-
ChatterFeed
-
0Best Answers
-
0Likes Received
-
0Likes Given
-
27Questions
-
47Replies
Kanban Settings - No Owner Field
I have a custom object "Milestone Task" that is part of a managed package. Because it has a master-detail relationship, no owner field exists on the object. Instead we have a custom user lookup field called "Assigned". I understand that the only options for Kanban settings are to group by a picklist field or an owner field. What are my options if the owner field does not exist? Do I have to code my own custom page to view the records by the custom User lookup field?
-
- Jean Grey 10
- November 06, 2018
- Like
- 0
How to shorten processing time on custom search page
I have a page with a class behind that searches the database for all related leads, contacts, accounts, opportunities, and tasks. The search term is the domain, which I match to the email address or the website. We have millions of records and the page works but it takes a really long time to load. What can I do to speed this up? Should I try paginating my queries? Any other ideas?
public class domainSearchPage { ApexPages.StandardSetController setCont; public domainSearchPage(ApexPages.StandardSetController controllerD) { setCont = controllerD; } public String domain {get;set;} transient List<Lead> leadList {get;set;} transient Set<Id> leadSet {get;set;} public Integer leadSize {get;set;} transient List<Lead> matchedLeads {get;set;} public List<Lead> leadList1K {get;set;} public List<Account> accList1K {get;set;} public List<Contact> conList1K {get;set;} public List<Task> existingTasks1K {get;set;} public List<Task> openTasks1K {get;set;} transient List<Account> accList {get;set;} transient Set<Id> accSet {get;set;} public Integer accSize {get;set;} transient List<Contact> conList {get;set;} transient Set<Id> conSet {get;set;} public Integer conSize {get;set;} transient List<Opportunity> oppList {get;set;} transient Set<Id> oppSet {get;set;} public Integer oppSize {get;set;} transient List<Task> taskList {get;set;} transient Set<Id> taskSet {get;set;} transient Set<Id> existingTaskSet {get;set;} transient Set<Id> openTaskSet {get;set;} public Integer openTaskSize {get;set;} public List<Task> openTasks {get;set;} public List<Task> existingTasks {get;set;} public Integer existingTaskSize {get;set;} transient Set<Id> whoSet {get;set;} transient Set<Id> whatSet {get;set;} transient Set<Id> repSet {get;set;} public Integer repSize {get;set;} public List<Lead> leadShortList {get;set;} public List<Contact> conShortList {get;set;} public List<Task> openTaskShortList {get;set;} public List<Task> existingTaskShortList {get;set;} public List<Account> accShortList {get;set;} public List<Opportunity> oppShortList {get;set;} public List<Opportunity> oppListTest {get;set;} public List<OpportunityContactRole> ocrList {get;set;} public void search(){ if(Test.isRunningTest()){domain='test';} leadSet= new Set<Id>(); conSet = new Set<Id>(); accSet = new Set<Id>(); oppSet = new Set<Id>(); taskSet = new Set<Id>(); whoSet = new Set<Id>(); whatSet = new Set<Id>(); existingTaskSet = new Set<Id>(); openTaskSet = new Set<Id>(); repSet = new Set<Id>(); leadList = new List<Lead>(); matchedLeads = new List<Lead>(); conList = new List<Contact>(); oppList = new List<Opportunity>(); ocrList = new List<OpportunityContactRole>(); oppShortList= new List<Opportunity>(); conShortList = new List<Contact>(); accShortList = new List<Account>(); leadShortList = new List<Lead>(); existingTaskShortList = new List<Task>(); openTaskShortList = new List<Task>(); existingTasks= new List<Task>(); openTasks= new List<Task>(); leadList = new List<Lead>(); conList=new List<Contact>(); existingTasks=new List<Task>(); openTasks=new List<Task>(); accList=new List<Account>(); oppList=new List<Opportunity>(); oppShortList=new List<Opportunity>(); //get list of users for task search later List<User> repList = new List<User>([SELECT Id FROM User WHERE Forecast_User__c=TRUE]); List<User> notSales = new List<User>([SELECT Id FROM User WHERE Forecast_User__c=FALSE]); Set<Id> notSalesSet = new Set<Id>(); for(User u :notSales){ notSalesSet.add(u.Id); } Set<Id> repSet = new Set<Id>(); Set<Id> salesReps = new Set<Id>(); for(User u :repList){ salesReps.add(u.Id); } String leadQuery = 'SELECT Id,Name,Domain__c,Part_of_Prospecting_List__c,Territory_ID__c,Company_Size__c,Matched_Account__c,Matched_Account__r.Name,Matched_Account__r.OwnerId,Website,OwnerId,Owner.Name,Owner.UserRole.Name,Company FROM Lead where IsConverted=FALSE AND (Part_of_Prospecting_List__c=TRUE OR Synced_with_HubSpot__c=TRUE) AND Domain__c LIKE \''+domain+'%\''; leadList = Database.query(leadQuery); system.debug('leadList '+leadList.size()); for(Lead l :leadList){ leadSet.add(l.Id); whoSet.add(l.Id); repSet.add(l.OwnerId); } String leadsWebsite = 'SELECT Id,Name,Domain__c,Part_of_Prospecting_List__c,Territory_ID__c,Company_Size__c,Matched_Account__c,Matched_Account__r.Name,Matched_Account__r.OwnerId,Website,OwnerId,Owner.Name,Owner.UserRole.Name,Company FROM Lead where IsConverted=FALSE AND (Part_of_Prospecting_List__c=TRUE OR Synced_with_HubSpot__c=TRUE) AND Website LIKE \''+domain+'%\''; List<Lead> leadListWebsite = new List<Lead>(); leadListWebsite = Database.query(leadsWebsite); system.debug('leadListWebsite '+leadListWebsite.size()); for(Lead l :leadListWebsite){ leadSet.add(l.Id); whoSet.add(l.Id); repSet.add(l.OwnerId); } system.debug('repSet '+repSet); String leadsLMdomain = 'SELECT Id,Name,Domain__c,Part_of_Prospecting_List__c,Territory_ID__c,Company_Size__c,Matched_Account__c,Matched_Account__r.Name,Matched_Account__r.OwnerId,Website,OwnerId,Owner.Name,Owner.UserRole.Name,Company FROM Lead where IsConverted=FALSE AND (Part_of_Prospecting_List__c=TRUE OR Synced_with_HubSpot__c=TRUE) AND Matched_Account__r.Domain_for_lead_conversion__c LIKE \''+domain+'%\''; List<Lead> leadListLMdomain = new List<Lead>(); leadListLMdomain = Database.query(leadsLMdomain); system.debug('leadListLMdomain '+leadListLMdomain.size()); for(Lead l :leadListLMdomain){ leadSet.add(l.Id); whoSet.add(l.Id); repSet.add(l.OwnerId); } String leadsLMwebsite = 'SELECT Id,Name,Domain__c,Part_of_Prospecting_List__c,Territory_ID__c,Company_Size__c,Matched_Account__c,Matched_Account__r.Name,Matched_Account__r.OwnerId,Website,OwnerId,Owner.Name,Owner.UserRole.Name,Company FROM Lead where IsConverted=FALSE AND (Part_of_Prospecting_List__c=TRUE OR Synced_with_HubSpot__c=TRUE) AND Matched_Account__r.Website LIKE \''+domain+'%\''; List<Lead> leadListLMwebsite = new List<Lead>(); leadListWebsite = Database.query(leadsLMwebsite); system.debug('leadListLMWebsite '+leadListLMWebsite.size()); for(Lead l :leadListLMwebsite){ leadSet.add(l.Id); whoSet.add(l.Id); repSet.add(l.OwnerId); } system.debug('repSet '+repSet); List<Lead> getRelated = new List<Lead>([SELECT Id, Matched_Account__c,Matched_Account__r.Name,Matched_Account__r.OwnerId FROM Lead WHERE Id IN :leadSet]); for(Lead l :getRelated){ repSet.add(l.Matched_Account__r.OwnerId); accSet.add(l.Matched_Account__c); } leadSize=leadSet.size(); String accQuery = 'SELECT Id,OwnerId,Name,Owner.UserRole.Name,Owner.Name,Territory_ID__c,Company_Size__c,Website,Domain_for_lead_conversion__c FROM Account WHERE Domain_for_lead_conversion__c LIKE \''+domain+'%\''; accList = Database.query(accQuery); for(Account a :accList){ accSet.add(a.Id); repSet.add(a.OwnerId); } String accWebsite = 'SELECT Id,OwnerId,Name,Owner.UserRole.Name,Owner.Name,Territory_ID__c,Company_Size__c,Website,Domain_for_lead_conversion__c FROM Account WHERE Website_Domain__c LIKE \''+domain+'%\''; List<Account> accWebsiteList = Database.query(accWebsite); for(Account a :accWebsiteList){ accSet.add(a.Id); repSet.add(a.OwnerId); } accSize=accSet.size(); String conQuery = 'SELECT Id,AccountId,OwnerId,Account.Name,Name,Owner.Name,Owner.UserRole.Name,Domain__c FROM Contact WHERE Domain__c LIKE \'%'+domain+'%\''; conList = Database.query(conQuery); for(Contact c :conList){ conSet.add(c.Id); whoSet.add(c.Id); } ocrList = new List<OpportunityContactRole>([SELECT Id,ContactId,Contact.OwnerId,Opportunity.OwnerId,OpportunityId FROM OpportunityContactRole WHERE ContactId IN :accSet]); for(OpportunityContactRole ocr :ocrList){ conSet.add(ocr.ContactId); whoSet.add(ocr.ContactId); whatSet.add(ocr.OpportunityId); repSet.add(ocr.Opportunity.OwnerId); } conSize=conSet.size(); oppList = new List<Opportunity>([SELECT Id,Name,Account.Name,AccountId,Amount,CloseDate,Territory_ID__c,Company_Size__c,OwnerId,Owner.Name,Owner.UserRole.Name,Type FROM Opportunity WHERE CloseDate>=TODAY AND IsClosed=FALSE AND AccountId IN :accSet]); for(Opportunity o :oppList){ oppSet.add(o.Id); whatSet.add(o.Id); repSet.add(o.OwnerId); } //find related tasks openTasks = new List<Task>([SELECT Id,OwnerId,Owner.Name,Owner.UserRole.Name,ActivityDate,Type,Subject,Who.Name,What.Name,WhoId,WhatId FROM Task WHERE OwnerId IN :repSet AND (WhoId IN :whoSet OR WhatId IN :whatSet) AND ActivityDate>=TODAY AND Status !='Completed' AND (NOT Subject LIKE '%Automated%') AND (Type LIKE 'Call%' OR Type LIKE 'Email%' OR Type = 'Follow up') ORDER BY ActivityDate LIMIT 50]); for(Task t :openTasks){ repSet.add(t.OwnerId); openTaskSet.add(t.Id); } existingTasks = new List<Task>([SELECT Id,OwnerId,Owner.Name,Owner.UserRole.Name,ActivityDate,Type,Subject,Who.Name,What.Name,WhoId,WhatId FROM Task WHERE OwnerId IN :repSet AND (WhoId IN :whoSet OR WhatId IN :whatSet) AND ActivityDate<=TODAY AND ActivityDate>=LAST_N_DAYS:120 AND Status ='Completed' AND (Type LIKE 'Call%' OR Type LIKE 'Email%' OR Type = 'Follow up') ORDER BY ActivityDate LIMIT 50]); if(existingTasks.size()!=0){ for(Task t :existingTasks){ repSet.add(t.OwnerId); existingTaskSet.add(t.Id); } } accSet.remove(null); repSet.remove(null); repSet.removeAll(notSalesSet); repSize=repSet.size(); oppSize=oppSet.size(); accSize=accSet.size(); //short lists conShortList = new List<Contact>([SELECT Id,AccountId,OwnerId,Account.Name,Name,Owner.Name,Owner.UserRole.Name,Domain__c FROM Contact WHERE ID IN :conSet ORDER BY LastModifiedDate DESC LIMIT 5]); conList1K = new List<Contact>([SELECT Id,AccountId,OwnerId,Account.Name,Name,Owner.Name,Owner.UserRole.Name,Domain__c FROM Contact WHERE ID IN :conSet ORDER BY LastModifiedDate DESC LIMIT 999]); oppShortList = new List<Opportunity>([SELECT Id,Name,Account.Name,AccountId,Amount,CloseDate,Territory_ID__c,Company_Size__c,OwnerId,Owner.Name,Owner.UserRole.Name,Type FROM Opportunity WHERE ID IN :oppSet ORDER BY CloseDate ASC LIMIT 5]); existingTaskShortList = new List<Task>([SELECT Id,OwnerId,Owner.Name,Owner.UserRole.Name,ActivityDate,Type,Subject,Who.Name,What.Name,WhoId,WhatId FROM Task WHERE OwnerId IN :repSet AND (WhoId IN :whoSet OR WhatId IN :whatSet) AND ActivityDate<=TODAY AND ActivityDate>=LAST_N_DAYS:120 AND Status ='Completed' AND (Type LIKE 'Call%' OR Type LIKE 'Email%' OR Type = 'Follow up') ORDER BY ActivityDate DESC LIMIT 5]); existingTasks1K = new List<Task>([SELECT Id,OwnerId,Owner.Name,Owner.UserRole.Name,ActivityDate,Type,Subject,Who.Name,What.Name,WhoId,WhatId FROM Task WHERE OwnerId IN :repSet AND (WhoId IN :whoSet OR WhatId IN :whatSet) AND ActivityDate<=TODAY AND ActivityDate>=LAST_N_DAYS:120 AND Status ='Completed' AND (Type LIKE 'Call%' OR Type LIKE 'Email%' OR Type = 'Follow up') ORDER BY ActivityDate DESC LIMIT 999]); openTaskShortList = new List<Task>([SELECT Id,OwnerId,Owner.Name,Owner.UserRole.Name,ActivityDate,Type,Subject,Who.Name,What.Name,WhoId,WhatId FROM Task WHERE OwnerId IN :repSet AND (WhoId IN :whoSet OR WhatId IN :whatSet) AND ActivityDate>=TODAY AND Status !