-
ChatterFeed
-
68Best Answers
-
0Likes Received
-
2Likes Given
-
3Questions
-
440Replies
How can rollback database in apex?
I process insert to some tables:
I want rollback all, if occur error/
How can rollback database in apex?
public PageReference checkoutPaypal() { try { List<AccountUser__c> listacc = [SELECT id,AccountId__c FROM AccountUser__c WHERE email__c=:email LIMIT 1]; AccountUser__c a=new AccountUser__c(); Account acc=new Account(); if(listacc.size()>0) { a=listacc.get(0); } else { acc.Name=name; insert acc; //save value ok // a.Name=name; a.AccountId__c=acc.Id; a.Email__c=email; a.Status__c=0; a.Password__c='0'; insert a; } Contact con=new Contact(); con.LastName=name; con.Email=email; //con.Phone__c=phonenumber; con.AccountId=a.AccountId__c; insert con; // Contract hd=new Contract(); hd.AccountId=a.AccountId__c; hd.StartDate=system.today(); hd.ContractTerm=1; insert hd; Order od=new Order(); od.AccountId=a.AccountId__c; od.ContractId=hd.Id; od.EffectiveDate=system.today(); insert od; Double itotalMoney=0; if(ids !='') { string searchquery='select ProductCode,Name,ImageName__c,(Select UnitPrice,Pricebook2.Name From PricebookEntries where IsActive=True Order By UnitPrice ASC ) from Product2 where ProductCode in ('+SOQL_IDs+')'; List<Product2> MyProducts= Database.query(searchquery); List<OrderItem> lsDetail=new List<OrderItem>(); for(Integer i=0;i<MyProducts.size();i++) { String code=MyProducts.get(i).ProductCode; String sl=listCarts.get(code); OrderItem ode=new OrderItem(); ode.OrderId=od.Id; ode.Quantity=Double.valueOf(sl); ode.UnitPrice=MyProducts.get(i).PricebookEntries.get(0).UnitPrice; ode.Product2Id=MyProducts.get(i).Id; lsDetail.Add(ode); itotalMoney=itotalMoney+ ode.Quantity * ode.UnitPrice; } insert lsDetail; } Pagereference redirectedPage = New PageReference('/apex/payment?amount='+itotalMoney); return redirectedPage; } catch(DmlException e) { err=e.getMessage(); } return null; }Current if function occur error, some process still insert data ok.
I want rollback all, if occur error/
How can rollback database in apex?
-
- dai tran 6
- July 20, 2018
- Like
- 0
- Continue reading or reply
hello all , i have a question regarding the vf page
i want to create contact records using checkbox in vf page
Apex Controller:
public class AddmultipleContactsVF {
Contact Cont = new Contact();
public list<Contact> listContact{get;set;}
public Boolean IsSelected{set;get;}
public AddmultipleContactsVF()
{
listContact=new list<Contact>();
listContact.add(Cont);
}
Public void addContact()
{
Contact con = new Contact();
listContact.add(con);
}
public PageReference saveContact() {
for(Integer i=1; i<listContact.size(); i++)
{
if(Isselected==true){
upsert listContact;
}
}
return null;
}
public class wrapperContact{
public Boolean IsSelected{set;get;}
public wrapperContact(){
IsSelected=false;
}
}
}
VF Page:
<apex:page Controller="AddmultipleContactsVF">
<apex:form >
<apex:pageBlock >
<apex:pageBlockTable value="{!listContact}" var="con">
<apex:column headerValue="Contact LastName">
<apex:inputCheckbox value="{!IsSelected}" id="InputId"/>
<apex:inputField value="{!con.LastName}"/>
<apex:CommandButton value="+" action="{!addContact}"/>
</apex:column>
</apex:pageBlockTable>
<apex:pageBlockButtons >
<apex:CommandButton value="Save Contact" action="{!saveContact}"/>
</apex:pageBlockButtons>
</apex:pageBlock>
</apex:form>
</apex:page>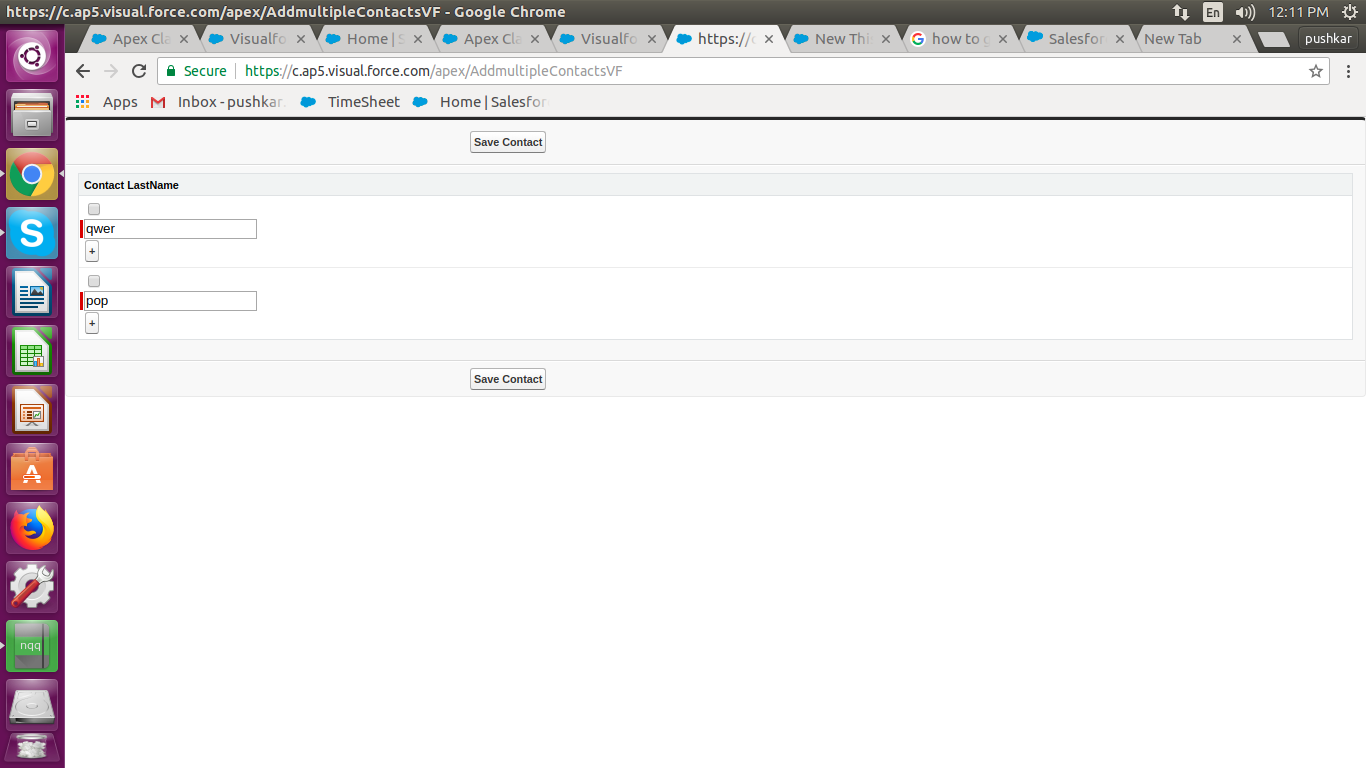
Apex Controller:
public class AddmultipleContactsVF {
Contact Cont = new Contact();
public list<Contact> listContact{get;set;}
public Boolean IsSelected{set;get;}
public AddmultipleContactsVF()
{
listContact=new list<Contact>();
listContact.add(Cont);
}
Public void addContact()
{
Contact con = new Contact();
listContact.add(con);
}
public PageReference saveContact() {
for(Integer i=1; i<listContact.size(); i++)
{
if(Isselected==true){
upsert listContact;
}
}
return null;
}
public class wrapperContact{
public Boolean IsSelected{set;get;}
public wrapperContact(){
IsSelected=false;
}
}
}
VF Page:
<apex:page Controller="AddmultipleContactsVF">
<apex:form >
<apex:pageBlock >
<apex:pageBlockTable value="{!listContact}" var="con">
<apex:column headerValue="Contact LastName">
<apex:inputCheckbox value="{!IsSelected}" id="InputId"/>
<apex:inputField value="{!con.LastName}"/>
<apex:CommandButton value="+" action="{!addContact}"/>
</apex:column>
</apex:pageBlockTable>
<apex:pageBlockButtons >
<apex:CommandButton value="Save Contact" action="{!saveContact}"/>
</apex:pageBlockButtons>
</apex:pageBlock>
</apex:form>
</apex:page>
-
- Pushkar Gupta
- July 19, 2018
- Like
- 0
- Continue reading or reply
OpportunityContactRole Permissions to Query
As a System Admin I can run this query on our production org in the developer console Query tab..
When I run the same query as System Admin in a sandbox I receive this error 'sObject type 'OpportunityContactRole' is not supported.'
I have compared access and permissions between teh orgs yet I can not figure out why.
I am able to create OpportunityContactRole in Apex code in both orgs yet I can't query from sandbox in apex to read the created object.
I need to get this to work so my unit tests will pass in both orgs.
Select Id, opportunityId, contact.account.Name, contact.Id, contact.Name, contact.FirstName, contact.LastName, contact.Phone, contact.Email, contact.LeadSource isPrimary, Role from OpportunityContactRole where opportunityId = '006xxxxxxxxx'
When I run the same query as System Admin in a sandbox I receive this error 'sObject type 'OpportunityContactRole' is not supported.'
I have compared access and permissions between teh orgs yet I can not figure out why.
I am able to create OpportunityContactRole in Apex code in both orgs yet I can't query from sandbox in apex to read the created object.
I need to get this to work so my unit tests will pass in both orgs.
-
- Maggie Longshore
- July 18, 2018
- Like
- 0
- Continue reading or reply
Apex code to view list of comments (Custom object) related to case
Hi All,
I created a custom object Name "Casecomments" related to Case object and below the Apex code I use to view all "Casecomments" created to a particular case by passing case id,
@RestResource(urlMapping='/CaseComments__c/*')
global with sharing class CaseComments__cListview{
@HttpGet
global static List<CaseComments__c> getCaseComments__cId() {
RestRequest req = RestContext.request;
RestResponse res = RestContext.response;
String Id = req.requestURI.substring(req.requestURI.lastIndexOf('/')+1);
List<CaseComments__c> result = [Select id from CaseComments__c where Case__c=:id];
return result;
}
}
I am facing this error,
Error: Compile Error: Invalid character in identifier: CaseComments__cListview at line 2 column 27
can someone help me on this.
thanks in advance.
I created a custom object Name "Casecomments" related to Case object and below the Apex code I use to view all "Casecomments" created to a particular case by passing case id,
@RestResource(urlMapping='/CaseComments__c/*')
global with sharing class CaseComments__cListview{
@HttpGet
global static List<CaseComments__c> getCaseComments__cId() {
RestRequest req = RestContext.request;
RestResponse res = RestContext.response;
String Id = req.requestURI.substring(req.requestURI.lastIndexOf('/')+1);
List<CaseComments__c> result = [Select id from CaseComments__c where Case__c=:id];
return result;
}
}
I am facing this error,
Error: Compile Error: Invalid character in identifier: CaseComments__cListview at line 2 column 27
can someone help me on this.
thanks in advance.
