by Sindhu for reference .
Let me know if it helps !!
Thanks
Manoj
You just check below links it wil help for sure !!
https://developer.salesforce.com/page/Creating_REST_APIs_using_Apex_REST
https://developer.salesforce.com/blogs/developer-relations/2015/07/using-apex-to-integrate-salesforce.html
Also you can check the link posted by Sindhu for reference .
Let me know if it helps !!
Thanks
Manoj
0% Code Coverage!?!
Hello all,
I've got a 'FundingAwardTrigger' on the FundingAward object (part of the Grantmaking package). When I run the test in Sandbox, I get 100% coverage.
When I validate the Inbound Change Set in Prod, I get the following error:
Code Coverage Failure
The following triggers have 0% code coverage. Each trigger must have at least 1% code coverage.
FundingAwardTrigger
Here's my test class:
Any tips appreciated!
I've got a 'FundingAwardTrigger' on the FundingAward object (part of the Grantmaking package). When I run the test in Sandbox, I get 100% coverage.
When I validate the Inbound Change Set in Prod, I get the following error:
Code Coverage Failure
The following triggers have 0% code coverage. Each trigger must have at least 1% code coverage.
FundingAwardTrigger
Here's my test class:
@isTest private class FundingAwardTriggerTest { @isTest static void testTriggerOnInsert() { // Create a test record for the insert operation FundingAward testAwardInsert = new FundingAward(Name = 'Test Insert', Amount = 100); // Start the test context Test.startTest(); // Perform the insert operation insert testAwardInsert; // Stop the test context Test.stopTest(); // Retrieve the inserted record and assert the result testAwardInsert = [SELECT Currency_Text__c FROM FundingAward WHERE Id = :testAwardInsert.Id]; System.assertEquals('One Hundred', testAwardInsert.Currency_Text__c, 'Trigger did not work as expected on insert'); } @isTest static void testTriggerOnUpdate() { // Create and insert a record for the update operation FundingAward testAwardUpdate = new FundingAward(Name = 'Test Update', Amount = 100); insert testAwardUpdate; // Start the test context Test.startTest(); // Perform the update operation testAwardUpdate.Amount = 200; update testAwardUpdate; // Stop the test context Test.stopTest(); // Retrieve the updated record and assert the result testAwardUpdate = [SELECT Currency_Text__c FROM FundingAward WHERE Id = :testAwardUpdate.Id]; System.assertEquals('Two Hundred', testAwardUpdate.Currency_Text__c, 'Trigger did not work as expected on update'); } @isTest static void testBulkOperation() { // Create multiple records for testing bulk operation List<FundingAward> awards = new List<FundingAward>(); for (Integer i = 1; i <= 10; i++) { awards.add(new FundingAward(Name = 'Bulk Test Award ' + i, Amount = i * 100)); } // Start the test context Test.startTest(); // Perform bulk insert insert awards; // Update the records for bulk update test for (Integer i = 0; i < awards.size(); i++) { awards[i].Amount += 1000; } // Perform bulk update update awards; // Stop the test context Test.stopTest(); // Optional: Add assertions here to validate the trigger's behavior on bulk operations } }Why would my test class show 100% in sandbox but 0% in prod?
Any tips appreciated!

Solved. The FundingAwardTriggerTest test class was omitted from my outbound change set. Including it solved the issue!

how to prevent record deletion based on a condition
Is there a way to prevent record deletion based on, for example, a status field without using code?




Hi Ahon,
Please find the below articles which might help you!
https://developer.salesforce.com/forums/?id=9062I000000g5mEQAQ
Using Flow to Prevent Record Deletion (https://salesforcetime.com/2023/09/03/using-flow-to-prevent-record-deletion/#:~:text=1%2D%20Create%20a%20record%2Dtriggered,flow%20should%20look%20like%20this.)
Please mark this as a best answer if this was useful. Thank you!
Important Update - what you need to do
As a reminder, on December 4, 2023 the Salesforce Discussion Forums will be removed from the Salesforce Developers website. These forums will shut down and all relevant discussions will migrate to the Trailblazer Community digital platform.
During the week starting November 20, you can view discussions, but not post new questions or responses. On December 4, you will no longer be able to access the discussion forums from the Salesforce Developers website.
Please take these steps before November 30, 2023, 11:59 p.m. PT to ensure your contributions follow you to the Trailblazer Community:
We know that moving platforms can be a hassle, so we created a special forums users group, the Migration Support Hub, in the Trailblazer Community where you can get training videos, information, and assistance.
We are here to help you through this transition!
Sincerely,
The Forums Support Team
Please find the below articles which might help you!
https://developer.salesforce.com/forums/?id=9062I000000g5mEQAQ
Using Flow to Prevent Record Deletion (https://salesforcetime.com/2023/09/03/using-flow-to-prevent-record-deletion/#:~:text=1%2D%20Create%20a%20record%2Dtriggered,flow%20should%20look%20like%20this.)
Please mark this as a best answer if this was useful. Thank you!
Important Update - what you need to do
As a reminder, on December 4, 2023 the Salesforce Discussion Forums will be removed from the Salesforce Developers website. These forums will shut down and all relevant discussions will migrate to the Trailblazer Community digital platform.
During the week starting November 20, you can view discussions, but not post new questions or responses. On December 4, you will no longer be able to access the discussion forums from the Salesforce Developers website.
Please take these steps before November 30, 2023, 11:59 p.m. PT to ensure your contributions follow you to the Trailblazer Community:
- If you’re not already a member of the Trailblazer Community, sign up for a Trailblazer account using the same login email address associated with your Developer Discussion Forums account. This is crucial.
- If you already have a Trailblazer account, and it’s using a different email address from your Developer Discussion Forums account, we recommend that you log in to the Trailblazer Community and connect your forums email address to your Trailblazer account.
We know that moving platforms can be a hassle, so we created a special forums users group, the Migration Support Hub, in the Trailblazer Community where you can get training videos, information, and assistance.
We are here to help you through this transition!
Sincerely,
The Forums Support Team