='Completed' AND (NOT Subject LIKE '%Automated%') AND (Type LIKE 'Call%' OR Type LIKE 'Email%' OR Type = 'Follow up') ORDER BY LastModifiedDate DESC LIMIT 5]); openTasks1K = new List<Task>([SELECT Id,OwnerId,Owner.Name,Owner.UserRole.Name,ActivityDate,Type,Subject,Who.Name,What.Name,WhoId,WhatId FROM Task WHERE OwnerId IN :repSet AND (WhoId IN :whoSet OR WhatId IN :whatSet) AND ActivityDate>=TODAY AND Status !='Completed' AND (NOT Subject LIKE '%Automated%') AND (Type LIKE 'Call%' OR Type LIKE 'Email%' OR Type = 'Follow up') ORDER BY ActivityDate DESC LIMIT 999]); leadShortList = new List<Lead>([SELECT Id,Name,Company,Domain__c,Website,Territory_ID__c,Company_Size__c,Part_of_Prospecting_List__c,Matched_Account__c,Matched_Account__r.Name,OwnerId,Owner.Name,Owner.UserRole.Name FROM Lead WHERE Id IN :leadSet ORDER BY LastModifiedDate DESC LIMIT 5]); leadList1K = new List<Lead>([SELECT Id,Name,Company,Domain__c,Website,Territory_ID__c,Company_Size__c,Part_of_Prospecting_List__c,Matched_Account__c,Matched_Account__r.Name,OwnerId,Owner.Name,Owner.UserRole.Name FROM Lead WHERE Id IN :leadSet ORDER BY LastModifiedDate DESC LIMIT 999]); accShortList = new List<Account>([SELECT Id,OwnerId,Name,Owner.UserRole.Name,Owner.Name,Territory_ID__c,Company_Size__c,Website,Domain_for_lead_conversion__c FROM Account WHERE ID IN :accSet ORDER BY LastModifiedDate DESC LIMIT 5]); accList1K = new List<Account>([SELECT Id,OwnerId,Name,Owner.UserRole.Name,Owner.Name,Territory_ID__c,Company_Size__c,Website,Domain_for_lead_conversion__c FROM Account WHERE ID IN :accSet ORDER BY LastModifiedDate DESC LIMIT 999]); existingTaskSize=existingTasks1K.size(); openTaskSize=openTasks1K.size(); } public pageReference clear(){ leadList.clear(); return null; } //method for Show More (leads) public pageReference showMore(){ PageReference leadPage = new PageReference('/apex/showMore'); return leadPage; } //method for Show More (Contacts) public pageReference showMoreContacts(){ PageReference conPage = new PageReference('/apex/showMoreContacts'); return conPage; } //method for Show More (Accounts) public pageReference showMoreAccounts(){ PageReference conPage = new PageReference('/apex/showMoreAccounts'); return conPage; } //method for Show More (open tasks) public pageReference showMoreOpenTasks(){ PageReference openPage = new PageReference('/apex/showMoreOpenTasks'); return openPage; } //method for Show More (existing tasks) public pageReference showMoreExistingTasks(){ PageReference exPage = new PageReference('/apex/showMoreExistingTasks'); return exPage; } }
-
- Jean Grey 10
- August 13, 2018
- Like
- 0
View State Error or Page Timeout in Visualforce
I have a page that searches the database for matching records based on domain. The page works great in sandbox but we have a lot more data in production and the search will not run. I get a view state error or a timeout every time I try to run a search.
I've tried changing some variables to transient. I also tried limiting the rows on the Visualforce page. What else can I do to shorten the execution time so my search will run?
VF Page:
I've tried changing some variables to transient. I also tried limiting the rows on the Visualforce page. What else can I do to shorten the execution time so my search will run?
VF Page:
<apex:page standardController="Lead" extensions="domainSearchPage" recordSetVar="dom" tabStyle="Lead" sidebar="false"> <apex:form > <apex:pageBlock title="Cross-Check Domain to locate all related records"> <apex:outputText value="Enter Each Domain to Search: " /> <apex:inputText value="{!domain}" label="Domain"/> <apex:commandButton value="Search" action="{!search}" >strong text </apex:commandButton> <a href="/apex/domainSearchPage"> Start Over</a> <br/> </apex:pageBlock> <apex:pageBlock title="Search Results"> <table cellspacing="5" cellpadding="5" border="1px"> <tr> <td width="100px" style="background-color:#c38af2"><apex:outputLabel ><strong>Opportunities</strong></apex:outputLabel></td> <td width="100px" style="background-color:#ffc6a0"><apex:outputLabel ><strong>Open Tasks</strong></apex:outputLabel></td> <td width="150px" style="background-color:#ffc6a0"><apex:outputLabel ><strong>Completed Tasks</strong> <td width="75px" style="background-color:#77d3f4"><apex:outputLabel ><strong>Leads</strong></apex:outputLabel></td> <td width="75px" style="background-color:#77d3f4"><apex:outputLabel ><strong>Contacts</strong></apex:outputLabel></td> <td width="75px" style="background-color:#77d3f4"><apex:outputLabel ><strong>Accounts</strong></apex:outputLabel></td> <td width="75px" style="background-color:#ffff56"><apex:outputLabel ><strong>Reps</strong></apex:outputLabel></td> </apex:outputLabel></td> </tr> <tr> <td style="background-color:#c38af2"><strong><apex:outputText >{!oppSize}</apex:outputText></strong></td> <td style="background-color:#ffc6a0"><strong><apex:outputText >{!openTaskSize}</apex:outputText></strong></td> <td style="background-color:#ffc6a0"><strong><apex:outputText >{!existingTaskSize}</apex:outputText></strong></td> <td style="background-color:#77d3f4"><strong><apex:outputText >{!leadSize}</apex:outputText></strong></td> <td style="background-color:#77d3f4"><strong><apex:outputText >{!conSize}</apex:outputText></strong></td> <td style="background-color:#77d3f4"><strong><apex:outputText >{!accSize}</apex:outputText></strong></td> <td style="background-color:#ffff56"><strong><apex:outputText >{!repSize}</apex:outputText></strong></td> </tr> </table> </apex:pageBlock> <apex:pageBlock tabStyle="Goals__c" title="Open Opportunity Details ({!oppSize})"> <apex:outputPanel rendered="{!IF(AND(NOT(ISBLANK(oppSize)),oppSize<1000),TRUE,FALSE)}"> <apex:pageblockTable id="opps" rows="5" value="{!oppShortList}" var="o"> <apex:column headerValue="Name"><apex:outputlink target="_blank" value="/{!o.Id}">{!o.Name}</apex:outputlink></apex:column> <apex:column headerValue="Account"><apex:outputlink target="_blank" value="/{!o.AccountId}">{!o.Account.Name}</apex:outputlink></apex:column> <apex:column headerValue="Type" value="{!o.Type}"/> <apex:column headerValue="Amount" value="{!o.Amount}"/> <apex:column headerValue="Close Date" value="{!o.CloseDate}" /> <apex:column headerValue="Territory" value="{!o.Territory_ID__c}" /> <apex:column headerValue="Company Size" value="{!o.Company_Size__c}"/> <apex:column headerValue="Owner Name" value="{!o.Owner.Name}" /> <apex:column headerValue="Owner Role" value="{!o.Owner.UserRole.Name}" /> </apex:pageblockTable> </apex:outputPanel> </apex:pageBlock> <apex:pageBlock tabStyle="Lead" title="Open Task Details ({!openTaskSize})"> <apex:outputPanel rendered="{!IF(AND(NOT(ISBLANK(openTaskSize)),openTaskSize<1000),TRUE,FALSE)}"> <apex:pageblockTable rows="5" value="{!openTasks}" var="t"> <apex:column headerValue="Subject"><apex:outputlink target="_blank" value="/{!t.Id}">{!t.Subject}</apex:outputlink></apex:column> <apex:column headerValue="Type" value="{!t.Type}"/> <apex:column headerValue="Lead/Contact Name"><apex:outputlink target="_blank" value="/{!t.WhoId}">{!t.Who.Name}</apex:outputlink></apex:column> <apex:column headerValue="Related To"><apex:outputlink target="_blank" value="/{!t.WhatId}">{!t.What.Name}</apex:outputlink></apex:column> <apex:column headerValue="Owner Name" value="{!t.Owner.Name}" /> <apex:column headerValue="Owner Role" value="{!t.Owner.UserRole.Name}" /> </apex:pageblockTable> <br /> <apex:commandLink target="_blank" style="color:green;" value="Show More" action="{!showMoreOpenTasks}"/> </apex:outputPanel> </apex:pageBlock> <apex:pageBlock tabStyle="Lead" title="Completed Task Details ({!existingTaskSize})"> <apex:outputPanel rendered="{!IF(AND(NOT(ISBLANK(existingTaskSize)),existingTaskSize<1000),TRUE,FALSE)}"> <apex:pageblockTable rows="5" value="{!existingTasks}" var="t"> <apex:column headerValue="Subject"><apex:outputlink target="_blank" value="/{!t.Id}">{!t.Subject}</apex:outputlink></apex:column> <apex:column headerValue="Type" value="{!t.Type}"/> <apex:column headerValue="Lead/Contact Name"><apex:outputlink target="_blank" value="/{!t.WhoId}">{!t.Who.Name}</apex:outputlink></apex:column> <apex:column headerValue="Related To"><apex:outputlink target="_blank" value="/{!t.WhatId}">{!t.What.Name}</apex:outputlink></apex:column> <apex:column headerValue="Owner Name" value="{!t.Owner.Name}" /> <apex:column headerValue="Owner Role" value="{!t.Owner.UserRole.Name}" /> </apex:pageblockTable> <br /> <apex:commandLink target="_blank" style="color:green;" value="Show More" action="{!showMoreExistingTasks}"/> </apex:outputPanel> </apex:pageBlock> <!-- I do the same here for leads, contacts, and accounts. removed to save char space --> </apex:form>Apex Class:
public class domainSearchPage { //set up controller & extension for vf page ApexPages.StandardSetController setCont; public domainSearchPage(ApexPages.StandardSetController controllerD) { setCont = controllerD; } //set up variables public String domain {get;set;} transient List<Lead> leadList {get;set;} transient Set<Id> leadSet {get;set;} public Integer leadSize {get;set;} transient List<Lead> matchedLeads {get;set;} transient List<Account> accList {get;set;} transient Set<Id> accSet {get;set;} public Integer accSize {get;set;} transient List<Account> matchedAccounts {get;set;} transient List<Contact> conList {get;set;} transient Set<Id> conSet {get;set;} public Integer conSize {get;set;} transient List<Contact> matchedContacts {get;set;} transient List<Opportunity> oppList {get;set;} transient Set<Id> oppSet {get;set;} public Integer oppSize {get;set;} transient List<Opportunity> matchedOpps {get;set;} transient List<Task> taskList {get;set;} transient Set<Id> taskSet {get;set;} transient Set<Id> existingTaskSet {get;set;} transient Set<Id> openTaskSet {get;set;} public Integer openTaskSize {get;set;} public List<Task> openTasks {get;set;} public List<Task> existingTasks {get;set;} public Integer existingTaskSize {get;set;} transient Set<Id> whoSet {get;set;} transient Set<Id> whatSet {get;set;} transient Set<Id> repSet {get;set;} public Integer repSize {get;set;} public List<Lead> leadShortList {get;set;} public List<Contact> conShortList {get;set;} public List<Task> openTaskShortList {get;set;} public List<Task> existingTaskShortList {get;set;} public List<Account> accShortList {get;set;} public List<Opportunity> oppShortList {get;set;} public List<Opportunity> oppListTest {get;set;} public List<OpportunityContactRole> ocrList {get;set;} public List<Lead> leadList1K {get;set;} public List<Contact> conList1K {get;set;} public List<Task> existingTasks1K {get;set;} public List<Task> openTasks1K {get;set;} public List<Account> accList1K {get;set;} public void search(){ leadSet= new Set<Id>(); conSet = new Set<Id>(); accSet = new Set<Id>(); oppSet = new Set<Id>(); taskSet = new Set<Id>(); whoSet = new Set<Id>(); whatSet = new Set<Id>(); existingTaskSet = new Set<Id>(); openTaskSet = new Set<Id>(); repSet = new Set<Id>(); leadList = new List<Lead>(); matchedLeads = new List<Lead>(); conList = new List<Contact>(); oppList = new List<Opportunity>(); ocrList = new List<OpportunityContactRole>(); oppShortList= new List<Opportunity>(); existingTasks= new List<Task>(); openTasks= new List<Task>(); leadList.clear(); matchedLeads.clear(); conList.clear(); oppList.clear(); ocrList.clear(); oppShortList.clear(); existingTasks.clear(); openTasks.clear(); openTaskSize=NULL; leadSet.clear(); conSet.clear(); accSet.clear(); oppSet.clear(); taskSet.clear(); whoSet.clear(); whatSet.clear(); existingTaskSet.clear(); openTaskSet.clear(); repSet.clear(); PageReference newPage = new PageReference('/apex/domainSearchPage'); leadList = new List<Lead>(); conList=new List<Contact>(); existingTasks=new List<Task>(); openTasks=new List<Task>(); accList=new List<Account>(); oppList=new List<Opportunity>(); oppShortList=new List<Opportunity>(); //set up domain string //find related leads String leadQuery = 'SELECT Id,Name,Domain__c,Part_of_Prospecting_List__c,Territory_ID__c,Company_Size__c,Matched_Account__c,Matched_Account__r.Name,Website,OwnerId,Owner.Name,Owner.UserRole.Name,Company FROM Lead where IsConverted=FALSE AND (Domain__c LIKE \'%'+domain+'%\' OR Website LIKE \'%'+domain+'%\' OR Matched_Account__r.Domain_for_lead_conversion__c LIKE \'%'+domain+'%\' OR Matched_Account__r.Website LIKE \'%'+domain+'%\')'; leadList = Database.query(leadQuery); system.debug('domain '+domain); system.debug('leadList '+leadList); for(Lead l :leadList){ leadSet.add(l.Id); whoSet.add(l.Id); repSet.add(l.OwnerId); } leadSize=leadSet.size(); leadList1K = new List<Lead>([SELECT Id,Name,Domain__c,Part_of_Prospecting_List__c,Territory_ID__c,Company_Size__c,Matched_Account__c,Matched_Account__r.Name,Website,OwnerId,Owner.Name,Owner.UserRole.Name,Company FROM Lead WHERE ID IN :leadSet ORDER BY LastModifiedDate DESC LIMIT 999]); //find related accounts String accQuery = 'SELECT Id,OwnerId,Name,Owner.UserRole.Name,Owner.Name,Territory_ID__c,Company_Size__c,Website,Domain_for_lead_conversion__c FROM Account WHERE Domain_for_lead_conversion__c LIKE \'%'+domain+'%\' OR Website LIKE \'%'+domain+'%\''; accList = Database.query(accQuery); for(Account a :accList){ accSet.add(a.Id); whatSet.add(a.Id); repSet.add(a.OwnerId); } //find related contacts String conQuery = 'SELECT Id,AccountId,OwnerId,Account.Name,Name,Owner.Name,Owner.UserRole.Name,Domain__c FROM Contact WHERE Email LIKE \'%'+domain+'%\' OR Account.Website LIKE \'%'+domain+'%\' OR Account.Domain_for_lead_conversion__c LIKE \'%'+domain+'%\' OR Account.Website LIKE \'%'+domain+'%\''; conList = Database.query(conQuery); for(Contact c :conList){ conSet.add(c.Id); accSet.add(c.AccountId); whoSet.add(c.Id); whatSet.add(c.AccountId); repSet.add(c.OwnerId); } conSize=conSet.size(); //find related opps ocrList = new List<OpportunityContactRole>([SELECT Id,ContactId,Contact.OwnerId,OpportunityId FROM OpportunityContactRole WHERE ContactId IN :conSet OR Contact.AccountId IN :accSet]); for(OpportunityContactRole ocr :ocrList){ oppSet.add(ocr.OpportunityId); whatSet.add(ocr.OpportunityId); whoSet.add(ocr.ContactId); repSet.add(ocr.Contact.OwnerId); conSet.add(ocr.ContactId); } oppList = new List<Opportunity>([SELECT Id,Name,Account.Name,AccountId,Amount,CloseDate,Territory_ID__c,Company_Size__c,OwnerId,Owner.Name,Owner.UserRole.Name,Type FROM Opportunity WHERE CloseDate>=TODAY AND IsClosed=FALSE AND AccountId IN :accSet]); for(Opportunity o :oppList){ oppSet.add(o.Id); whatSet.add(o.Id); repSet.add(o.OwnerId); } oppSize=oppSet.size(); accSize=accSet.size(); //find related tasks openTasks = new List<Task>([SELECT Id,OwnerId,Owner.Name,Owner.UserRole.Name,ActivityDate,Type,Subject,Who.Name,What.Name,WhoId,WhatId FROM Task WHERE (WhoId IN :whoSet OR WhatId IN :whatSet) AND ActivityDate>=TODAY AND Status !='Completed' AND (NOT Subject LIKE '%Automated%')]); openTaskSize = openTasks.size(); for(Task t :openTasks){ repSet.add(t.OwnerId); openTaskSet.add(t.Id); } existingTasks = new List<Task>([SELECT Id,OwnerId,Owner.Name,Owner.UserRole.Name,ActivityDate,Type,Subject,Who.Name,What.Name,WhoId,WhatId FROM Task WHERE (WhoId IN :whoSet OR WhatId IN :whatSet) AND ActivityDate<=TODAY AND ActivityDate>=LAST_N_DAYS:90 AND Status ='Completed' AND (Type LIKE '%Call%' OR Type LIKE '%Email%' OR Type LIKE 'Follow up')]); existingTaskSize=0; if(existingTasks.size()!=0){ existingTaskSize = existingTasks.size(); for(Task t :existingTasks){ repSet.add(t.OwnerId); existingTaskSet.add(t.Id); } } repSize=repSet.size(); //short lists leadShortList = new List<Lead>([SELECT Id,Name,Company,Domain__c,Website,Territory_ID__c,Company_Size__c,Part_of_Prospecting_List__c,Matched_Account__c,Matched_Account__r.Name,OwnerId,Owner.Name,Owner.UserRole.Name FROM Lead WHERE Id IN :leadSet ORDER BY LastModifiedDate DESC LIMIT 5]); conShortList = new List<Contact>([SELECT Id,AccountId,OwnerId,Account.Name,Name,Owner.Name,Owner.UserRole.Name,Domain__c FROM Contact WHERE ID IN :conSet ORDER BY LastModifiedDate DESC LIMIT 5]); conList1K = new List<Contact>([SELECT Id,AccountId,OwnerId,Account.Name,Name,Owner.Name,Owner.UserRole.Name,Domain__c FROM Contact WHERE ID IN :conSet ORDER BY LastModifiedDate DESC LIMIT 999]); oppShortList = new List<Opportunity>([SELECT Id,Name,Account.Name,AccountId,Amount,CloseDate,Territory_ID__c,Company_Size__c,OwnerId,Owner.Name,Owner.UserRole.Name,Type FROM Opportunity WHERE ID IN :oppSet ORDER BY CloseDate DESC LIMIT 5]); existingTaskShortList = new List<Task>([SELECT Id,OwnerId,Owner.Name,Owner.UserRole.Name,ActivityDate,Type,Subject,Who.Name,What.Name,WhoId,WhatId FROM Task WHERE ID IN :existingTaskSet ORDER BY ActivityDate DESC LIMIT 5]); openTaskShortList = new List<Task>([SELECT Id,OwnerId,Owner.Name,Owner.UserRole.Name,ActivityDate,Type,Subject,Who.Name,What.Name,WhoId,WhatId FROM Task WHERE ID IN :openTaskSet ORDER BY LastModifiedDate DESC LIMIT 5]); existingTasks1K = new List<Task>([SELECT Id,OwnerId,Owner.Name,Owner.UserRole.Name,ActivityDate,Type,Subject,Who.Name,What.Name,WhoId,WhatId FROM Task WHERE ID IN :existingTaskSet ORDER BY ActivityDate DESC LIMIT 999]); openTasks1K = new List<Task>([SELECT Id,OwnerId,Owner.Name,Owner.UserRole.Name,ActivityDate,Type,Subject,Who.Name,What.Name,WhoId,WhatId FROM Task WHERE ID IN :openTaskSet ORDER BY ActivityDate DESC LIMIT 999]); accShortList = new List<Account>([SELECT Id,OwnerId,Name,Owner.UserRole.Name,Owner.Name,Territory_ID__c,Company_Size__c,Website,Domain_for_lead_conversion__c FROM Account WHERE ID IN :accSet ORDER BY LastModifiedDate DESC LIMIT 5]); accList1K = new List<Account>([SELECT Id,OwnerId,Name,Owner.UserRole.Name,Owner.Name,Territory_ID__c,Company_Size__c,Website,Domain_for_lead_conversion__c FROM Account WHERE ID IN :accSet ORDER BY LastModifiedDate DESC LIMIT 999]); }
-
- Jean Grey 10
- July 24, 2018
- Like
- 0
Display Accounts with Related Data on Visualforce Page
I'm trying to display Accounts on a Visualforce page with counts of tasks, leads, opps, owners, etc. I believe have all of the data in my controller but I'm not sure how to connect each variable to the appropriate account. Is Wrapper class the correct approach? What about a map? If anyone can share an idea or documentation that I could learn from it would be much appreciated.
public class WrapperDemoController{ //variables to hold all related data public Set<Id> accSet {get;set;} public List<Account> accList {get;set;} public Set<Id> conSet {get;set;} public List<Contact> conList {get;set;} public Set<Id> leadSet {get;set;} public List<Lead> leadList {get;set;} public List<Lead> leadListAll {get;set;} public DateTime nowDate {get;set;} public Date todayDate {get;set;} public Set<Id> whoSet {get;set;} public Set<Id> whatSet {get;set;} public Set<Id> ocrSet {get;set;} public List<OpportunityContactRole> ocrList {get;set;} public List<Opportunity> oppList {get;set;} public Set<Id> oppSet {get;set;} public Set<Id> taskSet {get;set;} public List<Task> taskList {get;set;} public Set<Id> repSet {get;set;} public List<User> repList {get;set;} /*Our Wrapper Class*/ public class TableRow{ public String accName {get;set;} public String domain {get;set;} public Integer countLeads {get;set;} public Integer countOpenTasks {get;set;} public Integer countReps {get;set;} public Integer countOpenOpps {get;set;} public Integer countExistingTasks {get;set;} } /*Public Property that will hold all the TableRows and our PageBlockTable can use this Variable to get the whole List of rows*/ public List<TableRow> rowList {get; set;} /*Constructor that runs a SOQL to get all the Records and build the List. This get executed automatically on the Page Load.*/ public WrapperDemoController(){ nowDate = system.now(); todayDate = system.today(); rowList = new List<TableRow>(); TableRow tr; //Sets whoSet = new Set<Id>(); accSet = new Set<Id>(); whatSet = new Set<Id>(); conSet = new Set<Id>(); leadSet = new Set<Id>(); ocrSet = new Set<Id>(); oppSet = new Set<Id>(); repSet = new Set<Id>(); //leads that have reporting matched account populated. This will be the list of Accounts we look at leadList = new List<Lead<([SELECT Id,OwnerId,Owner.Name,Matched_Account__c, Matched_Account__r.Name FROM Lead WHERE Matched_Account__c!=NULL AND IsConverted=FALSE]); system.debug('leadList '+leadList); //first fill the account set. This is the Set to find all related data for(Lead l : leadList){ accSet.add(l.Matched_Account__r.Id); whatSet.add(l.Matched_Account__r.Id); repSet.add(l.OwnerId); } //now get all the leads leadListAll = new List<Lead>([SELECT Id,OwnerId,Owner.Name,Matched_Account__c, Matched_Account__r.Name FROM Lead WHERE Matched_Account__r.Id IN :accSet]); //now fill the contact list conList = new List<Contact>([SELECT Id,OwnerId,Owner.Name,AccountId,Account.Name FROM Contact WHERE AccountId IN :accSet]); //now use the lead and contact lists to fill the whoSet for(Lead l :leadListAll){ whoSet.add(l.Id); repSet.add(l.OwnerId); } for(Contact c :conList){ whoSet.add(c.Id); conSet.add(c.Id); repSet.add(c.OwnerId); } //find all ocrs related to these contacts or accounts //might need to split into searching by accounts and contacts separately, to avoid SOQL limits ocrList = new List<OpportunityContactRole> ([SELECT Id,OpportunityId,ContactId,Contact.AccountId,Opportunity.StageName,Opportunity.Amount FROM OpportunityContactRole WHERE Opportunity.StageName !='Duplicate Quote' AND IsClosed=FALSE AND (ContactId IN :conSet OR Contact.AccountId IN :accSet)]); for(OpportunityContactRole ocr :ocrList){ oppSet.add(ocr.OpportunityId); conSet.add(ocr.ContactId); whoSet.add(ocr.ContactId); whatSet.add(ocr.OpportunityId); whatSet.add(ocr.Contact.AccountId); accSet.add(ocr.Contact.AccountId); repSet.add(ocr.Contact.OwnerId); repSet.add(ocr.Contact.Account.OwnerId); repSet.add(ocr.Opportunity.OwnerId); } //find all opps by account oppList = new List<Opportunity>([SELECT Id,StageName,Amount,OwnerId,Owner.Name FROM Opportunity WHERE IsClosed=FALSE AND CloseDate>:todayDate AND AccountId IN :accSet]); for(Opportunity o :oppList){ oppSet.add(o.Id); whatSet.add(o.Id); repSet.add(o.OwnerId); } //now find all open tasks related to any above data taskList = [SELECT Id,OwnerId,WhoId,WhatId FROM Tasks WHERE Status!='Completed' AND ActivityDate>:todayDate AND (Type = 'Call/Email' OR Type = 'Call_Email' OR Type = 'Call:TT' OR Type = 'Call:LM' OR Type = 'Call:Demo' OR Type = 'Call:Other' OR Type = 'Follow_up' OR Type = 'Follow up') AND (WhoId IN :whoSet OR WhatId IN :whatSet)]; /*Building the List of TableRows*/ /*Fetch all the Contacts and then build the List*/ for(Account a : accSet){ tr = new TableRow(); tr.accName = acc.Name; tr.domain = acc.Domain; tr.countLeads = ; tr.countOpenOpps = ; tr.countOpenTasks = ; tr.countReps = ; /*Add the TableRow to the List then and there*/ rowList.add(tr); } } }
-
- Jean Grey 10
- July 11, 2018
- Like
- 0
Method defined as TestMethod do not support web service callouts, but I'm not using a callout
I'm getting this error but my code does not have any callouts. I received the same error when I tried to deploy with default test settings, which is what we always use. None of the test classes that threw an error are using callouts. How do I fix this? See code below of one of the test classes that gave an error.