-
- ManojKumar Muthu
- July 18, 2018
- Like
- 0
- Continue reading or reply
Apex Code for to list view all notes related a Case.
Hi All ,
Hi,
I am trying to list all case created to particular account here the parameter is the CaseId, below the apex code,
below the code,
RestResource(urlMapping='/NotesListView/*')
global with sharing class NotesListView{
@HttpGet
global static List<Note> getNoteById() {
RestRequest req = RestContext.request;
RestResponse res = RestContext.response;
String Id= req.requestURI.substring(req.requestURI.lastIndexOf('/')+1);
List<Note> result = [Select Id, Title, Body from Note where ParentId= :Id];
return result;
}
}
Where I Pass CaseId in ParentId.
Hi,
I am trying to list all case created to particular account here the parameter is the CaseId, below the apex code,
below the code,
RestResource(urlMapping='/NotesListView/*')
global with sharing class NotesListView{
@HttpGet
global static List<Note> getNoteById() {
RestRequest req = RestContext.request;
RestResponse res = RestContext.response;
String Id= req.requestURI.substring(req.requestURI.lastIndexOf('/')+1);
List<Note> result = [Select Id, Title, Body from Note where ParentId= :Id];
return result;
}
}
Where I Pass CaseId in ParentId.
-
- ManojKumar Muthu
- July 18, 2018
- Like
- 0
- Continue reading or reply
SOQL 101 issue due to query in for loop. Need help
I have the following trigger which is poorly written and causing during data load and record update for certain records. I am trying to fix the query issue at list for now. But I am not expert like you guys so need help.
Following Code trying to update but getting- Unexpected token issue.
Original Code:
Following Code trying to update but getting- Unexpected token issue.
List <Investor_Position__c> listIvPost = [Select Id,Investor_Position_Account__c,Is_Active__c, Current_Commitments_Functional__c, Source_of_Capital__c, Investment_Vehicle__r.Id, Investment_Vehicle__r.Number_of_Current_Investors__c, Investment_Vehicle__r.Number_of_Historical_Investors__c from Investor_Position__c where Investment_Vehicle__r.Id IN InvestmentVehicleIds]; for(Id id : InvestmentVehicleIds) { for( Investor_Position__c obj :listIvPost ) { if(obj.Is_Active__c == TRUE && obj.Source_of_Capital__c != null) {
Original Code:
trigger InvestorPositionTrigger on Investor_Position__c (after insert, after update, after delete) { Set<Id> numofhistinvestors = new Set<Id>(); Set<Id> numofcurrentinvestors = new Set<Id>(); Set<Id> InvestmentVehicleIds = new Set<Id>(); List<Investment_Vehicle__c> lstUpdateInvVehicle = new List<Investment_Vehicle__c>(); if(trigger.isafter) { if(trigger.isinsert || trigger.isupdate) { for(Investor_Position__c inv : Trigger.New) { InvestmentVehicleIds.add(inv.Investment_Vehicle__c); } } if(trigger.isdelete) { for(Investor_Position__c inv : Trigger.old) { InvestmentVehicleIds.add(inv.Investment_Vehicle__c); } } for(Id id : InvestmentVehicleIds) { for( Investor_Position__c obj : [Select Id,Investor_Position_Account__c,Is_Active__c, Current_Commitments_Functional__c, Source_of_Capital__c, Investment_Vehicle__r.Id, Investment_Vehicle__r.Number_of_Current_Investors__c, Investment_Vehicle__r.Number_of_Historical_Investors__c from Investor_Position__c where Investment_Vehicle__r.Id =:id]) { if(obj.Is_Active__c == TRUE && obj.Source_of_Capital__c != null) { if(obj.Current_Commitments_Functional__c > 0 && (obj.Source_of_Capital__c.contains('Limited Partners') || obj.Source_of_Capital__c.contains('Operating Partner'))) { numofcurrentinvestors.add(obj.Investor_Position_Account__c); } if(obj.Source_of_Capital__c.contains('Limited Partners') || obj.Source_of_Capital__c.contains('Operating Partner')) { numofhistinvestors.add(obj.Investor_Position_Account__c); } } } Investment_Vehicle__c invveh = new Investment_Vehicle__c(); invveh.Id = id; invveh.Number_of_Historical_Investors__c = numofhistinvestors.size(); invveh.Number_of_Current_Investors__c = numofcurrentinvestors.size(); lstUpdateInvVehicle.add(invveh); numofcurrentinvestors.clear(); numofhistinvestors.clear(); } try { if(lstUpdateInvVehicle.size() > 0) { update lstUpdateInvVehicle; } } catch(exception ex) { for (Investor_Position__c obj : trigger.new) { obj.addError(ex.getmessage()); } } } if(Trigger.isInsert){ ConfigurableRollup.rollup(trigger.new); } if(Trigger.isUpdate){ system.debug('when is update------'); ConfigurableRollup.rollup(trigger.new, Trigger.OldMap); } if(Trigger.isDelete){ ConfigurableRollup.rollup(trigger.old); } }
-
- Sunny Solanki 11
- July 17, 2018
- Like
- 0
- Continue reading or reply
Trigger for Updation
Hi All,
With thebelow code i am trying to update Billing Item Qty=0 ,Based on Document Type in Billing document in salsforce .
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
trigger UpdateQty on BillingDoc__c (after insert,after update) {
Set<id> setOfParentId = new Set<Id>();
for(BillingDoc__c BD:trigger.new){
List<BillingItem__c> listBI=new List<BillingItem__c>([Select Id,BillingDocumentId__c,Quantity__c from BillingItem__c where BillingDocumentId__c in: setOfParentId]);
if ( listBI.size() > 0 )
for(BillingItem__c BI : listBI){
if(BD.DocumentTypeCode__c=='ZG2')
{
BI.Quantity__c = 0;
}
listBI.add(BI);
}
}
}
But it is not updating the Quatity=0.
please suggest me if it need some updation.
Thanks,
Silpi
With thebelow code i am trying to update Billing Item Qty=0 ,Based on Document Type in Billing document in salsforce .
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
trigger UpdateQty on BillingDoc__c (after insert,after update) {
Set<id> setOfParentId = new Set<Id>();
for(BillingDoc__c BD:trigger.new){
List<BillingItem__c> listBI=new List<BillingItem__c>([Select Id,BillingDocumentId__c,Quantity__c from BillingItem__c where BillingDocumentId__c in: setOfParentId]);
if ( listBI.size() > 0 )
for(BillingItem__c BI : listBI){
if(BD.DocumentTypeCode__c=='ZG2')
{
BI.Quantity__c = 0;
}
listBI.add(BI);
}
}
}
But it is not updating the Quatity=0.
please suggest me if it need some updation.
Thanks,
Silpi
-
- Silpi roy 16
- July 17, 2018
- Like
- 0
- Continue reading or reply
Populate custom lookup field from custom URL button with a visualforce page
I created a custom button on the opportunity that goes to a visualforce page. That visualforce page creates a specifc case type and allows attachments. My goal is to populate the "Opportunity__c" lookup field on the Case with the opportunity Id. I have tried editing the button so the URL is this:
/apex/InfoSecCase?00N0v000002BPBH={!Opportunity.Id}
When clicking the button, I can see the parameter is passed through:

But when I click Save, the field isn't populated. Could someone please help point me in the right direction?
Thank you,
/apex/InfoSecCase?00N0v000002BPBH={!Opportunity.Id}
When clicking the button, I can see the parameter is passed through:
But when I click Save, the field isn't populated. Could someone please help point me in the right direction?
Thank you,
-
- Kerry Proksel
- July 16, 2018
- Like
- 0
- Continue reading or reply
Write test class for Scheduled Apex
Hello, can someone please help me write a test for this class?
global class CaseGenerator implements Schedulable { global void execute(SchedulableContext ctx) { createInvoiceCase(); } public void createInvoiceCase() { List<Case> newCaseList = new List<Case>(); List<Billing_Unit__c> invoiceCases = [SELECT Id, Contract__c, Name FROM Billing_Unit__c WHERE Invoicing_Date__c = TODAY AND Unlimited__c = TRUE]; if(invoiceCases != null){ for(Billing_Unit__c bgut : invoiceCases){ Case cseobj = new Case( subject='Rechnung erstellen: '+bgut.Name, Contract__c=bgut.Contract__c, Automatically_generated__c=TRUE, Billing_Unit__c=bgut.Id, Status='New'); newCaseList.add(cseobj); } insert newCaseList; } } }
This is what I have so far, but I do not think I am going in the right direction:
public class CaseGeneratorTest{ public static String CRON_EXP '0 0 6 * * *'; static testMethod void testScheduledJob(){ List<Case> newCaseList = new List<Case>(); Test.startTest(); invoiceCasesListTest invcs = new List<Case> invcs.Invoicing_date__c = TODAY, invcs.Unlimited__c = TRUE; ...
-
- linda b
- July 12, 2018
- Like
- 0
- Continue reading or reply
retrieve community url on non community triggered emails ?
how to retrieve community url on non community triggered emails ?
-
- maddy27
- July 11, 2018
- Like
- 0
- Continue reading or reply
can someone help me to cover code 100%. I'm getting 66% for my test class.
can someone help me to cover code 100%. I'm getting 66% for my test class.