How to open the login page in the PicsArt mobile app?
I am using a PicsArt photo editing mobile application to use REST Services to provide functionality. It is also multilingual and depends on the current device language. How can I submit the language parameter in the OAUTH URL and then read that parameter to open the Login page in the respective language (English / Spanish and Portuguese).


I have just downloaded a version of PicsArt that can open the login page in the app, but for now I still don't know how to pass parameters in the OAUTH URL. This is the link I downloaded https://techbigs.com/pt/picsart.html
Filtering by the FeedItem "Body" field
Hey everyone,
I received a request to remove the chatter alerts on accounts that say "[NAME] updated this account by converting a lead." I'm sure you've received that complaint before as well, since those alerts can't be removed via the admin setup.
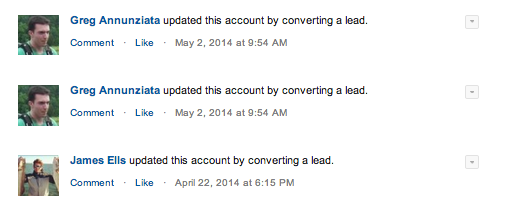
So I tried creating a trigger to delete those alerts when a lead is converted, but I get an error that says I can't filter by the FeedItem's "Body" field, which as far as I know, is the only way to distinguish these types of alerts. Here is the trigger I tried writing:
Is there any way to accomplish what I'm trying to do? Can I delete feeditems based on what's in the Body?
Thanks!
-Greg
I received a request to remove the chatter alerts on accounts that say "[NAME] updated this account by converting a lead." I'm sure you've received that complaint before as well, since those alerts can't be removed via the admin setup.
So I tried creating a trigger to delete those alerts when a lead is converted, but I get an error that says I can't filter by the FeedItem's "Body" field, which as far as I know, is the only way to distinguish these types of alerts. Here is the trigger I tried writing:
public class ClassDeleteConvertAlerts{ public void deleteAlerts(List<Lead> leads,Map<Id,Lead> oldLeads){ Set<String> convertedLeads = new Set<String>(); List<FeedItem> feedItemsToDelete = new List<FeedItem>(); FOR(Lead l : leads){ IF(l.IsConverted == TRUE && oldLeads.get(l.Id).IsConverted == FALSE){ convertedLeads.add(l.Id); } } IF(convertedLeads.size() > 0){ FOR(FeedItem item : [SELECT Id,Title FROM FeedItem WHERE Body LIKE '%updated this account by converting a lead%']){ feedItemsToDelete.add(item); } IF(feedItemsToDelete.size() > 0){ DELETE feedItemsToDelete; } } }
Is there any way to accomplish what I'm trying to do? Can I delete feeditems based on what's in the Body?
Thanks!
-Greg


Sometimes there is a slight delay in deletion otherwise it works great
Trigger DeleteAccountFeed on Lead (after update) { Set<String> convertedLeads = new Set<String>(); List<Id> convertedAccountList = new List<Id>(); FOR(Lead l : trigger.new){ IF(l.IsConverted == TRUE && trigger.oldmap.get(l.Id).IsConverted == FALSE){ convertedLeads.add(l.Id); convertedAccountList.add(l.convertedAccountId); } } IF(convertedLeads.size() > 0){ DeleteAccountFeedFutureClass.DeleteFeed(convertedAccountList); } } global class DeleteAccountFeedFutureClass { @future public static void DeleteFeed(List<ID> recordIds) { list<AccountFeed> listAccountFeedsToDelete = new list<AccountFeed>(); FOR(AccountFeed objFeed : [SELECT Id,Type,ParentId,(SELECT Id,FieldName FROM FeedTrackedChanges) FROM AccountFeed WHERE Type = 'TrackedChange' and ParentId in: recordIds]){ system.debug('ObjFeed....'+ObjFeed); FOR(FeedTrackedChange objChange : objFeed.FeedTrackedChanges){ system.debug('objChange...'+objChange); IF(objChange.FieldName == 'accountCreatedFromLead' || objChange.FieldName == 'accountUpdatedByLead'){ System.debug('Accound Feeds To Delete is ' + listAccountFeedsToDelete); listAccountFeedsToDelete.add(objFeed); System.debug('Accound Feeds To Delete is ' + listAccountFeedsToDelete); } } } DELETE listAccountFeedsToDelete; } }