@isTest(seeAllData=true) public class countOppsTest { static testMethod void validateTrigger(){ Test.startTest(); DateTime dateNow = system.now(); Date dateToday = system.today(); Account acct = new Account( name = 'Test Account' ); insert acct; Contact cont = new Contact( accountId = acct.id, firstName = 'Test', lastName = 'Contact', title = 'Test' ); insert cont; Goals__c goal = new Goals__c( Name = 'Test Goal', Amount__c = 1000, Date__c = dateToday, Active__c = TRUE, Opportunity__c = NULL ); insert goal; Opportunity opp = new Opportunity( Name = 'Test Opp', StageName = 'In Purchasing', CloseDate = dateToday, Amount = 1400, AccountId = acct.Id, LeadSource = 'Other' ); insert opp; opp.StageName = 'Closed–Won'; opp.Closed_Won_Reason__c = 'Test'; opp.Closed_Won_Explanation__c = 'Test'; update opp; ID sysAdm = [SELECT Id FROM Profile WHERE Name = 'System Administrator'].Id; //user test data List<User> userList = new List<User>(); User u = new User(ProfileId = sysAdm,LastName = 'last',Email = 'testuser@test.com', Username = 'testuser@test.com' + System.currentTimeMillis(), CompanyName = 'TEST',Title = 'title',Alias = 'alias', TimeZoneSidKey = 'America/Los_Angeles',EmailEncodingKey = 'UTF-8', LanguageLocaleKey = 'en_US',LocaleSidKey = 'en_US'); userList.add(u); insert userList; system.debug('opp '+opp+' goal '+goal); system.assertEquals(goal.Amount__c, 1000); } }
-
- Jean Grey 10
- July 02, 2018
- Like
- 0
Mixed DML Operation Error on Trigger
I'm getting this error, but I'm confused because I'm only doing one DML when I insert the User History list.
My trigger:
My class:
Can anyone help? Thanks in advance!
My trigger:
trigger userHistory on User (before update) { system.debug('--------------------------------------------start of trigger: userHistory'); system.debug('Number of Queries used in this apex code so far: ' + Limits.getQueries()); system.debug('Number of rows queried in this apex code so far: ' + Limits.getDmlRows()); system.debug('Number of script statements used so far : ' + Limits.getDmlStatements()); system.debug('CPU usage so far: '+Limits.getCpuTime()); list<User> userList =new list<User>(); userList=trigger.new; system.debug('userList '+userList); public List<User_History__c> histList =new List<User_History__c>(); if(Trigger.isUpdate){ for(User u:userList){ // FirstName if(trigger.newmap.get(u.id).FirstName !=trigger.oldmap.get(u.id).FirstName){ User_History__c h = new User_History__c(); h.User__c=u.Id; h.Field__c='FirstName'; h.OldValue__c=trigger.oldmap.get(u.id).FirstName; h.NewValue__c=trigger.newmap.get(u.id).FirstName; histList.add(h); } // LastName if(trigger.newmap.get(u.id).LastName !=trigger.oldmap.get(u.id).LastName){ User_History__c h = new User_History__c(); h.User__c=u.Id; h.Field__c='LastName'; h.OldValue__c=trigger.oldmap.get(u.id).LastName; h.NewValue__c=trigger.newmap.get(u.id).LastName; histList.add(h); } // ProfileId if(trigger.newmap.get(u.id).Profile__c !=trigger.oldmap.get(u.id).Profile__c){ User_History__c h = new User_History__c(); h.User__c=u.Id; h.Field__c='Profile'; h.OldValue__c=trigger.oldmap.get(u.id).Profile__c; h.NewValue__c=trigger.newmap.get(u.id).Profile__c; histList.add(h); } // Role.Name if(trigger.newmap.get(u.id).UserRole__c !=trigger.oldmap.get(u.id).UserRole__c){ User_History__c h = new User_History__c(); h.User__c=u.Id; h.Field__c='UserRole'; h.OldValue__c = trigger.oldmap.get(u.id).UserRole__c; h.NewValue__c = trigger.newmap.get(u.id).UserRole__c; histList.add(h); } // ManagerId if(trigger.newmap.get(u.id).ManagerId !=trigger.oldmap.get(u.id).ManagerId){ User_History__c h = new User_History__c(); h.User__c=u.Id; h.Field__c='ManagerId'; h.OldValue__c=trigger.oldmap.get(u.id).Manager.Name; h.NewValue__c=trigger.newmap.get(u.id).Manager.Name; histList.add(h); } // Quota if(trigger.newmap.get(u.id).This_Month_s_Quota__c !=trigger.oldmap.get(u.id).This_Month_s_Quota__c){ User_History__c h = new User_History__c(); h.User__c=u.Id; h.Field__c='Quota'; decimal oldQuota = trigger.oldmap.get(u.id).This_Month_s_Quota__c; h.OldValue__c=oldQuota.format(); decimal newQuota = trigger.newmap.get(u.id).This_Month_s_Quota__c; h.NewValue__c=newQuota.format(); histList.add(h); } } } try{ userHistoryList insertHistLit = new userHistoryList(); userHistoryList.insertList(histList); system.debug('histList '+histList); } catch(DMLException e1){ system.debug(e1); } try{ insert histList; } catch(DMLException e2){ system.debug(e2); } system.debug('--------------------------------------------end of trigger: userHistory'); system.debug('Number of Queries used in this apex code so far: ' + Limits.getQueries()); system.debug('Number of rows queried in this apex code so far: ' + Limits.getDmlRows()); system.debug('Number of script statements used so far : ' + Limits.getDmlStatements()); system.debug('Final CPU Usage: '+Limits.getCpuTime()); system.debug('Final heap size: ' + Limits.getHeapSize()); }
My class:
public class userHistoryList { public static void insertList(List<User_History__c> historyList){ insert historyList; } }The error:
12:44:21:054 USER_DEBUG [94]|DEBUG|System.DmlException: Insert failed. First exception on row 0; first error: MIXED_DML_OPERATION, DML operation on setup object is not permitted after you have updated a non-setup object (or vice versa): User_History__c, original object: User: []
Can anyone help? Thanks in advance!
-
- Jean Grey 10
- June 26, 2018
- Like
- 0
Trigger add contact to campaign
I'm working on a trigger to add contacts to a campaign based on temporary fields stored on the contact. This is working for new campaign members inserted, but I need to update the status on the campaign member record if it changes on the contact. I understand I need to update the existing campaign member rather than try to insert a new one, but I'm unsure how to go about that. Here is my code:
Any help is much appreciated!
trigger addToCampaignContact on Contact (after insert, after update) { public List<CampaignMember> cmList = new List<CampaignMember>(); public Set<Id> conSet = new Set<Id>(); public List<Contact> conList = new List<Contact>(); //create list of contact members to add for(Contact c:Trigger.New){ if(c.Campaign_Id__c != NULL && c.Member_Status__c != NULL){ conSet.add(c.Id); cmList.add( new CampaignMember( CampaignId = c.Campaign_Id__c, ContactId = c.Id, Status = c.Member_Status__c, UTM_Name__c = c.UTM_Campaign_Name__c, Content__c= c.UTM_Content__c, Medium__c=c.UTM_Medium__c, Source__c=c.UTM_Source__c, UTM_Term__c=c.UTM_Term__c )); }} system.debug('insert cmList '+cmList); if(!cmList.isEmpty()){ insert cmList;} } /* //update contacts back to null to prep for future campaign conList = [SELECT Id, Campaign_Id__c, Member_Status__c, UTM_Campaign_Name__c FROM Contact WHERE Id IN :conSet]; for(Contact c:conList){ c.Campaign_Id__c=NULL; c.Member_Status__c=NULL; c.UTM_Source__c=NULL; c.UTM_Medium__c=NULL; c.UTM_Campaign_Name__c=NULL; c.UTM_Content__c=NULL; c.UTM_Term__c=NULL; } system.debug('update conList '+conList); //update conList; */Error when I try to update the member status field on the contact to update the campaign member:
Error:Apex trigger addToCampaignContact caused an unexpected exception, contact your administrator: addToCampaignContact: execution of AfterUpdate caused by: System.DmlException: Insert failed. First exception on row 0; first error: DUPLICATE_VALUE, Already a campaign member.: []: Trigger.addToCampaignContact: line 32, column 1
Any help is much appreciated!
-
- Jean Grey 10
- June 18, 2018
- Like
- 0
Test Class for Search Page
I'm having a hard time with this test class - seems simple enough but I'm getting a "Constructor not defined" error. Can anyone help?