Apex class:
global class ValidateBusinessHours implements Process.Plugin {
global Process.PluginResult invoke(Process.PluginRequest request) {
DateTime dt = (DateTime) request.inputParameters.get('dt');
BusinessHours bh = [SELECT Id,name FROM BusinessHours WHERE Name='Zelle Flow' limit 1];
System.debug('Business Hours:'+bh);
System.debug('Current Date Time'+dt);
System.debug('Current Date time is in Business hours::'+BusinessHours.isWithin(bh.id, dt));
Map<String,boolean> result = new Map<String,boolean>();
result.put('value',BusinessHours.isWithin(bh.id, dt));
return new Process.PluginResult(result);
}
// Returns the describe information for the interface
global Process.PluginDescribeResult describe() {
Process.PluginDescribeResult result = new Process.PluginDescribeResult();
result.Name = 'businessHoursplugin';
result.Tag = 'BusinessHours';
result.inputParameters = new
List<Process.PluginDescribeResult.InputParameter>{
new Process.PluginDescribeResult.InputParameter('dt',
Process.PluginDescribeResult.ParameterType.DateTime, true)
};
result.outputParameters = new
List<Process.PluginDescribeResult.OutputParameter>{
new Process.PluginDescribeResult.OutputParameter(
'value',
Process.PluginDescribeResult.ParameterType.boolean)
};
return result;
}
}
Test Class:
@isTest
private class ValidateBusinessHours_Test{
@testSetup
static void setupTestData(){
test.startTest();
BusinessHours businesshours_Obj = new BusinessHours(Name = 'Name220', IsActive = true, IsDefault = false, TimeZoneSidKey = 'Pacific/Kiritimati');
test.stopTest();
}
static testMethod void test_PluginRequest_UseCase1(){
List<BusinessHours> businesshours_Obj = [SELECT Id,Name from BusinessHours];
System.assertEquals(true,businesshours_Obj.size()>0);
ValidateBusinessHours obj01 = new ValidateBusinessHours();
//obj01.PluginRequest(new Process.PluginRequest());
}
static testMethod void test_describe_UseCase1(){
List<BusinessHours> businesshours_Obj = [SELECT Id,Name from BusinessHours];
System.assertEquals(true,businesshours_Obj.size()>0);
ValidateBusinessHours obj01 = new ValidateBusinessHours();
obj01.describe();
}
static testMethod void test_describe_UseCase2(){
List<BusinessHours> businesshours_Obj = [SELECT Id,Name from BusinessHours];
System.assertEquals(true,businesshours_Obj.size()>0);
ValidateBusinessHours obj01 = new ValidateBusinessHours();
//businesshours_Obj[0].id='';
businesshours_Obj[0].Name='hgvhv';
obj01.describe();
}
}
Apex class:
global class ValidateBusinessHours implements Process.Plugin {
global Process.PluginResult invoke(Process.PluginRequest request) {
DateTime dt = (DateTime) request.inputParameters.get('dt');
BusinessHours bh = [SELECT Id,name FROM BusinessHours WHERE Name='Zelle Flow' limit 1];
System.debug('Business Hours:'+bh);
System.debug('Current Date Time'+dt);
System.debug('Current Date time is in Business hours::'+BusinessHours.isWithin(bh.id, dt));
Map<String,boolean> result = new Map<String,boolean>();
result.put('value',BusinessHours.isWithin(bh.id, dt));
return new Process.PluginResult(result);
}
// Returns the describe information for the interface
global Process.PluginDescribeResult describe() {
Process.PluginDescribeResult result = new Process.PluginDescribeResult();
result.Name = 'businessHoursplugin';
result.Tag = 'BusinessHours';
result.inputParameters = new
List<Process.PluginDescribeResult.InputParameter>{
new Process.PluginDescribeResult.InputParameter('dt',
Process.PluginDescribeResult.ParameterType.DateTime, true)
};
result.outputParameters = new
List<Process.PluginDescribeResult.OutputParameter>{
new Process.PluginDescribeResult.OutputParameter(
'value',
Process.PluginDescribeResult.ParameterType.boolean)
};
return result;
}
}
Test Class:
@isTest
private class ValidateBusinessHours_Test{
@testSetup
static void setupTestData(){
test.startTest();
BusinessHours businesshours_Obj = new BusinessHours(Name = 'Name220', IsActive = true, IsDefault = false, TimeZoneSidKey = 'Pacific/Kiritimati');
test.stopTest();
}
static testMethod void test_PluginRequest_UseCase1(){
List<BusinessHours> businesshours_Obj = [SELECT Id,Name from BusinessHours];
System.assertEquals(true,businesshours_Obj.size()>0);
ValidateBusinessHours obj01 = new ValidateBusinessHours();
//obj01.PluginRequest(new Process.PluginRequest());
}
static testMethod void test_describe_UseCase1(){
List<BusinessHours> businesshours_Obj = [SELECT Id,Name from BusinessHours];
System.assertEquals(true,businesshours_Obj.size()>0);
ValidateBusinessHours obj01 = new ValidateBusinessHours();
obj01.describe();
}
static testMethod void test_describe_UseCase2(){
List<BusinessHours> businesshours_Obj = [SELECT Id,Name from BusinessHours];
System.assertEquals(true,businesshours_Obj.size()>0);
ValidateBusinessHours obj01 = new ValidateBusinessHours();
//businesshours_Obj[0].id='';
businesshours_Obj[0].Name='hgvhv';
obj01.describe();
}
}
-
- Sai Theja
- July 10, 2018
- Like
- 0
- Continue reading or reply
Hi Friends..Am struggling to write Test class for below code for Getting Lead
Hi friends...
Am struggling to write Test class for below code for Getting Lead...please help me t's urgent..
public static Lead getLead(String recordId) {
Lead lead = [Select id,Status,Status__c from Lead where id=:recordId];
return lead;
}
Thanks
Chandu
Am struggling to write Test class for below code for Getting Lead...please help me t's urgent..
public static Lead getLead(String recordId) {
Lead lead = [Select id,Status,Status__c from Lead where id=:recordId];
return lead;
}
Thanks
Chandu
-
- chandrashekar Jangiti
- July 10, 2018
- Like
- 0
- Continue reading or reply
ConnectApi.ConnectApiException: Must have value for exactly one of the following: username, id
I am not sure if this is a new change from v43.0 but I am unable to get my Apex to post to chatter.
I have an "Administrator" case RecordType and if it has children case, once and update is processed on the child case, a chatter post will be posted to the parent with an @menttion to the owner.
CODE:
Other threads I have poured over seem to hint that it is a user permissions issue, but I am testing with the supper admin in a sandbox, so not sure what else I would need access to.
Seems like over the versions of the API there has been a varieance of signatures for the postFeedElement() method, so wondering if my issue is there.
Any advice would be much apreciated.
Thanks!
I have an "Administrator" case RecordType and if it has children case, once and update is processed on the child case, a chatter post will be posted to the parent with an @menttion to the owner.
CODE:
if (Trigger.isUpdate) { if (newCase.ParentId != null) { // If case has a parent case for (Case c : ParentAdminCases) { //<-- bulk soql'ed outside of trigger loop. if(c.ParentId == newCase.ParentId) { // If cases parent is of type admin. ConnectApi.FeedItemInput feedItemInput = new ConnectApi.FeedItemInput(); ConnectApi.MentionSegmentInput mentionSegmentInput = new ConnectApi.MentionSegmentInput(); ConnectApi.MessageBodyInput messageBodyInput = new ConnectApi.MessageBodyInput(); ConnectApi.TextSegmentInput textSegmentInput = new ConnectApi.TextSegmentInput(); messageBodyInput.messageSegments = new List<ConnectApi.MessageSegmentInput>(); mentionSegmentInput.id = newCase.Parent.OwnerId; // Set @mention messageBodyInput.messageSegments.add(mentionSegmentInput); textSegmentInput.text = 'Blah blah blah blha'; messageBodyInput.messageSegments.add(textSegmentInput); feedItemInput.body = messageBodyInput; feedItemInput.feedElementType = ConnectApi.FeedElementType.FeedItem; feedItemInput.subjectId = newCase.ParentId; ConnectApi.FeedElement feedElement = ConnectApi.ChatterFeeds.postFeedElement(Network.getNetworkId(), feedItemInput); break; } } } }My code compiles, but when I attempt to edit the child case I get the error in the description:
- ConnectApi.ConnectApiException: Must have value for exactly one of the following: username, id
Other threads I have poured over seem to hint that it is a user permissions issue, but I am testing with the supper admin in a sandbox, so not sure what else I would need access to.
Seems like over the versions of the API there has been a varieance of signatures for the postFeedElement() method, so wondering if my issue is there.
Any advice would be much apreciated.
Thanks!
-
- Daniel Watson 21
- July 09, 2018
- Like
- 0
- Continue reading or reply
Test class for controller with extension?
I'm trying to write a test class for a controller with an extension, but I'm not really sure where to start. I've looked at the SF documentation, but I'm not understanding. Can anyone help me with this? Thanks!
public class selectWarehouse { private final Purchase_Requisition__c pr; public string selectedWarehouse{get;set;} public selectWarehouse(ApexPages.StandardController stdController) { this.pr = (Purchase_Requisition__c)stdController.getRecord(); } public list<SelectOption> getWarehousenames() { List<SelectOption> options = new List<SelectOption>(); for (Warehouse__c w : [select name from Warehouse__c order by name desc limit 100]) { options.add(new SelectOption(w.name, w.name)); } return options; } public PageReference saveWarehouse(){ pr.Warehouse_Text__c = selectedWarehouse; update pr; return new PageReference('/'+pr.Id); } }
-
- Adriana Reyes 7
- July 06, 2018
- Like
- 0
- Continue reading or reply
Can't test Trigger isUpdate
Hello,
I'm a beginner with triggers and test classes.
I can't manage to cover the trigger below.
Thanks for your help.
Trigger EventBeforeUpdate on Event (before insert, before update){
If(AvoidRecursion.isFirstRun()){
If(!AdminSettings__c.getInstance().DisableTg__c){
For (Event myEvent: trigger.new){
If(Trigger.isInsert) {
}
If(Trigger.isUpdate){
If(UserInfo.getUserId()!= myEvent.OwnerId && UserInfo.getUserId()!= '00524000001WRNAAA4'&& myEvent.RecordTypeId == '0121o00000113r3' && trigger.oldMap.get(myEvent.Id).OwnerId == myEvent.OwnerId)
{
myEvent.adderror('ERROR1');
}
If(UserInfo.getUserId()!= myEvent.OwnerId && myEvent.RecordTypeId != '0121o00000113r3' && trigger.oldMap.get(myEvent.Id).OwnerId == myEvent.OwnerId)
{
myEvent.adderror('ERROR2.');
}
}
}
}
}
}
My Test :
@isTest
public class EventBeforeUpdateTest{
private static User makeStandardUser(String p_firstName, String p_lastName) {
User standardUser = new User();
standardUser.LastName = p_lastName;
standardUser.FirstName = p_firstName;
standardUser.Alias = 'JPJ';
standardUser.CommunityNickname = p_firstName + ' ' + p_lastName;
standardUser.UserName = p_firstName + p_lastName + '@softwaymedical.fr';
standardUser.Email = 'dev@dev.com';
standardUser.TimeZoneSidKey = 'America/Los_Angeles';
standardUser.LocaleSidKey = 'en_US';
standardUser.EmailEncodingKey = 'UTF-8';
standardUser.LanguageLocaleKey = 'en_US';
standardUser.profileId = '00e24000000tqX0';
return standardUser;
}
static testMethod void createAndUpdateEventTest() {
User User1 = makeStandardUser('JUL', 'JAV');
User User2 = makeStandardUser('CGL', 'GUI');
Insert User1;
Insert User2;
AdminSettings__c setting = new AdminSettings__c(SetupOwnerId=Userinfo.getUserId());
setting.DisablePb__c = True;
Insert setting;
Event myEvent1 = new Event();
myEvent1.RecordTypeId = '0121o00000113r4';
myEvent1.Subject = 'New Event Standard';
myEvent1.StartDateTime = system.Now();
myEvent1.EndDateTime = system.Now();
myEvent1.OwnerId = User1.Id;
Insert myEvent1;
system.runAs(User2){
try{
Update myEvent1;
}catch(Exception ex){
}
}
system.runAs(User1){
try{
Update myEvent1;
}catch(Exception ex){
}
}
}
}
I'm a beginner with triggers and test classes.
I can't manage to cover the trigger below.
Thanks for your help.