How to log lead activity
Hi everyone,
I'm trying to log a call as an activity in a lead. This is the record I'm passing to the saveLog Method:
task = { entityApiName: 'Task', CallDisposition: 'Success', CallType: direction, Subject: (isCallOutbound ? 'Call to ' : 'Call from ') + displayName, ActivityDate: date, CompletedDateTime: date, IsClosed: true, Type: 'Call', TaskSubtype: 'Call', Status: 'Completed' }; if (recordType == 'Contact') { task.WhoId = popData.Id; } else { // Account / Lead task.WhatId = popData.Id; } cti.saveLog({ value: task, callback: ... });I tried changing the entityApiName to 'Activity', but that seemed wrong as I was getting the following error: "You don't have access to this record. Ask your administrator for help or to request access.".
I've also been getting an error related to the WhatId attribute: "Related To ID: id value of incorrect type: 00Q0900000OHl0UEAT".
Would apprieciate any help. Also a link to a detailed description of which fields can be passed to the saveLog method, if there is one.




Hi Patryk,
Please find the below help document.
https://help.formassembly.com/help/salesforce-error-id-value-of-incorrect-type#:~:text=What%20To%20Expect-,Introduction,Account%20ID%2C%20etc.).
Please mark this as a best answer if this was useful to you!
Important Update
We appreciate your participation in these Salesforce Discussion Forums! It’s active members like you that keep our amazing community going strong.
At this time, we want to give you a heads up that on December 4, 2023, the discussion forums will shut down and all relevant discussions will migrate to the Trailblazer Community digital platform. This move brings all conversations around Salesforce development together in one place and provides more opportunities for our broader community to connect and share. We will be removing outdated, obsolete, or spam content and migrating only relevant discussions to the Trailblazer Community digital platform.
Starting November 20, you can view discussions but not post new questions or responses. On December 2, you will no longer be able to access the discussion forums from the Salesforce Developers website.
Please take these steps before November 30, 2023, 11:59 p.m. PT to ensure your contributions follow you to the Trailblazer Community:
We will keep you up to date throughout the transition, and we look forward to seeing you joining the developer discussions in the Trailblazer Community!
Sincerely,
The Forums Support Team
Please find the below help document.
https://help.formassembly.com/help/salesforce-error-id-value-of-incorrect-type#:~:text=What%20To%20Expect-,Introduction,Account%20ID%2C%20etc.).
Please mark this as a best answer if this was useful to you!
Important Update
We appreciate your participation in these Salesforce Discussion Forums! It’s active members like you that keep our amazing community going strong.
At this time, we want to give you a heads up that on December 4, 2023, the discussion forums will shut down and all relevant discussions will migrate to the Trailblazer Community digital platform. This move brings all conversations around Salesforce development together in one place and provides more opportunities for our broader community to connect and share. We will be removing outdated, obsolete, or spam content and migrating only relevant discussions to the Trailblazer Community digital platform.
Starting November 20, you can view discussions but not post new questions or responses. On December 2, you will no longer be able to access the discussion forums from the Salesforce Developers website.
Please take these steps before November 30, 2023, 11:59 p.m. PT to ensure your contributions follow you to the Trailblazer Community:
- If you’re not already a member of the Trailblazer Community, sign up for a Trailblazer account using the same login email address associated with your Developer Discussion Forums account. This is crucial.
- If you already have a Trailblazer account, and it’s using a different email address from the one you used for your Developer Discussion Forums account, we recommend that you log in to the Trailblazer Community and connect your forums email address to your Trailblazer account.
We will keep you up to date throughout the transition, and we look forward to seeing you joining the developer discussions in the Trailblazer Community!
Sincerely,
The Forums Support Team
It looks you havent create custom Tabs while defining custom objects first you need to create custom Tab for new objects Creating Tab Setup -->App Set up---> Create---> Tabs Click on new Tab [drop down shows all untabed objects ] select your object, set Tab style , click Next select profiles Save Adding To App Setup -->App Set up---> Create---> Apps Click on edit, edit available tab section Save Done Thanks, Bala