Class:
Test:
Class:
public class mtaSearch{ public Date startDate {get;set;} public Date endDate {get;set;} public String vendor {get;set;} public String accName {get;set;} public String searchQuery {get;set;} public String leadQuery {get;set;} public String accountQuery {get;set;} public Integer querySize {get;set;} public Integer leadQuerySize {get;set;} public String searchString {get;set;} public List<Account> accList {get;set;} public Set<Id> accSet {get;set;} public List<Lead> leadList {get;set;} public Set<Id> leadSet {get;set;} public List<Contact> conList {get;set;} public List<Contact> conList1 {get;set;} public Set<Id> conSet {get;set;} public String contactQuery {get;set;} public Integer contactQuerySize {get;set;} public String contactQuery1 {get;set;} public List<OpportunityContactRole> ocrList {get;set;} public Set<Id> ocrSet {get;set;} public Integer ocrSize {get;set;} public List<Task> taskSearch {get;set;} public List<CampaignMember> cmSearch {get;set;} public List<Campaign> campSearch {get;set;} public List<Opportunity> oppList {get;set;} public Set<Id> oppSet {get;set;} public Integer oppSize {get;set;} public List<Opportunity> wonOppList {get;set;} public Set<Id> wonOppSet {get;set;} public Integer wonOppSize {get;set;} public List<Lead> cvList {get;set;} public Set<Id> cvSet {get;set;} public Integer cvSize {get;set;} public String cvLeadQuery {get;set;} public List<Task> taskList {get;set;} public Set<Id> taskSet {get;set;} public Date todayDate {get;set;} public object totalWon {get;set;} public String sWon {get;set;} public Decimal QTotal {get;set;} public List<String> argsTotal{get;set;} //set up controller & extension for vf page ApexPages.StandardSetController setCon; public mtaSearch(ApexPages.StandardSetController controller) { setCon = controller; } public void search(){ //set up string to display $ argsTotal= new String[]{'0','number','###','###','##0.00'}; //set up date variable todayDate = system.today(); if(startDate==NULL){startDate=todayDate-90;} if(endDate==NULL){endDate=todayDate;} accountQuery = 'SELECT Name,Id from Account where Name LIKE \'%'+accName+'%\' LIMIT 50000'; accList = Database.query(accountQuery); querySize = accList.size(); accSet = new Set<Id>(); if(accList.size()>0){ for(Account a:accList){accSet.add(a.Id);}} leadQuery = 'SELECT Name,Id from Lead where (Company LIKE \'%'+accName+'%\' OR Origination__c LIKE \'%'+vendor+'%\') AND IsConverted =FALSE LIMIT 50000'; leadList = Database.query(leadQuery); leadQuerySize = leadList.size(); leadSet = new Set<Id>(); if(leadList.size()>0){ for(Lead l:leadList){leadSet.add(l.Id);}} cvLeadQuery = 'SELECT Name,Id,ConvertedContactId from Lead where (Company LIKE \'%'+accName+'%\' OR Origination__c LIKE \'%'+vendor+'%\') AND IsConverted =TRUE LIMIT 50000'; cvList = Database.query(cvLeadQuery); cvSize = cvList.size(); cvSet = new Set<Id>(); if(cvList.size()>0){ for(Lead l:cvList){leadSet.add(l.Id);}} conList = new List<Contact>([SELECT Name, Id FROM Contact WHERE Id IN:cvSet]); conSet = new Set<Id>(); if(conList.size()>0){ for(Contact c:conList){conSet.add(c.Id);}} system.debug('conSet '+conSet); contactQuery1 = 'SELECT Name,Id from Contact where (Account.Name LIKE \'%'+accName+'%\' OR Origination__c LIKE \'%'+vendor+'%\') LIMIT 50000'; system.debug('contactQuery1 '+contactQuery1); conList1 = Database.query(contactQuery1); system.debug('conList1 '+conList1); if(conList1.size()>0){ for(Contact c:conList1){conSet.add(c.Id);}} system.debug('conSet '+conSet); contactQuerySize = conSet.size(); ocrList = new List<OpportunityContactRole> ([SELECT Id,OpportunityId,ContactId,Contact.AccountId,Opportunity.StageName FROM OpportunityContactRole WHERE ContactId IN :conSet LIMIT 50000]); oppSet = new Set<Id>(); if(ocrList.size()>0){ for(OpportunityContactRole ocr :ocrList){ oppSet.add(ocr.OpportunityId); oppSize = oppSet.size(); }} wonOppList = new List<Opportunity>([SELECT Id, Owner.UserRole.Name, Original_Lead_Source__c, Name,StageName, Amount,OwnerId, Owner.Name,CreatedDate,CloseDate FROM Opportunity WHERE StageName = 'Closed–Won' AND Id IN :oppSet LIMIT 50000]); wonOppSet = new Set<Id>(); if(wonOppList.size()>0){ for(Opportunity o:wonOppList){wonOppSet.add(o.Id);} wonOppSize = wonOppSet.size(); //get total amount of won opps for this search List<AggregateResult> wonAgg = new List<AggregateResult> ([SELECT Sum(Amount) FROM Opportunity WHERE Id IN :wonOppSet]); if(wonAgg.size()>0){ totalWon = wonAgg[0].get('expr0');} if(totalWon==NULL){QTotal=0;} if(integer.valueOf(totalWon)>0){ QTotal = integer.valueOf(totalWon);} sWon = String.format(qTotal.format(), argsTotal);} }
Test:
@isTest(seeAllData=true) public class mtaSearchTest { static testMethod void validateSearch(){ Test.startTest(); DateTime dateNow = system.now(); Date dateToday = system.today(); Account acct = new Account( name = 'Test Account' ); insert acct; Contact cont = new Contact( accountId = acct.id, firstName = 'Test', lastName = 'Contact', title = 'Test' ); insert cont; Opportunity opp = new Opportunity( Name = 'Test Opp', StageName = 'In Purchasing', CloseDate = dateToday, Amount = 1400, AccountId = acct.Id, LeadSource = 'Other' ); insert opp; opp.StageName = 'Closed–Won'; opp.Closed_Won_Reason__c = 'Data Breach'; opp.Closed_Won_Explanation__c = 'Test'; update opp; Opportunity oppLost = new Opportunity( Name = 'Test Opp', StageName = 'In Purchasing', CloseDate = dateToday, Amount = 1500, AccountId = acct.Id, LeadSource = 'Other'); insert oppLost; ID sysAdm = [SELECT Id FROM Profile WHERE Name = 'System Administrator'].Id; //user test data List<User> userList = new List<User>(); User u = new User(ProfileId = sysAdm,LastName = 'last',Email = 'testuser@test.com', Username = 'testuser@test.com' + System.currentTimeMillis(), CompanyName = 'TEST',Title = 'title',Alias = 'alias', TimeZoneSidKey = 'America/Los_Angeles',EmailEncodingKey = 'UTF-8', LanguageLocaleKey = 'en_US',LocaleSidKey = 'en_US'); userList.add(u); insert userList; PageReference pageRef = Page.mtaSearch; pageRef.getParameters().put('query', 'test'); Test.setCurrentPage(pageRef); //this is where i get the error mtaSearch doSearch=new mtaSearch(); doSearch.search(); } }
-
- Jean Grey 10
- June 04, 2018
- Like
- 0
Map of User Ids and Total Sales this Month
I have a trigger on Opp, after each insert/update I need to recalculate a user's sales for the month. I have the user set and I can do an aggregate query, but how do I connect these in the map?
Here is what I have so far, any help would be appreciated!
Here is what I have so far, any help would be appreciated!
//get total current count of opps oppList = [SELECT Id,Amount FROM Opportunity WHERE CloseDate = THIS_MONTH AND StageName = 'Closed–Won']; //add opps to set to get comm splits for(Opportunity o:oppList){ oppSet.add(o.Id); } //get aggregate of all commission splits csAgg = [SELECT SUM(Split_Amount__c),User__c FROM Commission_Split__c WHERE Opportunity__c IN :oppSet]; //get comm splits attached to this opp csList = [SELECT Id,Split_Amount__c,User__c,Opportunity__c FROM Commission_Split__c WHERE Opportunity__c IN :oppSet]; //get users for(Commission_Split__c c:csList){ userSet.add(c.User__r.Id); } //now I have my users in a Set, and aggregate results above, how do I connect? public Map<Id, AggregateResult> userSales = new Map<Id,AggregateResult>([SELECT SUM(Split_Amount__c) FROM Commission_Split__c WHERE User__c IN :userSet)]);
-
- Jean Grey 10
- June 02, 2018
- Like
- 0
Match user to user lookup on custom object to update field in for loop
I have a custom object Daily_Forecast__c, with a field called Forecast_Amount__c (currency) and another field that looks up to User. For each day I need to update the custom field on User.Monday__c with the corresponding Forecast_Amount__c from the Daily_Forecast__c object. There will only ever be one forecast record for each day. I feel I am almost there but my for loop is looping through all forecast records instead of just the one with the correct user lookup. How do I match the User to the Forecast record in my loop?
Please help! Thanks in advance.
//list of forecasts for user & direct reports (this is working) fList = new List<Daily_Forecast__c>([SELECT Id,User__r.Name,User__r.Team__c,User__r.Territory__c,User__r.UserRole.Name,Split_Forecast_Amount__c,Forecast_Amount__c,Day__c,Day_Date__c,RecordType.Name FROM Daily_Forecast__c WHERE Date__c = THIS_WEEK AND (RecordType.Name = 'Daily Forecast' OR RecordType.Name='Weekly Forecast - Monday' OR RecordType.Name='Weekly Forecast - Thursday') AND User__c IN :myTeam]); system.debug('fList '+fList); //MONDAY - add forecast records from monday to monday list (this is working) MondayListE = new List<Daily_Forecast__c>(); for(Daily_Forecast__c e :fList){ if(e.Day__c=='Monday' && e.RecordType.Name=='Daily Forecast'){ MondayListE.add(e);}} system.debug('MondayListE should be daily forecasts from fList with day=monday '+MondayListE); //get users who are represented in the list of Monday forecasts (this is working) userMon = new List<User>([SELECT Id, Name,Monday__c FROM User WHERE Id IN (SELECT User__c FROM Daily_Forecast__c WHERE Id IN :MondayListE)]); system.debug('userMon '+userMon); //my for loop - should loop through the list of users and update them with monday's forecast amount - not working - every user ends up with the same amount, the forecast amount from the last user in the list if(userMon.size()>0){ for(User u :userMon){ for(Daily_Forecast__c f :MondayListE){ u.Monday__c = f.Forecast_Amount__c; update u; system.debug('updates on users '+u); } }
Please help! Thanks in advance.
-
- Jean Grey 10
- May 22, 2018
- Like
- 0
Display user's daily sales in apex datatable (Visualforce)
I have an apex datatable returning some User fields from a list in my apex class. I would like to include a column "This Month's Sales" which would return the total $ of opps closed won this month. I can run the SOQL in my class but I'm not sure how to get the correct result per user into the table, i.e. how to put it into the correct row and column in my table. Can I attach this $amount to each user without creating a custom field?
-
- Jean Grey 10
- May 21, 2018
- Like
- 0
Display Error Message from Unique Field Error
We have a unique field on an object, populated by workflow (datestamp + userId), which prevents a user from submitting two records on the same day. I'd like to display an error message on the visualforce input page, but the normal error message approaches don't seem to be working. Is there some special way I need to display this error? Here is my controller:
And vf page:
What is the correct way to catch and display the unique/duplicate error message on the vf page? Without any error handling i get the expected INSERT FAILED error screen, which is not user-friendly. With the exception catching above, no message shows at all. How can I program the exception handling to display a user-friendly error message? Thanks in advance.
public class NewDailyForecast { public Daily_Forecast__c fc {get;set;} public String userId {get;set;} public Date todayDate {get;set;} public String dailyType {get;set;} public String weeklyType {get;set;} public String fName {get;set;} public double fAmount {get;set;} public String recType {get;set;} public List<Daily_Forecast__c> thisWeekDaily {get;set;} public List<Daily_Forecast__c> thisWeekWeekly {get;set;} public List<Daily_Forecast__c> checkUnique {get;set;} //set up controller & extension for vf page ApexPages.StandardSetController setCon; public NewDailyForecast(ApexPages.StandardSetController controller) { setCon = controller; } public void getValues(){ //get logged in user userId = UserInfo.getUserId(); system.debug('userId '+userId); //get today's date todayDate = system.today(); system.debug('todayDate '+todayDate); //get record type dailyType = [SELECT Id FROM RecordType WHERE Name = 'Daily Forecast'].Id; weeklyType = [SELECT Id FROM RecordType WHERE Name = 'Weekly Forecast'].Id; system.debug('dailyType '+dailyType); system.debug('weeklyType '+weeklyType); fName = userId + todayDate; fAmount=0; //new forecast record on page fc = new Daily_Forecast__c (RecordTypeId = dailyType, Date__c = todayDate, Forecast_Amount__c = fAmount, User__c = userId); system.debug('fc '+fc); //list of related forecast records thisWeekDaily = new List<Daily_Forecast__c>([SELECT Id, Date__c, User__c, User__r.UserRole.Name, User__r.Territory__c, Forecast_Amount__c, RecordTypeId, RecordType.Name FROM Daily_Forecast__c WHERE User__c = :userId AND Date__c = THIS_WEEK AND RecordTypeId = :dailyType ORDER BY Date__c ASC]); system.debug('thisWeekDaily '+thisWeekDaily); thisWeekWeekly = new List<Daily_Forecast__c>([SELECT Id, Date__c, User__c, User__r.UserRole.Name, User__r.Territory__c, Forecast_Amount__c, RecordTypeId, RecordType.Name FROM Daily_Forecast__c WHERE User__c = :userId AND Date__c = THIS_WEEK AND RecordTypeId = :weeklyType ORDER BY Date__c ASC]); system.debug('thisWeekWeekly '+thisWeekWeekly); } public PageReference saveRecord(){ //error catching method 1: build list to match existing and throw error if list>0 //this doesn't work checkUnique = new List<Daily_Forecast__c>([SELECT Id, Date__c, User__c FROM Daily_Forecast__c WHERE Date__c = :fc.Date__c AND User__c = :fc.User__c]); /* if(checkUnique.size()>0){ system.debug('checkUnique '+checkUnique); ApexPages.Message myFatalMsg = new ApexPages.Message(ApexPages.Severity.FATAL,'Duplicate record created. Please try again'); ApexPages.addMessage(myFatalMsg); system.debug('fatal error '+myFatalMsg); ApexPages.Message myErrorMsg = new ApexPages.Message(ApexPages.Severity.ERROR,'Error Message'); ApexPages.addMessage(myErrorMsg); system.debug('error '+myErrorMsg); } insert fc; */ //error catching method 2: try insert and catch exception //This doesn't work either try { insert fc; } catch(DMLException e) { for(Daily_Forecast__c df :thisWeekDaily){ df.addError('Duplicate entry not allowed'); } system.debug(e); ApexPages.addMessages(e); ApexPages.Message myFatalMsg = new ApexPages.Message(ApexPages.Severity.FATAL,'Duplicate record created. Please try again'); ApexPages.addMessage(myFatalMsg); return null; } return new pageReference( '/' + fc.id ); } }
And vf page:
<apex:page standardController="Daily_Forecast__c" extensions="NewDailyForecast" recordSetVar="forecasts" tabStyle="Daily_Forecast__c" sidebar="false" action="{!getValues}"> <apex:form > <apex:pageblock > <apex:messages /> </apex:pageblock> <apex:pageBlock title="Create New Forecast" mode="edit"> <apex:pageBlockButtons > <apex:commandButton value="Save" action="{!saveRecord}" rerender="error"/> <apex:commandButton value="Cancel" action="{!cancel}"/> </apex:pageBlockButtons> <apex:pageBlockSection > <apex:inputField label="Forecast Type" value="{!fc.RecordTypeId}" id="recType" /> <apex:inputField label="Amount" value="{!fc.Forecast_Amount__c}" id="fAmount" /> <apex:inputField label="User Name" value="{!fc.User__c}" id="fUser" /> <apex:inputField label="Date" value="{!fc.Date__c}" id="fDate" /> </apex:pageBlockSection> </apex:pageBlock> <apex:pageBlock title="This Week's Forecast"> <apex:pageBlockSection > <apex:pageBlockTable id="thisWeek" value="{!thisWeekDaily}" var="d" > <apex:column headerValue="Day"><apex:outputText value="{0, date, EEEE}"> <apex:param value="{!d.Date__c}" /> </apex:outputText></apex:column> <apex:column headerValue="Forecast Amount"><apex:outputField value="{!d.Forecast_Amount__c}"/></apex:column> <apex:column headerValue="Type"><apex:outputField value="{!d.RecordType.Name}" /></apex:column> </apex:pageBlockTable> <apex:pageBlockTable id="thisWeekWeekly" value="{!thisWeekWeekly}" var="w" > <apex:column headerValue="Day"><apex:outputText value="{0, date, EEEE}"> <apex:param value="{!w.Date__c}" /> </apex:outputText></apex:column> <apex:column headerValue="Forecast Amount"><apex:outputField value="{!w.Forecast_Amount__c}"/></apex:column> <apex:column headerValue="Type"><apex:outputField value="{!w.RecordType.Name}" /></apex:column> </apex:pageBlockTable> </apex:pageBlockSection> </apex:pageBlock> </apex:form> </apex:page>
What is the correct way to catch and display the unique/duplicate error message on the vf page? Without any error handling i get the expected INSERT FAILED error screen, which is not user-friendly. With the exception catching above, no message shows at all. How can I program the exception handling to display a user-friendly error message? Thanks in advance.