Trigger EventBeforeUpdate on Event (before insert, before update){
If(AvoidRecursion.isFirstRun()){
If(!AdminSettings__c.getInstance().DisableTg__c){
For (Event myEvent: trigger.new){
If(Trigger.isInsert) {
}
If(Trigger.isUpdate){
If(UserInfo.getUserId()!= myEvent.OwnerId && UserInfo.getUserId()!= '00524000001WRNAAA4'&& myEvent.RecordTypeId == '0121o00000113r3' && trigger.oldMap.get(myEvent.Id).OwnerId == myEvent.OwnerId)
{
myEvent.adderror('ERROR1');
}
If(UserInfo.getUserId()!= myEvent.OwnerId && myEvent.RecordTypeId != '0121o00000113r3' && trigger.oldMap.get(myEvent.Id).OwnerId == myEvent.OwnerId)
{
myEvent.adderror('ERROR2.');
}
}
}
}
}
}
My Test :
@isTest
public class EventBeforeUpdateTest{
private static User makeStandardUser(String p_firstName, String p_lastName) {
User standardUser = new User();
standardUser.LastName = p_lastName;
standardUser.FirstName = p_firstName;
standardUser.Alias = 'JPJ';
standardUser.CommunityNickname = p_firstName + ' ' + p_lastName;
standardUser.UserName = p_firstName + p_lastName + '@softwaymedical.fr';
standardUser.Email = 'dev@dev.com';
standardUser.TimeZoneSidKey = 'America/Los_Angeles';
standardUser.LocaleSidKey = 'en_US';
standardUser.EmailEncodingKey = 'UTF-8';
standardUser.LanguageLocaleKey = 'en_US';
standardUser.profileId = '00e24000000tqX0';
return standardUser;
}
static testMethod void createAndUpdateEventTest() {
User User1 = makeStandardUser('JUL', 'JAV');
User User2 = makeStandardUser('CGL', 'GUI');
Insert User1;
Insert User2;
AdminSettings__c setting = new AdminSettings__c(SetupOwnerId=Userinfo.getUserId());
setting.DisablePb__c = True;
Insert setting;
Event myEvent1 = new Event();
myEvent1.RecordTypeId = '0121o00000113r4';
myEvent1.Subject = 'New Event Standard';
myEvent1.StartDateTime = system.Now();
myEvent1.EndDateTime = system.Now();
myEvent1.OwnerId = User1.Id;
Insert myEvent1;
system.runAs(User2){
try{
Update myEvent1;
}catch(Exception ex){
}
}
system.runAs(User1){
try{
Update myEvent1;
}catch(Exception ex){
}
}
}
}
-
- Cindy GUILLET
- July 06, 2018
- Like
- 0
- Continue reading or reply
Apex Code for customer Rest API
Hi,
I am trying to list all case created to particular account here the parameter is the AccountId, below the apex code,
@RestResource(urlMapping='/CaseAccountid/*')
global with sharing class CaseAccountid{
@HttpGet
global static List<Case> getCaseById() {
RestRequest req = RestContext.request;
RestResponse res = RestContext.response;
String emailAddress = req.requestURI.substring(req.requestURI.lastIndexOf('/')+1);
List<case> result = [Select id, subject, status, Version__c, type, priority, AccountId, OwnerId, CSM_ID__c, ContactEmail from Case where AccountId = :ID];
return result;
}
}
I get Error: Compile Error: Variable does not exist: AccountId at line 9 column 170
Can someone help me on this asap.
Thanks in advance.
-
- ManojKumar Muthu
- July 06, 2018
- Like
- 0
- Continue reading or reply
Hi, I want to send the records link of any objects(Contact,Account etc) by an apex trigger in an email
how to insert the record link.
The trigger is below , please give the code to add the hyper link of the record
-------
trigger DocLink on Contact (before insert) {
// Step 0: Create a master list to hold the emails we'll send
List<Messaging.SingleEmailMessage> mails =
new List<Messaging.SingleEmailMessage>();
for (Contact myContact : Trigger.new) {
if (myContact.Email != null && myContact.FirstName != null) {
// Step 1: Create a new Email
Messaging.SingleEmailMessage mail =
new Messaging.SingleEmailMessage();
// Step 2: Set list of people who should get the email
List<String> sendTo = new List<String>();
sendTo.add(myContact.Email);
mail.setToAddresses(sendTo);
// Step 3: Set who the email is sent from
mail.setReplyTo('ikrammohiuddin@gmail.com');
mail.setSenderDisplayName('Mohammed Ikram Moinuddin');
// (Optional) Set list of people who should be CC'ed
List<String> ccTo = new List<String>();
ccTo.add('ikrammohiuddin@gmail.com');
mail.setCcAddresses(ccTo);
// Step 4. Set email contents - you can use variables!
mail.setSubject('Link of the Contact '+ myContact.FirstName);
String body = 'Dear ' + myContact.FirstName + ', ';
body += 'I confess this will come as a surprise to you.';
body += 'I am good';
body += 'to a persom. Please respond with ';
body += 'your details.';
mail.setHtmlBody(body);
// Step 5. Add your email to the master list
mails.add(mail);
}
}
// Step 6: Send all emails in the master list
Messaging.sendEmail(mails);
}
The trigger is below , please give the code to add the hyper link of the record
-------
trigger DocLink on Contact (before insert) {
// Step 0: Create a master list to hold the emails we'll send
List<Messaging.SingleEmailMessage> mails =
new List<Messaging.SingleEmailMessage>();
for (Contact myContact : Trigger.new) {
if (myContact.Email != null && myContact.FirstName != null) {
// Step 1: Create a new Email
Messaging.SingleEmailMessage mail =
new Messaging.SingleEmailMessage();
// Step 2: Set list of people who should get the email
List<String> sendTo = new List<String>();
sendTo.add(myContact.Email);
mail.setToAddresses(sendTo);
// Step 3: Set who the email is sent from
mail.setReplyTo('ikrammohiuddin@gmail.com');
mail.setSenderDisplayName('Mohammed Ikram Moinuddin');
// (Optional) Set list of people who should be CC'ed
List<String> ccTo = new List<String>();
ccTo.add('ikrammohiuddin@gmail.com');
mail.setCcAddresses(ccTo);
// Step 4. Set email contents - you can use variables!
mail.setSubject('Link of the Contact '+ myContact.FirstName);
String body = 'Dear ' + myContact.FirstName + ', ';
body += 'I confess this will come as a surprise to you.';
body += 'I am good';
body += 'to a persom. Please respond with ';
body += 'your details.';
mail.setHtmlBody(body);
// Step 5. Add your email to the master list
mails.add(mail);
}
}
// Step 6: Send all emails in the master list
Messaging.sendEmail(mails);
}
-
- Mohammed Ikram 7
- July 05, 2018
- Like
- 0
- Continue reading or reply
After insert trigger error: maximum trigger depth exceeded
Hi,
I have a problem with an apex class. I have created an object related to opportunity, “billing detail”.
The result I want to reach is the creation of multiple child object, Billing details, according the number of months that I Have from the difference to “End of billing” and “Created date” divided for a number. The number is 2 if the billing is two-monthly, 1 if is monthly, 3 if is quarterly, 12 for annual. I have an after insert trigger that call an apex class(Helper).
Apex class:
"Error: Invalid Data.
Review all error messages below to correct your data.
Apex trigger bd caused an unexpected exception, contact your administrator: bd: execution of AfterInsert caused by: System.DmlException: Insert failed. First exception on row 0; first error: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY, bd: maximum trigger depth exceeded Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert: []: Class.Helper.createBD: line 137, column 1"
The error appears only for i=2, in other cases it works well; I don't understand why and how I can resolve.
Can you help me?
Thanks
I have a problem with an apex class. I have created an object related to opportunity, “billing detail”.
The result I want to reach is the creation of multiple child object, Billing details, according the number of months that I Have from the difference to “End of billing” and “Created date” divided for a number. The number is 2 if the billing is two-monthly, 1 if is monthly, 3 if is quarterly, 12 for annual. I have an after insert trigger that call an apex class(Helper).
Apex class:
public class Helper { public static void createBD(Billing_Detail__c bd){ //method to create billing details List <Billing_Detail__c> listBD = new List <Billing_Detail__c> (); Integer i=0; Integer K=0; if (bd.Billing_Period__c=='two-monthly') { k=2; // convert from dateTime to date Date myDate = date.newinstance(bd.CreatedDate.year(), bd.CreatedDate.month(), bd.CreatedDate.day()); if(((bd.Billing_Type__c=='FEE')||(bd.Billing_Type__c=='PPU'))&& bd.Billing_Period__c=='two-monthly'){ i= myDate.monthsBetween(bd.End_of_Billing__c)/2;//number of month divided 2 } //creation for(Integer n =0; n<i-1; n++){//-1 I need of this otherwise it creates one more instance if(i!=1){ Billing_Detail__c newBd = new Billing_Detail__c (); newBd.Amount__c=bd.Amount__c; newBd.Billing_Date__c=bd.Billing_Date__c; newBd.Billing_Type__c=bd.Billing_Type__c; newBd.Billing_Status__c=bd.Billing_Status__c; newBd.Billing_Period__c= bd.Billing_Period__c; newBd.Product_Line__c=bd.Product_Line__c; newBd.Product__c=bd.Product__c; newBd.End_of_Billing__c=bd.End_of_Billing__c; newBd.Opportunity_billing__c=bd.Opportunity_billing__c; newBd.Monthly_Forecast__c= bd.Monthly_Forecast__c.addMonths(k); k+=2; listBD.add(newBd); } } insert listBD; } // I reapet for other cases else if (bd.Billing_Period__c=='quarterly') { k=3; Date myDate = date.newinstance(bd.CreatedDate.year(), bd.CreatedDate.month(), bd.CreatedDate.day()); if (((bd.Billing_Type__c=='FEE')||(bd.Billing_Type__c=='PPU'))&& bd.Billing_Period__c=='quarterly'){ i= myDate.monthsBetween(bd.End_of_Billing__c)/3; } for(Integer n =0; n<i-1; n++){ if(i!=1){ Billing_Detail__c newBd = new Billing_Detail__c (); newBd.Amount__c=bd.Amount__c; newBd.Billing_Date__c=bd.Billing_Date__c; newBd.Billing_Type__c=bd.Billing_Type__c; newBd.Billing_Status__c=bd.Billing_Status__c; newBd.Billing_Period__c= bd.Billing_Period__c; newBd.Product_Line__c=bd.Product_Line__c; newBd.Product__c=bd.Product__c; newBd.End_of_Billing__c=bd.End_of_Billing__c; newBd.Opportunity_billing__c=bd.Opportunity_billing__c; newBd.Monthly_Forecast__c= bd.Monthly_Forecast__c.addMonths(k);//parametro k per data scalata k+=3; listBD.add(newBd); } } insert listBD; } else if (bd.Billing_Period__c=='annual') { k=12; Date myDate = date.newinstance(bd.CreatedDate.year(), bd.CreatedDate.month(), bd.CreatedDate.day()); if (((bd.Billing_Type__c=='FEE')||(bd.Billing_Type__c=='PPU'))&& bd.Billing_Period__c=='annual'){ i= myDate.monthsBetween(bd.End_of_Billing__c)/12; } for(Integer n =0; n<i-1; n++){ if(i!=1){ Billing_Detail__c newBd = new Billing_Detail__c (); newBd.Amount__c=bd.Amount__c; newBd.Billing_Date__c=bd.Billing_Date__c; newBd.Billing_Type__c=bd.Billing_Type__c; newBd.Billing_Status__c=bd.Billing_Status__c; newBd.Billing_Period__c= bd.Billing_Period__c; newBd.Product_Line__c=bd.Product_Line__c; newBd.Product__c=bd.Product__c; newBd.End_of_Billing__c=bd.End_of_Billing__c; newBd.Opportunity_billing__c=bd.Opportunity_billing__c; newBd.Monthly_Forecast__c= bd.Monthly_Forecast__c.addMonths(k); k+=12; listBD.add(newBd); } } insert listBD; } else if (bd.Billing_Period__c=='monthly'){ Date myDate = date.newinstance(bd.CreatedDate.year(), bd.CreatedDate.month(), bd.CreatedDate.day()); if(((bd.Billing_Type__c=='FEE')||(bd.Billing_Type__c=='PPU'))&& bd.Billing_Period__c=='monthly'){ i= myDate.monthsBetween(bd.End_of_Billing__c); System.debug('i='+i); } for(Integer n =0; n<i-1; n++){ if(i!=1){ Billing_Detail__c newBd = new Billing_Detail__c (); System.debug('n='+n); newBd.Amount__c=bd.Amount__c; System.debug('amount'+newBd.Amount__c); newBd.Billing_Date__c=bd.Billing_Date__c; newBd.Billing_Type__c=bd.Billing_Type__c; newBd.Billing_Status__c=bd.Billing_Status__c; newBd.Billing_Period__c= bd.Billing_Period__c; newBd.Product_Line__c=bd.Product_Line__c; newBd.Product__c=bd.Product__c; newBd.End_of_Billing__c=bd.End_of_Billing__c; newBd.Opportunity_billing__c=bd.Opportunity_billing__c; newBd.Monthly_Forecast__c= bd.Monthly_Forecast__c.addMonths(n+1); System.debug('monthly forecast'+newBd.Monthly_Forecast__c); listBD.add(newBd); } } insert listBD; } } }the trigger :
trigger bd on Billing_Detail__c (after insert) { for (Billing_Detail__c bd : Trigger.new) { if(trigger.size==1){ Helper.createBD(trigger.new[0]); } } }The ERROR:
"Error: Invalid Data.