-
- Jean Grey 10
- March 21, 2018
- Like
- 0
Match up data on VF page
I am using Apex to find all opportunities related to certain tasks:
public with sharing class DemoOppsController { //set up controller & extension for vf page ApexPages.StandardSetController setCon; public DemoOppsController(ApexPages.StandardSetController controller) { setCon = controller; } //set up variables public List<Task> demoList {get;set;} public List<Task> ownerList {get;set;} public List<Id> repIdList {get;set;} public List<User> repList {get;set;} public List<Id> conIdList {get;set;} public List<Contact> conList {get;set;} public List<Id> accIdList {get;set;} public Set<Id> accSet {get;set;} public List<Account> accList {get;set;} public List<Id> oppIdList {get;set;} public Set<Id> oppSet {get;set;} public List<Opportunity> oppList {get;set;} public List<Id> IdList {get;set;} public List<Wrapper> wrapTastic {get;set;} public void getDemos(){ //get tasks demoList = new List<Task> ([SELECT Id, ActivityDate, WhoId,Who.Name,Owner.Name, Type,Subject,Status,AccountId, OwnerId,Owner.UserRole.Name, WhatId FROM Task WHERE ActivityDate<=TODAY AND ActivityDate=THIS_YEAR AND Type='Call:DM' ORDER BY Owner.UserRole.Name]); //get rep Ids repIdList = new List<Id>(); repList = new List<User>(); for(Task t :demoList){ repIdList.add(t.OwnerId); for(User u :[SELECT Id,Name,UserRole.Name,Territory__c From User WHERE Id IN :repIdList ORDER BY UserRole.Name]){ repList.add(u); } } system.debug('repIdList : '+repIdList); //get contact Ids conIdList = new List<Id>(); conList = new List<Contact>(); for(Task t :demoList){ conIdList.add(t.WhoId); for(Contact c :[SELECT Id, LeadSource,OwnerId,AccountId FROM Contact WHERE Id IN :conIdList ORDER BY Owner.UserRole.Name]){ conList.add(c); } } //get account Ids from Tasks accSet = new Set<Id>(); accList = new List<Account>(); for(Task t :demoList){ accSet.add(t.AccountId);} //get account Ids from conList for(Contact cons :conList){ accSet.add(cons.AccountId);} for(Account a :[SELECT Id, OwnerId,Owner.UserRole.Name FROM Account WHERE Id IN :accSet ORDER BY Owner.UserRole.Name]){ accList.add(a); } //get Opps Ids oppIdList = new List<Id>(); oppSet = new Set<Id>(); //may need to add commission split to this for(Opportunity opp :[SELECT Id,AccountId,Owner.UserRole.Name FROM Opportunity WHERE OwnerId IN :repIdList AND CloseDate = THIS_YEAR ORDER BY Owner.UserRole.Name]){ for(Account acc :accList){ if(acc.Id == opp.AccountId){ oppSet.add(opp.Id); } } } for(Commission_Split__c cs :[SELECT Id,User__c,Opportunity__c,Opportunity__r.Id FROM Commission_Split__c WHERE Opportunity__r.CloseDate = THIS_YEAR AND Opportunity__r.AccountId IN :accList]){ oppSet.add(cs.Opportunity__r.Id); } oppList = new List<Opportunity>([SELECT Id,Name,Amount,StageName,OwnerId,AccountId,Owner.UserRole.Name,Owner.Name FROM Opportunity WHERE Id IN :oppSet ORDER BY Owner.UserRole.Name]); system.debug('demoList '+demoList); system.debug('repList '+repList); system.debug('conList '+conList); system.debug('accList '+accList); system.debug('oppList '+oppList); } }I have confirmed in debug logs that this is working as expected. The problem is in displaying the data so that the opportunities appear next to the related tasks. Right now I just have two lists side by side, with no matching so the data is hard to read. Here is my vf page:
<apex:page standardController="User" extensions="DemoOppsController" recordSetVar="users" tabStyle="User" sidebar="false" action="{!getDemos}"> <style> #col1,#col2{width:45%;display:inline-block;} </style> <apex:pageBlock title="SMB Demos with Opportunity Info"> <div id="col1"> <apex:pageBlockSection title="Demos"> <apex:pageBlockTable value="{!demoList}" var="d"> <apex:column headerValue="Team" value="{!d.Owner.UserRole.Name}"></apex:column> <apex:column headerValue="Rep Name" value="{!d.Owner.Name}"></apex:column> <apex:column headerValue="Contact Name"><apex:outputLink value="{!d.WhoId}">{!d.Who.Name}</apex:outputLink></apex:column> <apex:column headerValue="Date" value="{!d.ActivityDate}"></apex:column> <apex:column headerValue="Demos" value="{!d.Subject}"></apex:column> </apex:pageBlockTable> </apex:pageBlockSection> </div> <div id="col2"> <apex:pageBlockSection title="Opportunities"> <apex:pageBlockTable value="{!oppList}" var="o"> <apex:column headerValue="Team" value="{!o.Owner.UserRole.Name}"></apex:column> <apex:column headerValue="Opportunity Owner" value="{!o.Owner.Name}"></apex:column> <apex:column headerValue="Opportunity Name"><apex:outputLink value="{!o.Id}">{!o.Name}</apex:outputLink></apex:column> </apex:pageBlockTable> </apex:pageBlockSection> </div> </apex:pageBlock> </apex:page>Any suggestions? Thanks in advance!
-
- Jean Grey 10
- March 13, 2018
- Like
- 0
Nested SOQL query to get both campaign and opportunity data from contact records
I am trying to build a query to help with buyer-journey reporting. I am trying to do this with only SOQL, not Apex. I think I might be querying the wrong object but I'm not sure how to build this query to give me everything I need.
Here is my query:
SELECT Id,FirstRespondedDate,Campaign.Name,Status,Date_Responded__c,CreatedDate,ContactId FROM CampaignMember WHERE CampaignId IN (SELECT CampaignId FROM Opportunity WHERE StageName = 'Closed–Won' AND CloseDate = THIS_YEAR) AND ContactId IN (SELECT ContactId FROM OpportunityContactRole)
This works and gives me almost everything I need, but I need to return CloseDate in addition to these other fields. Is this possible? Do I need to flip this and query the Contact object instead, using nested queries to grab both campaign member and opportunity fields?
Here is my query:
SELECT Id,FirstRespondedDate,Campaign.Name,Status,Date_Responded__c,CreatedDate,ContactId FROM CampaignMember WHERE CampaignId IN (SELECT CampaignId FROM Opportunity WHERE StageName = 'Closed–Won' AND CloseDate = THIS_YEAR) AND ContactId IN (SELECT ContactId FROM OpportunityContactRole)
This works and gives me almost everything I need, but I need to return CloseDate in addition to these other fields. Is this possible? Do I need to flip this and query the Contact object instead, using nested queries to grab both campaign member and opportunity fields?
-
- Jean Grey 10
- March 07, 2018
- Like
- 0
Remove white space when using conditional rendering on Visualforce component in Lightning view
I can't get rid of the white space above the Visualforce page that is embedded on my page layout. The Visualforce markup has conditional rendering depending on the user's display theme. The whitespace is only showing in Lightning, not in Classic (see screenshots).
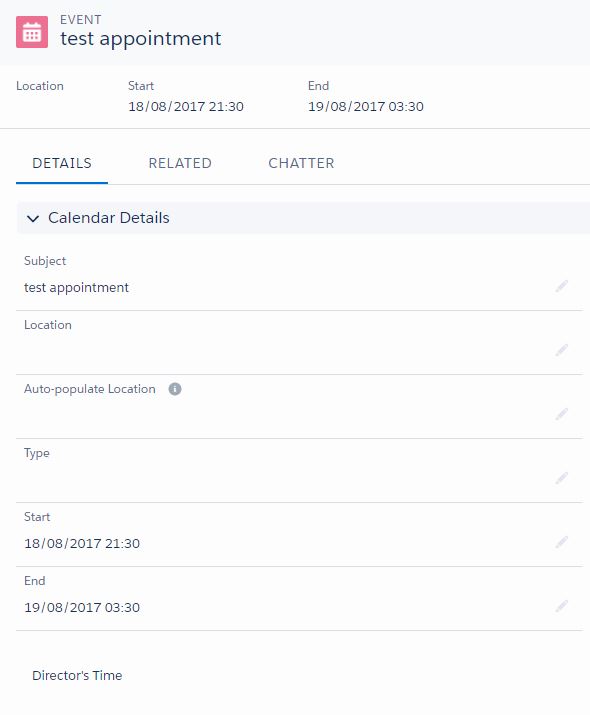
<apex:page standardController="Event" standardStylesheets="{!isClassic}" applyBodyTag="{!isClassic}" extensions="TZTranslateController" >
<apex:outputPanel rendered="{! !isClassic}">
<apex:slds />
</apex:outputPanel>
<div class="slds-scope">
<apex:outputPanel rendered="{!isClassic}">
<div style="font-size: 10;padding-left: 38%">
Director's Time
</div>
</apex:outputPanel>
<apex:outputPanel rendered="{! !isClassic}">
<div class="slds-grid slds-m-top_none">
Director's Time
</div>
</apex:outputPanel>
</div>
</apex:page>
<apex:outputPanel rendered="{! !isClassic}">
<apex:slds />
</apex:outputPanel>
<div class="slds-scope">
<apex:outputPanel rendered="{!isClassic}">
<div style="font-size: 10;padding-left: 38%">
Director's Time
</div>
</apex:outputPanel>
<apex:outputPanel rendered="{! !isClassic}">
<div class="slds-grid slds-m-top_none">
Director's Time
</div>
</apex:outputPanel>
</div>
</apex:page>
-
- Jean Grey 10
- August 24, 2017
- Like
- 0
Compare dates on child objects
I need to run a query to compare dates on child objects. We have a custom object Job Placements (child of Contacts) with Start Date and End Date fields. I need to find all Job Placements where the Start Date is within 30 days of an End Date from another Job Placement on the same Contact. Is this possible with SOQL or do I need to write a trigger to mark these records when they are created?
-
- Jean Grey 10
- August 15, 2017
- Like
- 0
How do I find the name of a custom field? I have the API id but don't know what field it's referring to.
One of our templates is referencing a field with {!Opportunity.someid} and I'm not sure what field the id is referring to. How do I search for this field name by the id?
-
- Jean Grey 10
- August 11, 2017
- Like
- 0
How can I find my instance number? I have my domain enabled.
I need to know the instance number for an external integration. It's not in my Salesforce URL because I have a custom domain. Is there a way I can look this up or query in dev console?
-
- Jean Grey 10
- August 09, 2017
- Like
- 0
access user roles through helper class
I wrote the following class that I intend to use as a helper class:
public class RoleUtils {
public static ID userId {get;set;} //variable for this user id
public static ID userRoleId {get;set;} //variable for this user role id
public static Set<ID> userSubs {get;set;} //variable for all subordinates to this user in role hierarchy
public static Set<ID> userSubRoles {get;set;}
public static Set<ID> userPar {get;set;} //variable for all parents to this user in role hierarchy
public static Set<ID> usergroup {get;set;}
public static void getIds(){
usergroup = new Set<ID>();
userId = UserInfo.getUserId();
usergroup.add(userId);
userRoleId = UserInfo.getUserRoleId();
userSubRoles = new Set<ID>();
for(UserRole subRole :[select Id from UserRole where ParentRoleId = :userRoleId AND ParentRoleID != null])
{
userSubRoles.add(subRole.Id);
}
for(User subuser :[SELECT ID, UserRoleId FROM User WHERE UserRoleId IN :userSubRoles])
{
usergroup.add(subuser.Id);
}
system.debug('userId '+userId);
system.debug('userRoleId '+userRoleId);
system.debug('userSubRoles '+userSubRoles);
system.debug('usergroup '+usergroup);
}
}
In another class I have:
Date currentDate = System.today();
Date minusonemonth = currentDate.addMonths(-1);
Date plusoneyear = currentDate.addYears(1);
Date plusthreemonths = currentDate.addDays(90);
RoleUtils roleUtil = new RoleUtils();
getCon = [SELECT RecordTypeId, Contract_Number__c, Owned_by_me__c, Account__c, Account__r.Name, Id, Name, Utility__c, Contract_Nom_Group__c, Supplier__c, Consultant__c, Contract_End_Date__c, Pro_forma_Annual_Margin__c, Annual_Contract_Volume__c
FROM NG_Contract__c
WHERE Contract_End_Date__c >= :minusonemonth
AND Contract_End_Date__c <= :plusthreemonths
ORDER BY Contract_End_Date__c ASC
LIMIT 10];
I would like to add a filter to my list to choose records owned by one of the users in my usergroup variable from my helper class. How do I do this?
public class RoleUtils {
public static ID userId {get;set;} //variable for this user id
public static ID userRoleId {get;set;} //variable for this user role id
public static Set<ID> userSubs {get;set;} //variable for all subordinates to this user in role hierarchy
public static Set<ID> userSubRoles {get;set;}
public static Set<ID> userPar {get;set;} //variable for all parents to this user in role hierarchy
public static Set<ID> usergroup {get;set;}
public static void getIds(){
usergroup = new Set<ID>();
userId = UserInfo.getUserId();
usergroup.add(userId);
userRoleId = UserInfo.getUserRoleId();
userSubRoles = new Set<ID>();
for(UserRole subRole :[select Id from UserRole where ParentRoleId = :userRoleId AND ParentRoleID != null])
{
userSubRoles.add(subRole.Id);
}
for(User subuser :[SELECT ID, UserRoleId FROM User WHERE UserRoleId IN :userSubRoles])
{
usergroup.add(subuser.Id);
}
system.debug('userId '+userId);
system.debug('userRoleId '+userRoleId);
system.debug('userSubRoles '+userSubRoles);
system.debug('usergroup '+usergroup);
}
}
In another class I have:
Date currentDate = System.today();
Date minusonemonth = currentDate.addMonths(-1);
Date plusoneyear = currentDate.addYears(1);
Date plusthreemonths = currentDate.addDays(90);
RoleUtils roleUtil = new RoleUtils();
getCon = [SELECT RecordTypeId, Contract_Number__c, Owned_by_me__c, Account__c, Account__r.Name, Id, Name, Utility__c, Contract_Nom_Group__c, Supplier__c, Consultant__c, Contract_End_Date__c, Pro_forma_Annual_Margin__c, Annual_Contract_Volume__c
FROM NG_Contract__c
WHERE Contract_End_Date__c >= :minusonemonth
AND Contract_End_Date__c <= :plusthreemonths
ORDER BY Contract_End_Date__c ASC
LIMIT 10];
I would like to add a filter to my list to choose records owned by one of the users in my usergroup variable from my helper class. How do I do this?
-
- Jean Grey 10
- July 19, 2017
- Like
- 0
Null Pointer Exception Error from new lines of code
I've added just a few lines of code for time zone handling and the page is now throwing an error on submit.
I added these lines in the submit method:

adjustment is a public variable declared in the top of the code.
Here is my error from the log (screenshot):
I added these lines in the submit method:
adjustment is a public variable declared in the top of the code.
Here is my error from the log (screenshot):
-
- Jean Grey 10
- May 22, 2017
- Like
- 0
Display Accounts with Related Data on Visualforce Page
I'm trying to display Accounts on a Visualforce page with counts of tasks, leads, opps, owners, etc. I believe have all of the data in my controller but I'm not sure how to connect each variable to the appropriate account. Is Wrapper class the correct approach? What about a map? If anyone can share an idea or documentation that I could learn from it would be much appreciated.
public class WrapperDemoController{ //variables to hold all related data public Set<Id> accSet {get;set;} public List<Account> accList {get;set;} public Set<Id> conSet {get;set;} public List<Contact> conList {get;set;} public Set<Id> leadSet {get;set;} public List<Lead> leadList {get;set;} public List<Lead> leadListAll {get;set;} public DateTime nowDate {get;set;} public Date todayDate {get;set;} public Set<Id> whoSet {get;set;} public Set<Id> whatSet {get;set;} public Set<Id> ocrSet {get;set;} public List<OpportunityContactRole> ocrList {get;set;} public List<Opportunity> oppList {get;set;} public Set<Id> oppSet {get;set;} public Set<Id> taskSet {get;set;} public List<Task> taskList {get;set;} public Set<Id> repSet {get;set;} public List<User> repList {get;set;} /*Our Wrapper Class*/ public class TableRow{ public String accName {get;set;} public String domain {get;set;} public Integer countLeads {get;set;} public Integer countOpenTasks {get;set;} public Integer countReps {get;set;} public Integer countOpenOpps {get;set;} public Integer countExistingTasks {get;set;} } /*Public Property that will hold all the TableRows and our PageBlockTable can use this Variable to get the whole List of rows*/ public List<TableRow> rowList {get; set;} /*Constructor that runs a SOQL to get all the Records and build the List. This get executed automatically on the Page Load.*/ public WrapperDemoController(){ nowDate = system.now(); todayDate = system.today(); rowList = new List<TableRow>(); TableRow tr; //Sets whoSet = new Set<Id>(); accSet = new Set<Id>(); whatSet = new Set<Id>(); conSet = new Set<Id>(); leadSet = new Set<Id>(); ocrSet = new Set<Id>(); oppSet = new Set<Id>(); repSet = new Set<Id>(); //leads that have reporting matched account populated. This will be the list of Accounts we look at leadList = new List<Lead<([SELECT Id,OwnerId,Owner.Name,Matched_Account__c, Matched_Account__r.Name FROM Lead WHERE Matched_Account__c!=NULL AND IsConverted=FALSE]); system.debug('leadList '+leadList); //first fill the account set. This is the Set to find all related data for(Lead l : leadList){ accSet.add(l.Matched_Account__r.Id); whatSet.add(l.Matched_Account__r.Id); repSet.add(l.OwnerId); } //now get all the leads leadListAll = new List<Lead>([SELECT Id,OwnerId,Owner.Name,Matched_Account__c, Matched_Account__r.Name FROM Lead WHERE Matched_Account__r.Id IN :accSet]); //now fill the contact list conList = new List<Contact>([SELECT Id,OwnerId,Owner.Name,AccountId,Account.Name FROM Contact WHERE AccountId IN :accSet]); //now use the lead and contact lists to fill the whoSet for(Lead l :leadListAll){ whoSet.add(l.Id); repSet.add(l.OwnerId); } for(Contact c :conList){ whoSet.add(c.Id); conSet.add(c.Id); repSet.add(c.OwnerId); } //find all ocrs related to these contacts or accounts //might need to split into searching by accounts and contacts separately, to avoid SOQL limits ocrList = new List<OpportunityContactRole> ([SELECT Id,OpportunityId,ContactId,Contact.AccountId,Opportunity.StageName,Opportunity.Amount FROM OpportunityContactRole WHERE Opportunity.StageName !='Duplicate Quote' AND IsClosed=FALSE AND (ContactId IN :conSet OR Contact.AccountId IN :accSet)]); for(OpportunityContactRole ocr :ocrList){ oppSet.add(ocr.OpportunityId); conSet.add(ocr.ContactId); whoSet.add(ocr.ContactId); whatSet.add(ocr.OpportunityId); whatSet.add(ocr.Contact.AccountId); accSet.add(ocr.Contact.AccountId); repSet.add(ocr.Contact.OwnerId); repSet.add(ocr.Contact.Account.OwnerId); repSet.add(ocr.Opportunity.OwnerId); } //find all opps by account oppList = new List<Opportunity>([SELECT Id,StageName,Amount,OwnerId,Owner.Name FROM Opportunity WHERE IsClosed=FALSE AND CloseDate>:todayDate AND AccountId IN :accSet]); for(Opportunity o :oppList){ oppSet.add(o.Id); whatSet.add(o.Id); repSet.add(o.OwnerId); } //now find all open tasks related to any above data taskList = [SELECT Id,OwnerId,WhoId,WhatId FROM Tasks WHERE Status!='Completed' AND ActivityDate>:todayDate AND (Type = 'Call/Email' OR Type = 'Call_Email' OR Type = 'Call:TT' OR Type = 'Call:LM' OR Type = 'Call:Demo' OR Type = 'Call:Other' OR Type = 'Follow_up' OR Type = 'Follow up') AND (WhoId IN :whoSet OR WhatId IN :whatSet)]; /*Building the List of TableRows*/ /*Fetch all the Contacts and then build the List*/ for(Account a : accSet){ tr = new TableRow(); tr.accName = acc.Name; tr.domain = acc.Domain; tr.countLeads = ; tr.countOpenOpps = ; tr.countOpenTasks = ; tr.countReps = ; /*Add the TableRow to the List then and there*/ rowList.add(tr); } } }
- Jean Grey 10
- July 11, 2018
- Like
- 0
Convert string date to Date in Apex
Hi,
I have String date as "Jul 27, 2018". How to convert to Date in Apex
I have String date as "Jul 27, 2018". How to convert to Date in Apex
- Pandeiswari
- July 11, 2018
- Like
- 0
Method defined as TestMethod do not support web service callouts, but I'm not using a callout
I'm getting this error but my code does not have any callouts. I received the same error when I tried to deploy with default test settings, which is what we always use. None of the test classes that threw an error are using callouts. How do I fix this? See code below of one of the test classes that gave an error.
@isTest(seeAllData=true) public class countOppsTest { static testMethod void validateTrigger(){ Test.startTest(); DateTime dateNow = system.now(); Date dateToday = system.today(); Account acct = new Account( name = 'Test Account' ); insert acct; Contact cont = new Contact( accountId = acct.id, firstName = 'Test', lastName = 'Contact', title = 'Test' ); insert cont; Goals__c goal = new Goals__c( Name = 'Test Goal', Amount__c = 1000, Date__c = dateToday, Active__c = TRUE, Opportunity__c = NULL ); insert goal; Opportunity opp = new Opportunity( Name = 'Test Opp', StageName = 'In Purchasing', CloseDate = dateToday, Amount = 1400, AccountId = acct.Id, LeadSource = 'Other' ); insert opp; opp.StageName = 'Closed–Won'; opp.Closed_Won_Reason__c = 'Test'; opp.Closed_Won_Explanation__c = 'Test'; update opp; ID sysAdm = [SELECT Id FROM Profile WHERE Name = 'System Administrator'].Id; //user test data List<User> userList = new List<User>(); User u = new User(ProfileId = sysAdm,LastName = 'last',Email = 'testuser@test.com', Username = 'testuser@test.com' + System.currentTimeMillis(), CompanyName = 'TEST',Title = 'title',Alias = 'alias', TimeZoneSidKey = 'America/Los_Angeles',EmailEncodingKey = 'UTF-8', LanguageLocaleKey = 'en_US',LocaleSidKey = 'en_US'); userList.add(u); insert userList; system.debug('opp '+opp+' goal '+goal); system.assertEquals(goal.Amount__c, 1000); } }
- Jean Grey 10
- July 02, 2018
- Like
- 0
Mixed DML Operation Error on Trigger
I'm getting this error, but I'm confused because I'm only doing one DML when I insert the User History list.
My trigger:
My class:
Can anyone help? Thanks in advance!