Review all error messages below to correct your data.
Apex trigger bd caused an unexpected exception, contact your administrator: bd: execution of AfterInsert caused by: System.DmlException: Insert failed. First exception on row 0; first error: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY, bd: maximum trigger depth exceeded Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert Billing_Detail trigger event AfterInsert: []: Class.Helper.createBD: line 137, column 1"
The error appears only for i=2, in other cases it works well; I don't understand why and how I can resolve.
Can you help me?
Thanks
-
- Frank Carter
- July 05, 2018
- Like
- 0
- Continue reading or reply
how can i delete records for particular contact on click by del hyperlink in action field
VF page
<apex:page controller="contactEditDelete" tabStyle="Account">
<apex:form >
<apex:pageBlock title="Account Detail">
<apex:pageBlockSection >
<apex:outputField value="{!acc.Name}"/>
<apex:outputField value="{!acc.Phone}"/>
<apex:outputField value="{!acc.Fax}"/>
<apex:outputField value="{!acc.AccountNumber}"/>
</apex:pageBlockSection>
<apex:pageBlockSection title="Contact" collapsible="false" columns="1">
<apex:pageBlockTable value="{!acts}" var="conts">
<apex:column headerValue="Action">
<apex:commandLink value="Edit |" action="{!editRedirect}">
<apex:param value="{!conts.Id}" name="selectedContactId" assignTo="{!selectedContact}"/>
</apex:commandLink>
<apex:commandLink value=" Del"/>
</apex:column>
<apex:column value="{!conts.Name}"/>
<apex:column value="{!conts.Title}"/>
<apex:column value="{!conts.Email}"/>
</apex:pageBlockTable>
</apex:pageBlockSection>
</apex:pageBlock>
</apex:form>
</apex:page>
Controller
public class contactEditDelete{
public Account acc {get;set;}
public String selectedContact {get;set;}
public String currentRecordId {get;set;}
public String parameterValue {get;set;}
public contactEditDelete(){
Id id = ApexPages.CurrentPage().getparameters().get('id');
acc = (id==null)?new Account():[select Name,Phone,fax,AccountNumber From Account where Id =:id];
}
public List<Contact> getacts(){
currentRecordId = ApexPages.CurrentPage().getparameters().get('id');
List<Contact> acts = [select id,Name,Title,Email,Phone from Contact where AccountId =: currentRecordId ];
parameterValue = ApexPages.CurrentPage().getparameters().get('nameParam');
return acts;
}
public PageReference editRedirect(){
selectedContact =apexpages.currentPage().getParameters().get('selectedContactId');
PageReference pg=new PageReference('/'+selectedContact+'/e');
return pg;
}
}
<apex:page controller="contactEditDelete" tabStyle="Account">
<apex:form >
<apex:pageBlock title="Account Detail">
<apex:pageBlockSection >
<apex:outputField value="{!acc.Name}"/>
<apex:outputField value="{!acc.Phone}"/>
<apex:outputField value="{!acc.Fax}"/>
<apex:outputField value="{!acc.AccountNumber}"/>
</apex:pageBlockSection>
<apex:pageBlockSection title="Contact" collapsible="false" columns="1">
<apex:pageBlockTable value="{!acts}" var="conts">
<apex:column headerValue="Action">
<apex:commandLink value="Edit |" action="{!editRedirect}">
<apex:param value="{!conts.Id}" name="selectedContactId" assignTo="{!selectedContact}"/>
</apex:commandLink>
<apex:commandLink value=" Del"/>
</apex:column>
<apex:column value="{!conts.Name}"/>
<apex:column value="{!conts.Title}"/>
<apex:column value="{!conts.Email}"/>
</apex:pageBlockTable>
</apex:pageBlockSection>
</apex:pageBlock>
</apex:form>
</apex:page>
Controller
public class contactEditDelete{
public Account acc {get;set;}
public String selectedContact {get;set;}
public String currentRecordId {get;set;}
public String parameterValue {get;set;}
public contactEditDelete(){
Id id = ApexPages.CurrentPage().getparameters().get('id');
acc = (id==null)?new Account():[select Name,Phone,fax,AccountNumber From Account where Id =:id];
}
public List<Contact> getacts(){
currentRecordId = ApexPages.CurrentPage().getparameters().get('id');
List<Contact> acts = [select id,Name,Title,Email,Phone from Contact where AccountId =: currentRecordId ];
parameterValue = ApexPages.CurrentPage().getparameters().get('nameParam');
return acts;
}
public PageReference editRedirect(){
selectedContact =apexpages.currentPage().getParameters().get('selectedContactId');
PageReference pg=new PageReference('/'+selectedContact+'/e');
return pg;
}
}
-
- SalesforceAddict
- July 05, 2018
- Like
- 0
- Continue reading or reply
Error message: Invalid operator on multipicklist field
This is what I want to do:
SELECT Account.Name FROM Account WHERE Business_Type__c like '%Manufacturer/Supplier%'
However I'm getting this error: invalid operator on multipicklist field. Could you tell me what it means and how to fix it?
SELECT Account.Name FROM Account WHERE Business_Type__c like '%Manufacturer/Supplier%'
However I'm getting this error: invalid operator on multipicklist field. Could you tell me what it means and how to fix it?
-
- Support 3743
- July 03, 2018
- Like
- 0
- Continue reading or reply
Probably Limit Exceeded or 0 recipients
Hi Guys, I am getting below error(Probably Limit Exceeded or 0 recipients) from process builder. Please help me to troubeshoot this issue.
ERROR EMAIL :
Error Occurred During Flow "Route_Case_to_relevant_Queue_on_Creation": Probably Limit Exceeded or 0 recipi...
Error element myRule_7_A1 (FlowActionCall).
Probably Limit Exceeded or 0 recipients
This report lists the elements that the flow interview executed. The report is a beta feature.
We welcome your feedback on IdeaExchange.
Flow Details
Flow Name: Route_Case_to_relevant_Queue_on_Creation
Type: Workflow
Version: 11
Status: Active
Flow Interview Details
Interview Label: Route_Case_to_relevant_Queue_on_Creation-11_InterviewLabel
Current User: Rashmi (005O0000004JXSw)
Start time: 07/08/2017 12:25
Duration: 0 seconds
How the Interview Started
Rashmi (005O0000004JXSw) started the flow interview.
Some of this flow's variables were set when the interview started.
myVariable_old = null
myVariable_current = 500O000000AqkLaIAJ
ASSIGNMENT: myVariable_waitStartTimeAssignment
{!myVariable_waitStartTimeVariable} Equals {!Flow.CurrentDateTime}
Result
{!myVariable_waitStartTimeVariable} = "07/08/2017 12:25"
DECISION: myDecision
Executed this outcome: myRule_1
Outcome conditions: and
1. {!myVariable_current.EntitlementId} (550O000000053WQIAY) Is null true
Logic: All conditions must be true (AND)
RECORD UPDATE: myRule_1_A1
Find all Case records where:
Id Equals {!myVariable_current.Id} (500O000000AqkLaIAJ)
Update the records’ field values.
EntitlementId = 550O000000053WQ
Start_First_Response_Milestone__c = true
Result
All records that meet the filter criteria are ready to be updated when the next Screen or Wait element is executed or when the interview finishes.
CASEUPDATEWITHPAC (APEX): myRule_1_A2
Inputs:
caseIds = {!myVariable_current.Id} (500O000000AqkLaIAJ)
Outputs:
None.
DECISION: myDecision2
DECISION: myDecision4
DECISION: myDecision6
Executed this outcome: myRule_7
Outcome conditions: and
1. {!myVariable_current.Origin} (Web) Equals Web
2. {!myVariable_current.Case_Language__c} (English) Equals English
3. {!myVariable_current.ParentId} (null) Is null true
Logic: All conditions must be true (AND)
CASE.ARR_WEB_ENGLISH (EMAIL ALERTS): myRule_7_A1
Inputs:
SObjectRowId = {!myVariable_current.Id} (500O000000AqkLaIAJ)
Error Occurred: Probably Limit Exceeded or 0 recipients
ERROR EMAIL :
Error Occurred During Flow "Route_Case_to_relevant_Queue_on_Creation": Probably Limit Exceeded or 0 recipi...
Error element myRule_7_A1 (FlowActionCall).
Probably Limit Exceeded or 0 recipients
This report lists the elements that the flow interview executed. The report is a beta feature.
We welcome your feedback on IdeaExchange.
Flow Details
Flow Name: Route_Case_to_relevant_Queue_on_Creation
Type: Workflow
Version: 11
Status: Active
Flow Interview Details
Interview Label: Route_Case_to_relevant_Queue_on_Creation-11_InterviewLabel
Current User: Rashmi (005O0000004JXSw)
Start time: 07/08/2017 12:25
Duration: 0 seconds
How the Interview Started
Rashmi (005O0000004JXSw) started the flow interview.
Some of this flow's variables were set when the interview started.
myVariable_old = null
myVariable_current = 500O000000AqkLaIAJ
ASSIGNMENT: myVariable_waitStartTimeAssignment
{!myVariable_waitStartTimeVariable} Equals {!Flow.CurrentDateTime}
Result
{!myVariable_waitStartTimeVariable} = "07/08/2017 12:25"
DECISION: myDecision
Executed this outcome: myRule_1
Outcome conditions: and
1. {!myVariable_current.EntitlementId} (550O000000053WQIAY) Is null true
Logic: All conditions must be true (AND)
RECORD UPDATE: myRule_1_A1
Find all Case records where:
Id Equals {!myVariable_current.Id} (500O000000AqkLaIAJ)
Update the records’ field values.
EntitlementId = 550O000000053WQ
Start_First_Response_Milestone__c = true
Result
All records that meet the filter criteria are ready to be updated when the next Screen or Wait element is executed or when the interview finishes.
CASEUPDATEWITHPAC (APEX): myRule_1_A2
Inputs:
caseIds = {!myVariable_current.Id} (500O000000AqkLaIAJ)
Outputs:
None.
DECISION: myDecision2
DECISION: myDecision4
DECISION: myDecision6
Executed this outcome: myRule_7
Outcome conditions: and
1. {!myVariable_current.Origin} (Web) Equals Web
2. {!myVariable_current.Case_Language__c} (English) Equals English
3. {!myVariable_current.ParentId} (null) Is null true
Logic: All conditions must be true (AND)
CASE.ARR_WEB_ENGLISH (EMAIL ALERTS): myRule_7_A1
Inputs:
SObjectRowId = {!myVariable_current.Id} (500O000000AqkLaIAJ)
Error Occurred: Probably Limit Exceeded or 0 recipients
-
- PawanKumar
- August 07, 2017
- Like
- 0
- Continue reading or reply
How to put field level security(FLS) check for Formula field in Apex?
Hi All,
I have a field level security requirement around custom formula. If the user does not have read access for the custom formula field at the profile level, User should not be abe to see the value at all in the custom Lightning component page / VF page but whenever i do security check in apex code for the field and assign any value to it then it is throwing error saying "formula fields are not writeable".
Please take a snap of my code as below:
List<hed__Course_Offering__c> courseOfferingList = [Select Id,Course_ID__c from hed__Course_Offering__c where hed__Term__c IN :termList ];
for(Integer i=0; i<spProgramDetail.courseOfferingList.size();i++){
// Course_ID__c is a FORMULA Field.
if (!Schema.sObjectType.hed__Course_Offering__c.fields.Course_ID__c.isAccessible()){
courseOfferingList[i].Course_ID__c = '';
}
}
Any help would be appreciated.
Regards,
Pawan Kumar
I have a field level security requirement around custom formula. If the user does not have read access for the custom formula field at the profile level, User should not be abe to see the value at all in the custom Lightning component page / VF page but whenever i do security check in apex code for the field and assign any value to it then it is throwing error saying "formula fields are not writeable".
Please take a snap of my code as below:
List<hed__Course_Offering__c> courseOfferingList = [Select Id,Course_ID__c from hed__Course_Offering__c where hed__Term__c IN :termList ];
for(Integer i=0; i<spProgramDetail.courseOfferingList.size();i++){
// Course_ID__c is a FORMULA Field.
if (!Schema.sObjectType.hed__Course_Offering__c.fields.Course_ID__c.isAccessible()){
courseOfferingList[i].Course_ID__c = '';
}
}
Any help would be appreciated.
Regards,
Pawan Kumar
-
- PawanKumar
- November 29, 2016
- Like
- 0
- Continue reading or reply
How to run trigger in user context to pass App Exchange security review?
Hi All,
Recently, We have been asked to make trigger to run in user context. Please help me if any body has gone thru this kind of secuirty check for trigger. Thanks in advance.
Regards,
Pawan Kumar
Recently, We have been asked to make trigger to run in user context. Please help me if any body has gone thru this kind of secuirty check for trigger. Thanks in advance.
Regards,
Pawan Kumar
-
- PawanKumar
- November 27, 2016
- Like
- 0
- Continue reading or reply
How to fix callout error -- You have uncommitted work pending. Please commit or rollback before calling out?
I'm doing an upload attachment to AWS, but I'm getting this error -- 'You have uncommitted work pending. Please commit or rollback before calling out'
How do I fix this?
@AuraEnabled public static void savePDupcsSettings(ForUploadCustomSettings__c upcsSet, String recId){ ForUploadCustomSettings__c upcs = new ForUploadCustomSettings__c(); upcs = upcsSet; if (upcs.Objects__c != null) { if (recordId != null) { upcs.Name = upcs.Objects__c +'-'+ upcs.Fields__c +'-'+ upcs.Value__c; update upcs; } else { ForUploadCustomSettings__c newVal = new ForUploadCustomSettings__c(); newVal.Name = upcs.Objects__c +'-'+ upcs.Fields__c +'-'+ upcs.Value__c; newVal.Objects__c = upcs.Objects__c; newVal.Fields__c = upcs.Fields__c; newVal.Value__c = upcs.Value__c; insert newVal; } } String stage = 'Closed Won'; ForUploadCustomSettings__c csList = [SELECT Id, Id, Objects__c, Fields__c, Value__c FROM ForUploadCustomSettings__c WHERE Value__c = :stage LIMIT 1]; if (csList != null) { Set<Id> Ids = new Set<Id>(); for (Opportunity opp : [SELECT Id, Name FROM Opportunity WHERE IsClosed = true AND IsWon = true]) { Ids.add(opp.Id); } String formattedDateString = Datetime.now().format('EEE, dd MMM yyyy HH:mm:ss z'); String host = 's3.amazonaws.com/'; String method = 'PUT'; HttpRequest req = new HttpRequest(); Http http = new Http(); List<Attachment> att = [SELECT Id, Name, Body, ContentType FROM Attachment WHERE ParentId IN :Ids]; List<AWScredentialsSettings__c> creds = [SELECT Id, ClientKey__c, SecretKey__c, BucketName__c FROM AWScredentialsSettings__c LIMIT 1]; if (!att.isEmpty() && !creds.isEmpty()) { String bucketname = creds[0].BucketName__c; String key = creds[0].ClientKey__c; String secret = creds[0].SecretKey__c; String attachmentBody = EncodingUtil.base64Encode(att[0].Body); String filename = att[0].Name; req.setMethod(method); req.setEndpoint('https://' + host + bucketname + '/' + filename); // The file should be uploaded to this path in AWS -- Opportunity/Salesforce Id/Secret Files/filename req.setHeader('Content-Length', String.valueOf(attachmentBody.length())); req.setHeader('Content-Encoding', 'UTF-8'); req.setHeader('Content-type', att[0].ContentType); req.setHeader('Connection', 'keep-alive'); req.setHeader('Date', formattedDateString); req.setHeader('ACL', 'public-read'); req.setBody(attachmentBody); String stringToSign = method+'\n\n\n'+ att[0].ContentType + '\n' + formattedDateString +'\n/'+ bucketname +'/' + filename; Blob mac = Crypto.generateMac('HMACSHA1', blob.valueof(stringToSign),blob.valueof(secret)); String signed = EncodingUtil.base64Encode(mac); String authHeader = 'AWS' + ' ' + secret + ':' + signed; req.setHeader('Authorization',authHeader); } HTTPResponse res = http.send(req); System.debug('*Resp:' + String.ValueOF(res.getBody())); System.debug('RESPONSE STRING: ' + res.toString()); System.debug('RESPONSE STATUS: ' + res.getStatus()); System.debug('STATUS_CODE: ' + res.getStatusCode()); } }
- ForceRookie
- July 05, 2019
- Like
- 0
- Continue reading or reply
How to write a test class for Update Trigger on account
trigger UpdateType on Account (before insert,before update,after insert) { if(trigger.isInsert && trigger.isBefore) { for(Account a:trigger.new) { if(a.industry=='education') { a.addError('we dont deal with education industry'); } } } if(trigger.isUpdate) { for(Account a:trigger.new) { if(a.Type=='Prospect') { a.Type='Other'; } } } }
@isTest public class UpdateTypeTest { static testmethod void accUpdate() { Account acc= new Account( Name='Example', Industry='Education'); insert acc; acc=[select name, industry, type from account where type=:acc.Type]; System.assertEquals('Other', acc.Type); update acc; } }
Test class for the Trigger
Here the trigger fires whenever we give the type on account as prospect it would update it to 'Other'
My test class is covering only 60% of the code , somehow it is not covering the updated part. Can someone help me out?
- hanifa fatima
- July 20, 2018
- Like
- 0
- Continue reading or reply
what is the best practice to invoke rest webservice after a batch job gets completed successfully?
My requirement is once all the records are updated using Batch, all the updated records should get synced with the external System using REST services.
- Rishiraj Singh 19
- July 20, 2018
- Like
- 0
- Continue reading or reply
How can rollback database in apex?
I process insert to some tables:
I want rollback all, if occur error/
How can rollback database in apex?
public PageReference checkoutPaypal() { try { List<AccountUser__c> listacc = [SELECT id,AccountId__c FROM AccountUser__c WHERE email__c=:email LIMIT 1]; AccountUser__c a=new AccountUser__c(); Account acc=new Account(); if(listacc.size()>0) { a=listacc.get(0); } else { acc.Name=name; insert acc; //save value ok // a.Name=name; a.AccountId__c=acc.Id; a.Email__c=email; a.Status__c=0; a.Password__c='0'; insert a; } Contact con=new Contact(); con.LastName=name; con.Email=email; //con.Phone__c=phonenumber; con.AccountId=a.AccountId__c; insert con; // Contract hd=new Contract(); hd.AccountId=a.AccountId__c; hd.StartDate=system.today(); hd.ContractTerm=1; insert hd; Order od=new Order(); od.AccountId=a.AccountId__c; od.ContractId=hd.Id; od.EffectiveDate=system.today(); insert od; Double itotalMoney=0; if(ids !='') { string searchquery='select ProductCode,Name,ImageName__c,(Select UnitPrice,Pricebook2.Name From PricebookEntries where IsActive=True Order By UnitPrice ASC ) from Product2 where ProductCode in ('+SOQL_IDs+')'; List<Product2> MyProducts= Database.query(searchquery); List<OrderItem> lsDetail=new List<OrderItem>(); for(Integer i=0;i<MyProducts.size();i++) { String code=MyProducts.get(i).ProductCode; String sl=listCarts.get(code); OrderItem ode=new OrderItem(); ode.OrderId=od.Id; ode.Quantity=Double.valueOf(sl); ode.UnitPrice=MyProducts.get(i).PricebookEntries.get(0).UnitPrice; ode.Product2Id=MyProducts.get(i).Id; lsDetail.Add(ode); itotalMoney=itotalMoney+ ode.Quantity * ode.UnitPrice; } insert lsDetail; } Pagereference redirectedPage = New PageReference('/apex/payment?amount='+itotalMoney); return redirectedPage; } catch(DmlException e) { err=e.getMessage(); } return null; }Current if function occur error, some process still insert data ok.
I want rollback all, if occur error/
How can rollback database in apex?
- dai tran 6
- July 20, 2018
- Like
- 0
- Continue reading or reply
SBQQ__ContractService: SBQQ,QuoteAfter: ... System.CalloutException: Callout from triggers are currently not supported.
Hello!
I am developing automation to create SBQQ quotes and opportunities in order to eventually create contracts, etc... One step of the automation tries to contract the opportunity. When calling, I notice that I am getting the following error. The contract is produced but I am not getting a renewal opportunity created. Any help would be greatly appreciated.
Insert failed. First exception on row 0; first error: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY, SBQQ.QuoteAfter: execution of AfterUpdate
caused by: System.CalloutException: Callout from triggers are currently not supported.
(SBQQ)
We see this error in the Apex Jobs status detail for the apex class SBQQ ContractService. Does anyone know what is failing and why?
Update, if we reduce the batch size to 1, this error doesn't occur.
I am developing automation to create SBQQ quotes and opportunities in order to eventually create contracts, etc... One step of the automation tries to contract the opportunity. When calling, I notice that I am getting the following error. The contract is produced but I am not getting a renewal opportunity created. Any help would be greatly appreciated.
Insert failed. First exception on row 0; first error: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY, SBQQ.QuoteAfter: execution of AfterUpdate
caused by: System.CalloutException: Callout from triggers are currently not supported.
(SBQQ)
We see this error in the Apex Jobs status detail for the apex class SBQQ ContractService. Does anyone know what is failing and why?
Update, if we reduce the batch size to 1, this error doesn't occur.
- Dave Berry 9
- July 19, 2018
- Like
- 0
- Continue reading or reply
How to update field after sending Email notification
Hello All,
I have a trigger which is after trigger and it is sending an email to the contact, my requiremnt is once I sent email I want to update one of field of my custom object. Can someone help with sample code.
Thanks.
I have a trigger which is after trigger and it is sending an email to the contact, my requiremnt is once I sent email I want to update one of field of my custom object. Can someone help with sample code.
Thanks.
- DJ 367
- July 19, 2018
- Like
- 0
- Continue reading or reply
Error: Compile Error: Method does not exist or incorrect signature: void runEligibilityCheck() from the type Eligibility at line 4 column 15
What i'm trying to achive:
Simply call a class from a trigger when checkbox is checked and lead record is saved.
Error message:
Error: Compile Error: Method does not exist or incorrect signature: void runEligibilityCheck() from the type Eligibility at line 4 column 15
Simply call a class from a trigger when checkbox is checked and lead record is saved.
Error message:
Error: Compile Error: Method does not exist or incorrect signature: void runEligibilityCheck() from the type Eligibility at line 4 column 15
// My Class global with sharing class Eligibility { // Code here private static String runEligibilityCheck(String patientID) { // More code in my method } }
// My Trigger. Trigger CheckEligiblityUponSelfSchedule on Lead (before update, before insert) { for (Lead l: Trigger.new) { if(l.self_scheduled__c = TRUE){ Eligibility.runEligibilityCheck(); } } }
- Mahmoud Coudsi 1
- July 19, 2018
- Like
- 0
- Continue reading or reply
select from where structure
I'm sure that this is something very simple that I'm missing in my SOQL structure. My current code is:
public class NewActionListController {
public List<ACT_Action__c> getnewActions() {
List<ACT_Action__c> results = Database.query(
'SELECT ID, Name FROM ACT_Action__c'
'WHERE username=:UserInfo.getUserName()');
return results;
}
}
I am getting an error that says the following:
Extra ')', at 'WHERE username=:UserInfo.getUserName()'
Can someone help? Thanks!
public class NewActionListController {
public List<ACT_Action__c> getnewActions() {
List<ACT_Action__c> results = Database.query(
'SELECT ID, Name FROM ACT_Action__c'
'WHERE username=:UserInfo.getUserName()');
return results;
}
}
I am getting an error that says the following:
Extra ')', at 'WHERE username=:UserInfo.getUserName()'
Can someone help? Thanks!
- Diane Roberts 3
- July 19, 2018
- Like
- 0
- Continue reading or reply
unable to get the code to work but no errors
I have no errors in the code, but the button is not working. The idea is that it is supposed to send an email to the contact email of X3_Party_Installer and then create an activity as well. Yet, when I press the button nothing happens. Is there something wrong with the code?
Controller:
VF Page:
Controller:
public class SendSiteSurveyEmailController { public String Site_Survey {get;set;} Public SendSiteSurveyEmailController(ApexPages.StandardController controller) { Site_Survey = ApexPages.currentPage().getParameters().get('Id'); } Public Pagereference SendSiteSurveyFunction() { list<Site_Survey__c> sitelist = [SELECT X3rd_Party_Installer__c, X3rd_Party_Installer__r.email FROM Site_Survey__c LIMIT 1]; String toaddress= sitelist[0].X3rd_Party_Installer__r.email; try{ Messaging.SingleEmailMessage mail = new Messaging.SingleEmailMessage(); String[] addr = new String[] {toaddress}; mail.setToAddresses(addr); mail.setReplyTo(toaddress); mail.setSenderDisplayName('Name'); mail.setSubject('DarPro Site Survey'); mail.setBccSender(false); mail.setUseSignature(true); mail.setHtmlBody('<b> BODY </b>'); List<Messaging.EmailFileAttachment> fileAttachments = new List<Messaging.Emailfileattachment>(); for (Attachment a : [SELECT Name, Body, BodyLength FROM Attachment WHERE ParentId = :Site_Survey Order By LastModifiedDate DESC Limit 1]){ Messaging.Emailfileattachment efa = new Messaging.Emailfileattachment(); efa.setFileName(a.Name); efa.setBody(a.Body); fileAttachments.add(efa); //mail.setFileAttachments(new Messaging.SingleEmailMessage[] {efa}); } mail.setFileAttachments(fileAttachments); Messaging.sendEmail(new Messaging.SingleEmailMessage[] { mail }); Contact ct = [SELECT id FROM Contact WHERE Email = :toaddress LIMIT 1]; Task newTask = new Task(Description = 'Site Survey Email', Priority = 'Normal', Status = 'Completed', Subject = 'Site Survey', Type = 'Email', WhoId = ct.Id); } catch(Exception e) {} PageReference reference = new PageReference('https://darlingingredients--griffsdbx.cs11.my.salesforce.com/'+Site_Survey); reference.setRedirect(true); return reference; } }
VF Page:
<apex:page standardController="Site_Survey__c" extensions="SendSiteSurveyEmailController"> <apex:form > <script type="text/javascript"> function init() { sendEmail(); } if(window.addEventListener) window.addEventListener('load',init,true) else window.attachEvent('onload',init) </script> <apex:actionFunction name="sendEmail" action="{!sendSiteSurveyFunction}"> </apex:actionFunction> </apex:form> </apex:page>
- Kathryn Bullock
- July 19, 2018
- Like
- 0
- Continue reading or reply
Dynamic Visualforce page to add multiple contact with checkbox .
i want to create a button add contact when i clicked one more empty contact show in my page.
like this screen shot.
like this screen shot.
- Shriwastab Singh
- July 19, 2018
- Like
- 0
- Continue reading or reply
Poat method sending request twice while updatinng a record
Hi All,
I created post method in my controller class but i can see that post method is send request twice to the middlewre while updating record,but while creating a record it is sending request only onece ,hereis my sample post class ,can someone please suggest me how i can improve my method.
I created post method in my controller class but i can see that post method is send request twice to the middlewre while updating record,but while creating a record it is sending request only onece ,hereis my sample post class ,can someone please suggest me how i can improve my method.
public static HttpResponse postData(String url, String json) { HttpResponse response; HttpRequest req = new HttpRequest(); req.setClientCertificateName('HttpClientCert'); req.setMethod('POST'); req.setEndpoint(url); req.setHeader('Content-Type', 'text/plain; charset=utf-8'); req.setTimeout(30000); //req.setHeader('Content-Type', 'application/json; charset=utf-8'); req.setHeader('SOAPAction', url); req.setBody(json); Http httpSender = new Http(); String responseString = ''; try { if (!Test.isRunningTest()){ response = httpSender.send(req); }else{ response = new HttpResponse(); } } catch(System.CalloutException e) { system.debug('***********'+e); } return response; }
- divya1234
- July 19, 2018
- Like
- 0
- Continue reading or reply
hello all , i have a question regarding the vf page
i want to create contact records using checkbox in vf page
Apex Controller:
public class AddmultipleContactsVF {
Contact Cont = new Contact();
public list<Contact> listContact{get;set;}
public Boolean IsSelected{set;get;}
public AddmultipleContactsVF()
{
listContact=new list<Contact>();
listContact.add(Cont);
}
Public void addContact()
{
Contact con = new Contact();
listContact.add(con);
}
public PageReference saveContact() {
for(Integer i=1; i<listContact.size(); i++)
{
if(Isselected==true){
upsert listContact;
}
}
return null;
}
public class wrapperContact{
public Boolean IsSelected{set;get;}
public wrapperContact(){
IsSelected=false;
}
}
}
VF Page:
<apex:page Controller="AddmultipleContactsVF">
<apex:form >
<apex:pageBlock >
<apex:pageBlockTable value="{!listContact}" var="con">
<apex:column headerValue="Contact LastName">
<apex:inputCheckbox value="{!IsSelected}" id="InputId"/>
<apex:inputField value="{!con.LastName}"/>
<apex:CommandButton value="+" action="{!addContact}"/>
</apex:column>
</apex:pageBlockTable>
<apex:pageBlockButtons >
<apex:CommandButton value="Save Contact" action="{!saveContact}"/>
</apex:pageBlockButtons>
</apex:pageBlock>
</apex:form>
</apex:page>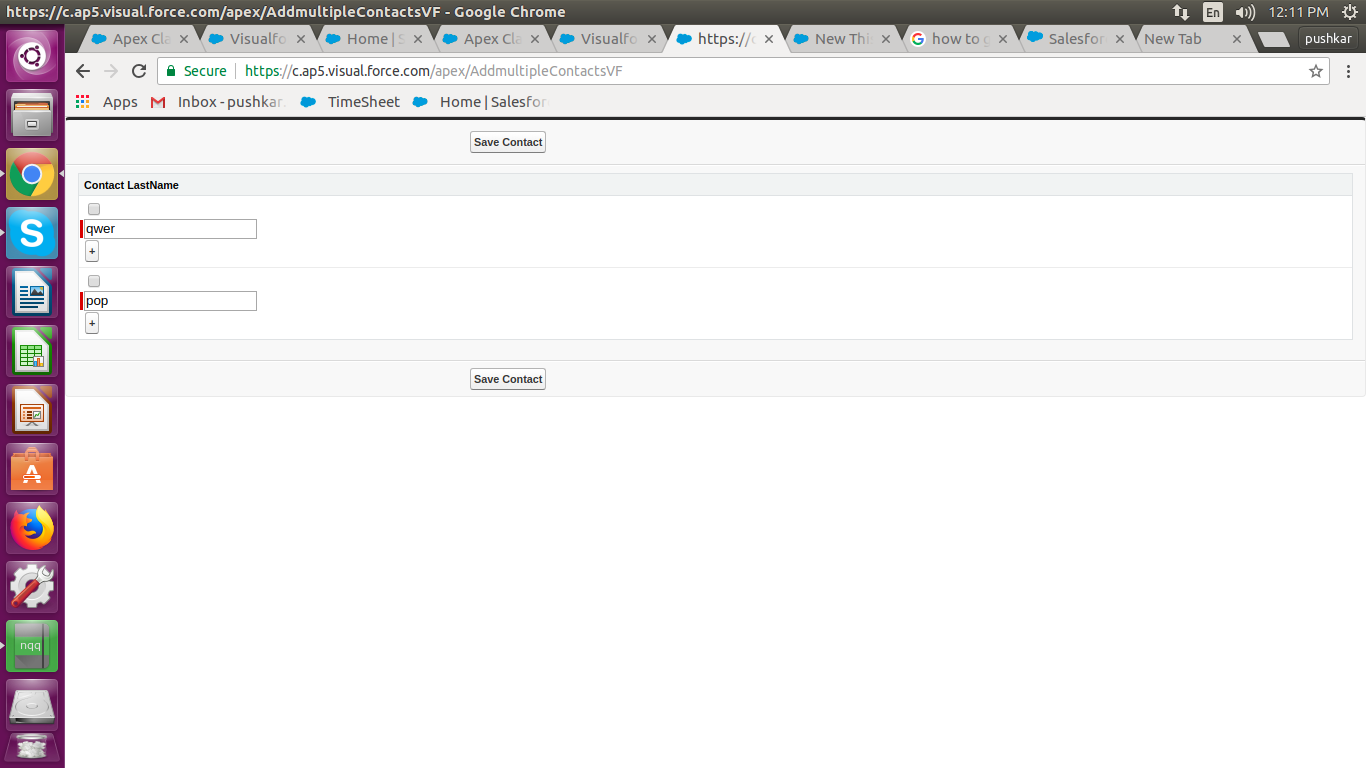
Apex Controller:
public class AddmultipleContactsVF {
Contact Cont = new Contact();
public list<Contact> listContact{get;set;}
public Boolean IsSelected{set;get;}
public AddmultipleContactsVF()
{
listContact=new list<Contact>();
listContact.add(Cont);
}
Public void addContact()
{
Contact con = new Contact();
listContact.add(con);
}
public PageReference saveContact() {
for(Integer i=1; i<listContact.size(); i++)
{
if(Isselected==true){
upsert listContact;
}
}
return null;
}
public class wrapperContact{
public Boolean IsSelected{set;get;}
public wrapperContact(){
IsSelected=false;
}
}
}
VF Page:
<apex:page Controller="AddmultipleContactsVF">
<apex:form >
<apex:pageBlock >
<apex:pageBlockTable value="{!listContact}" var="con">
<apex:column headerValue="Contact LastName">
<apex:inputCheckbox value="{!IsSelected}" id="InputId"/>
<apex:inputField value="{!con.LastName}"/>
<apex:CommandButton value="+" action="{!addContact}"/>
</apex:column>
</apex:pageBlockTable>
<apex:pageBlockButtons >
<apex:CommandButton value="Save Contact" action="{!saveContact}"/>
</apex:pageBlockButtons>
</apex:pageBlock>
</apex:form>
</apex:page>
- Pushkar Gupta
- July 19, 2018
- Like
- 0
- Continue reading or reply
Is it possible to register a salesforce developer account with a group email?
I plan to register a salesforce developer account with a group email, is it possible? Thanks for your help
- compqa talend
- July 19, 2018
- Like
- 0
- Continue reading or reply
application event concept
My understanding of application event is if two components not related to each then we can use applicaiton event to pass data.
Please correct me if wrong.
I have done a code but I am unable to pass data.
I checked using debugger but value is not comoing into the child attribute from the parent.
Please let me know where I go wrong.
thanks
sheila S
Please correct me if wrong.
I have done a code but I am unable to pass data.
ApplicationEvent.vt <aura:event type="APPLICATION" description="Event template"> <aura:attribute name="message" type="string" access="public"/> </aura:event> ParentComponent.cmp <aura:component implements="flexipage:availableForAllPageTypes" access="global" > <aura:attribute name="someattr" type="string" default="I am GOD" access="private"/> <aura:registerEvent name="handleApplicationEvent" type="c.ApplicationEvent"/> <lightning:button name="clickMe" label="ClickMe" onclick="{!c.HandleApplicationEvent}"/> </aura:component> PrentComponentController.js ({ HandleApplicationEvent : function(component, event, helper) { debugger; var applnEvent=$A.get("e.c:ApplicationEvent"); var val=component.get("v.someattr"); console.log(val); applnEvent.setParams({"message" : val}); applnEvent.fire(); } }) ChildComponent.cmp <aura:component > <aura:attribute name="receivedvaluefromparent" type="string" access="private"/> <aura:handler event="c:ApplicationEvent" action="{!c.HandleApplicationEvent}"/> <c:ParentComponent/> Inside the child component : {!v.receivedvaluefromparent} </aura:component> ChildComponent.js ({ HandelApplicationEvent : function(component, event, helper) { debugger; var evtMessage=event.getParam("message"); console.log(evtMessage); component.set("v.receivedvaluefromparent",evtMessage.ToString()); } }) MainApp.app <aura:application > <c:ParentComponent/> </aura:application>
I checked using debugger but value is not comoing into the child attribute from the parent.
Please let me know where I go wrong.
thanks
sheila S
- sheila srivatsav
- July 19, 2018
- Like
- 0
- Continue reading or reply
SOQL 101 issue due to query in for loop. Need help
I have the following trigger which is poorly written and causing during data load and record update for certain records. I am trying to fix the query issue at list for now. But I am not expert like you guys so need help.
Following Code trying to update but getting- Unexpected token issue.
Original Code:
Following Code trying to update but getting- Unexpected token issue.
List <Investor_Position__c> listIvPost = [Select Id,Investor_Position_Account__c,Is_Active__c, Current_Commitments_Functional__c, Source_of_Capital__c, Investment_Vehicle__r.Id, Investment_Vehicle__r.Number_of_Current_Investors__c, Investment_Vehicle__r.Number_of_Historical_Investors__c from Investor_Position__c where Investment_Vehicle__r.Id IN InvestmentVehicleIds]; for(Id id : InvestmentVehicleIds) { for( Investor_Position__c obj :listIvPost ) { if(obj.Is_Active__c == TRUE && obj.Source_of_Capital__c != null) {
Original Code:
trigger InvestorPositionTrigger on Investor_Position__c (after insert, after update, after delete) { Set<Id> numofhistinvestors = new Set<Id>(); Set<Id> numofcurrentinvestors = new Set<Id>(); Set<Id> InvestmentVehicleIds = new Set<Id>(); List<Investment_Vehicle__c> lstUpdateInvVehicle = new List<Investment_Vehicle__c>(); if(trigger.isafter) { if(trigger.isinsert || trigger.isupdate) { for(Investor_Position__c inv : Trigger.New) { InvestmentVehicleIds.add(inv.Investment_Vehicle__c); } } if(trigger.isdelete) { for(Investor_Position__c inv : Trigger.old) { InvestmentVehicleIds.add(inv.Investment_Vehicle__c); } } for(Id id : InvestmentVehicleIds) { for( Investor_Position__c obj : [Select Id,Investor_Position_Account__c,Is_Active__c, Current_Commitments_Functional__c, Source_of_Capital__c, Investment_Vehicle__r.Id, Investment_Vehicle__r.Number_of_Current_Investors__c, Investment_Vehicle__r.Number_of_Historical_Investors__c from Investor_Position__c where Investment_Vehicle__r.Id =:id]) { if(obj.Is_Active__c == TRUE && obj.Source_of_Capital__c != null) { if(obj.Current_Commitments_Functional__c > 0 && (obj.Source_of_Capital__c.contains('Limited Partners') || obj.Source_of_Capital__c.contains('Operating Partner'))) { numofcurrentinvestors.add(obj.Investor_Position_Account__c); } if(obj.Source_of_Capital__c.contains('Limited Partners') || obj.Source_of_Capital__c.contains('Operating Partner')) { numofhistinvestors.add(obj.Investor_Position_Account__c); } } } Investment_Vehicle__c invveh = new Investment_Vehicle__c(); invveh.Id = id; invveh.Number_of_Historical_Investors__c = numofhistinvestors.size(); invveh.Number_of_Current_Investors__c = numofcurrentinvestors.size(); lstUpdateInvVehicle.add(invveh); numofcurrentinvestors.clear(); numofhistinvestors.clear(); } try { if(lstUpdateInvVehicle.size() > 0) { update lstUpdateInvVehicle; } } catch(exception ex) { for (Investor_Position__c obj : trigger.new) { obj.addError(ex.getmessage()); } } } if(Trigger.isInsert){ ConfigurableRollup.rollup(trigger.new); } if(Trigger.isUpdate){ system.debug('when is update------'); ConfigurableRollup.rollup(trigger.new, Trigger.OldMap); } if(Trigger.isDelete){ ConfigurableRollup.rollup(trigger.old); } }
- Sunny Solanki 11
- July 17, 2018
- Like
- 0
- Continue reading or reply
I have a problem with Pagination, it's working properly on standard object but not custom object.... why?????
<apex:page standardController="sale__c" recordSetVar="sales" sidebar="false">
<apex:form >
<apex:pageBlock >
<apex:pageMessages />
<apex:pageBlockButtons >
<apex:commandButton value="Save" action="{!save}"/>
<apex:commandButton value="Return" action="{!cancel}"/>
</apex:pageBlockButtons>
<apex:pageBlockTable value="{!sales}" var="s" id="table" rows="10">
<apex:column headerValue="Account Name">
<apex:outputField value="{!s.Name}"/>
</apex:column>
<apex:column headerValue="External Id">
<apex:inputField value="{!s.External_Id__c}"/>
</apex:column>
<apex:column headerValue="DOJ">
<apex:inputField value="{!s.DOJ__c}"/>
</apex:column>
<apex:column headerValue="Select">
<apex:inputField value="{!s.Select_Intrested_Field__c}"/>
</apex:column>
<apex:inlineEditSupport />
</apex:pageBlockTable>
<apex:commandLink action="{!Previous}" value="Previous Page" rendered="{!HasPrevious}"/>
<apex:commandLink action="{!Next}" value="Next Page" rendered="{!HasNext}"/>
<apex:commandLink action="{!Last}" value="Last Page" rendered="{!HasNext}"/>
<apex:commandLink action="{!First}" value="First Page" rendered="{!HasPrevious}"/>
</apex:pageBlock>
</apex:form>
</apex:page>
pagination links are not visible in apex page....
what is the mistake here....
Thanks in Advance
<apex:form >
<apex:pageBlock >
<apex:pageMessages />
<apex:pageBlockButtons >
<apex:commandButton value="Save" action="{!save}"/>
<apex:commandButton value="Return" action="{!cancel}"/>
</apex:pageBlockButtons>
<apex:pageBlockTable value="{!sales}" var="s" id="table" rows="10">
<apex:column headerValue="Account Name">
<apex:outputField value="{!s.Name}"/>
</apex:column>
<apex:column headerValue="External Id">
<apex:inputField value="{!s.External_Id__c}"/>
</apex:column>
<apex:column headerValue="DOJ">
<apex:inputField value="{!s.DOJ__c}"/>
</apex:column>
<apex:column headerValue="Select">
<apex:inputField value="{!s.Select_Intrested_Field__c}"/>
</apex:column>
<apex:inlineEditSupport />
</apex:pageBlockTable>
<apex:commandLink action="{!Previous}" value="Previous Page" rendered="{!HasPrevious}"/>
<apex:commandLink action="{!Next}" value="Next Page" rendered="{!HasNext}"/>
<apex:commandLink action="{!Last}" value="Last Page" rendered="{!HasNext}"/>
<apex:commandLink action="{!First}" value="First Page" rendered="{!HasPrevious}"/>
</apex:pageBlock>
</apex:form>
</apex:page>
pagination links are not visible in apex page....
what is the mistake here....
Thanks in Advance
- sai tarun
- August 18, 2017
- Like
- 1
- Continue reading or reply
Hello guys i have to write Trigger on account insertion and updation
Using Trigger
· On Account insertion and updation, get a Map (Account Id as Key and Account record as Value) with no duplicates.
· Just show Map values in Debug
· On Account insertion and updation, get a Map (Account Id as Key and Account record as Value) with no duplicates.
· Just show Map values in Debug
- Priyank Sahu
- August 17, 2017
- Like
- 1
- Continue reading or reply