My trigger:
trigger userHistory on User (before update) { system.debug('--------------------------------------------start of trigger: userHistory'); system.debug('Number of Queries used in this apex code so far: ' + Limits.getQueries()); system.debug('Number of rows queried in this apex code so far: ' + Limits.getDmlRows()); system.debug('Number of script statements used so far : ' + Limits.getDmlStatements()); system.debug('CPU usage so far: '+Limits.getCpuTime()); list<User> userList =new list<User>(); userList=trigger.new; system.debug('userList '+userList); public List<User_History__c> histList =new List<User_History__c>(); if(Trigger.isUpdate){ for(User u:userList){ // FirstName if(trigger.newmap.get(u.id).FirstName !=trigger.oldmap.get(u.id).FirstName){ User_History__c h = new User_History__c(); h.User__c=u.Id; h.Field__c='FirstName'; h.OldValue__c=trigger.oldmap.get(u.id).FirstName; h.NewValue__c=trigger.newmap.get(u.id).FirstName; histList.add(h); } // LastName if(trigger.newmap.get(u.id).LastName !=trigger.oldmap.get(u.id).LastName){ User_History__c h = new User_History__c(); h.User__c=u.Id; h.Field__c='LastName'; h.OldValue__c=trigger.oldmap.get(u.id).LastName; h.NewValue__c=trigger.newmap.get(u.id).LastName; histList.add(h); } // ProfileId if(trigger.newmap.get(u.id).Profile__c !=trigger.oldmap.get(u.id).Profile__c){ User_History__c h = new User_History__c(); h.User__c=u.Id; h.Field__c='Profile'; h.OldValue__c=trigger.oldmap.get(u.id).Profile__c; h.NewValue__c=trigger.newmap.get(u.id).Profile__c; histList.add(h); } // Role.Name if(trigger.newmap.get(u.id).UserRole__c !=trigger.oldmap.get(u.id).UserRole__c){ User_History__c h = new User_History__c(); h.User__c=u.Id; h.Field__c='UserRole'; h.OldValue__c = trigger.oldmap.get(u.id).UserRole__c; h.NewValue__c = trigger.newmap.get(u.id).UserRole__c; histList.add(h); } // ManagerId if(trigger.newmap.get(u.id).ManagerId !=trigger.oldmap.get(u.id).ManagerId){ User_History__c h = new User_History__c(); h.User__c=u.Id; h.Field__c='ManagerId'; h.OldValue__c=trigger.oldmap.get(u.id).Manager.Name; h.NewValue__c=trigger.newmap.get(u.id).Manager.Name; histList.add(h); } // Quota if(trigger.newmap.get(u.id).This_Month_s_Quota__c !=trigger.oldmap.get(u.id).This_Month_s_Quota__c){ User_History__c h = new User_History__c(); h.User__c=u.Id; h.Field__c='Quota'; decimal oldQuota = trigger.oldmap.get(u.id).This_Month_s_Quota__c; h.OldValue__c=oldQuota.format(); decimal newQuota = trigger.newmap.get(u.id).This_Month_s_Quota__c; h.NewValue__c=newQuota.format(); histList.add(h); } } } try{ userHistoryList insertHistLit = new userHistoryList(); userHistoryList.insertList(histList); system.debug('histList '+histList); } catch(DMLException e1){ system.debug(e1); } try{ insert histList; } catch(DMLException e2){ system.debug(e2); } system.debug('--------------------------------------------end of trigger: userHistory'); system.debug('Number of Queries used in this apex code so far: ' + Limits.getQueries()); system.debug('Number of rows queried in this apex code so far: ' + Limits.getDmlRows()); system.debug('Number of script statements used so far : ' + Limits.getDmlStatements()); system.debug('Final CPU Usage: '+Limits.getCpuTime()); system.debug('Final heap size: ' + Limits.getHeapSize()); }
My class:
public class userHistoryList { public static void insertList(List<User_History__c> historyList){ insert historyList; } }The error:
12:44:21:054 USER_DEBUG [94]|DEBUG|System.DmlException: Insert failed. First exception on row 0; first error: MIXED_DML_OPERATION, DML operation on setup object is not permitted after you have updated a non-setup object (or vice versa): User_History__c, original object: User: []
Can anyone help? Thanks in advance!
- Jean Grey 10
- June 26, 2018
- Like
- 0
Trigger add contact to campaign
I'm working on a trigger to add contacts to a campaign based on temporary fields stored on the contact. This is working for new campaign members inserted, but I need to update the status on the campaign member record if it changes on the contact. I understand I need to update the existing campaign member rather than try to insert a new one, but I'm unsure how to go about that. Here is my code:
Any help is much appreciated!
trigger addToCampaignContact on Contact (after insert, after update) { public List<CampaignMember> cmList = new List<CampaignMember>(); public Set<Id> conSet = new Set<Id>(); public List<Contact> conList = new List<Contact>(); //create list of contact members to add for(Contact c:Trigger.New){ if(c.Campaign_Id__c != NULL && c.Member_Status__c != NULL){ conSet.add(c.Id); cmList.add( new CampaignMember( CampaignId = c.Campaign_Id__c, ContactId = c.Id, Status = c.Member_Status__c, UTM_Name__c = c.UTM_Campaign_Name__c, Content__c= c.UTM_Content__c, Medium__c=c.UTM_Medium__c, Source__c=c.UTM_Source__c, UTM_Term__c=c.UTM_Term__c )); }} system.debug('insert cmList '+cmList); if(!cmList.isEmpty()){ insert cmList;} } /* //update contacts back to null to prep for future campaign conList = [SELECT Id, Campaign_Id__c, Member_Status__c, UTM_Campaign_Name__c FROM Contact WHERE Id IN :conSet]; for(Contact c:conList){ c.Campaign_Id__c=NULL; c.Member_Status__c=NULL; c.UTM_Source__c=NULL; c.UTM_Medium__c=NULL; c.UTM_Campaign_Name__c=NULL; c.UTM_Content__c=NULL; c.UTM_Term__c=NULL; } system.debug('update conList '+conList); //update conList; */Error when I try to update the member status field on the contact to update the campaign member:
Error:Apex trigger addToCampaignContact caused an unexpected exception, contact your administrator: addToCampaignContact: execution of AfterUpdate caused by: System.DmlException: Insert failed. First exception on row 0; first error: DUPLICATE_VALUE, Already a campaign member.: []: Trigger.addToCampaignContact: line 32, column 1
Any help is much appreciated!
- Jean Grey 10
- June 18, 2018
- Like
- 0
is there any way to deploy guest your profile.
Hi,
I created a force.com site, i applied many field permissions to guest user. now i need to deploy same profile with all field permissions to production.
Thanks:
Ramakrishna Reddy Gouni
I created a force.com site, i applied many field permissions to guest user. now i need to deploy same profile with all field permissions to production.
Thanks:
Ramakrishna Reddy Gouni
- Ramakrishna Reddy Gouni
- June 12, 2018
- Like
- 0
Help with column header in Visualforce
Please let me know if more info is needed, but this is what we had originally:
I then changed it by adding another filter to "rendered"...
It is working in that it is showing me only the Opportunity Products (OP) that have a Lease Service Group of "NQP", and it is showing the English names, but for some reason my headerValue "Description" no longer appears on the page. The column no longer has a header.
Is there something I don't understand or did wrong to cause this?
Any help would be appreciated!
<apex:column headerValue="Description" value="{!OP.PriceBookEntry.Name}" headerClass="column_ttl" rendered="{!Opportunity.Language__c = 'ENGLISH'}"> </apex:column>
I then changed it by adding another filter to "rendered"...
<apex:column headerValue="Description" value="{!OP.PriceBookEntry.Name}" headerClass="column_ttl" rendered="{!Opportunity.Language__c = 'ENGLISH' && OP.Lease_Service_Group__c = 'NQP'}"> </apex:column>
It is working in that it is showing me only the Opportunity Products (OP) that have a Lease Service Group of "NQP", and it is showing the English names, but for some reason my headerValue "Description" no longer appears on the page. The column no longer has a header.
Is there something I don't understand or did wrong to cause this?
Any help would be appreciated!
- Heather_Hanson
- June 12, 2018
- Like
- 0
Test Class for Search Page
I'm having a hard time with this test class - seems simple enough but I'm getting a "Constructor not defined" error. Can anyone help?
Class:
Test:
Class:
public class mtaSearch{ public Date startDate {get;set;} public Date endDate {get;set;} public String vendor {get;set;} public String accName {get;set;} public String searchQuery {get;set;} public String leadQuery {get;set;} public String accountQuery {get;set;} public Integer querySize {get;set;} public Integer leadQuerySize {get;set;} public String searchString {get;set;} public List<Account> accList {get;set;} public Set<Id> accSet {get;set;} public List<Lead> leadList {get;set;} public Set<Id> leadSet {get;set;} public List<Contact> conList {get;set;} public List<Contact> conList1 {get;set;} public Set<Id> conSet {get;set;} public String contactQuery {get;set;} public Integer contactQuerySize {get;set;} public String contactQuery1 {get;set;} public List<OpportunityContactRole> ocrList {get;set;} public Set<Id> ocrSet {get;set;} public Integer ocrSize {get;set;} public List<Task> taskSearch {get;set;} public List<CampaignMember> cmSearch {get;set;} public List<Campaign> campSearch {get;set;} public List<Opportunity> oppList {get;set;} public Set<Id> oppSet {get;set;} public Integer oppSize {get;set;} public List<Opportunity> wonOppList {get;set;} public Set<Id> wonOppSet {get;set;} public Integer wonOppSize {get;set;} public List<Lead> cvList {get;set;} public Set<Id> cvSet {get;set;} public Integer cvSize {get;set;} public String cvLeadQuery {get;set;} public List<Task> taskList {get;set;} public Set<Id> taskSet {get;set;} public Date todayDate {get;set;} public object totalWon {get;set;} public String sWon {get;set;} public Decimal QTotal {get;set;} public List<String> argsTotal{get;set;} //set up controller & extension for vf page ApexPages.StandardSetController setCon; public mtaSearch(ApexPages.StandardSetController controller) { setCon = controller; } public void search(){ //set up string to display $ argsTotal= new String[]{'0','number','###','###','##0.00'}; //set up date variable todayDate = system.today(); if(startDate==NULL){startDate=todayDate-90;} if(endDate==NULL){endDate=todayDate;} accountQuery = 'SELECT Name,Id from Account where Name LIKE \'%'+accName+'%\' LIMIT 50000'; accList = Database.query(accountQuery); querySize = accList.size(); accSet = new Set<Id>(); if(accList.size()>0){ for(Account a:accList){accSet.add(a.Id);}} leadQuery = 'SELECT Name,Id from Lead where (Company LIKE \'%'+accName+'%\' OR Origination__c LIKE \'%'+vendor+'%\') AND IsConverted =FALSE LIMIT 50000'; leadList = Database.query(leadQuery); leadQuerySize = leadList.size(); leadSet = new Set<Id>(); if(leadList.size()>0){ for(Lead l:leadList){leadSet.add(l.Id);}} cvLeadQuery = 'SELECT Name,Id,ConvertedContactId from Lead where (Company LIKE \'%'+accName+'%\' OR Origination__c LIKE \'%'+vendor+'%\') AND IsConverted =TRUE LIMIT 50000'; cvList = Database.query(cvLeadQuery); cvSize = cvList.size(); cvSet = new Set<Id>(); if(cvList.size()>0){ for(Lead l:cvList){leadSet.add(l.Id);}} conList = new List<Contact>([SELECT Name, Id FROM Contact WHERE Id IN:cvSet]); conSet = new Set<Id>(); if(conList.size()>0){ for(Contact c:conList){conSet.add(c.Id);}} system.debug('conSet '+conSet); contactQuery1 = 'SELECT Name,Id from Contact where (Account.Name LIKE \'%'+accName+'%\' OR Origination__c LIKE \'%'+vendor+'%\') LIMIT 50000'; system.debug('contactQuery1 '+contactQuery1); conList1 = Database.query(contactQuery1); system.debug('conList1 '+conList1); if(conList1.size()>0){ for(Contact c:conList1){conSet.add(c.Id);}} system.debug('conSet '+conSet); contactQuerySize = conSet.size(); ocrList = new List<OpportunityContactRole> ([SELECT Id,OpportunityId,ContactId,Contact.AccountId,Opportunity.StageName FROM OpportunityContactRole WHERE ContactId IN :conSet LIMIT 50000]); oppSet = new Set<Id>(); if(ocrList.size()>0){ for(OpportunityContactRole ocr :ocrList){ oppSet.add(ocr.OpportunityId); oppSize = oppSet.size(); }} wonOppList = new List<Opportunity>([SELECT Id, Owner.UserRole.Name, Original_Lead_Source__c, Name,StageName, Amount,OwnerId, Owner.Name,CreatedDate,CloseDate FROM Opportunity WHERE StageName = 'Closed–Won' AND Id IN :oppSet LIMIT 50000]); wonOppSet = new Set<Id>(); if(wonOppList.size()>0){ for(Opportunity o:wonOppList){wonOppSet.add(o.Id);} wonOppSize = wonOppSet.size(); //get total amount of won opps for this search List<AggregateResult> wonAgg = new List<AggregateResult> ([SELECT Sum(Amount) FROM Opportunity WHERE Id IN :wonOppSet]); if(wonAgg.size()>0){ totalWon = wonAgg[0].get('expr0');} if(totalWon==NULL){QTotal=0;} if(integer.valueOf(totalWon)>0){ QTotal = integer.valueOf(totalWon);} sWon = String.format(qTotal.format(), argsTotal);} }
Test:
@isTest(seeAllData=true) public class mtaSearchTest { static testMethod void validateSearch(){ Test.startTest(); DateTime dateNow = system.now(); Date dateToday = system.today(); Account acct = new Account( name = 'Test Account' ); insert acct; Contact cont = new Contact( accountId = acct.id, firstName = 'Test', lastName = 'Contact', title = 'Test' ); insert cont; Opportunity opp = new Opportunity( Name = 'Test Opp', StageName = 'In Purchasing', CloseDate = dateToday, Amount = 1400, AccountId = acct.Id, LeadSource = 'Other' ); insert opp; opp.StageName = 'Closed–Won'; opp.Closed_Won_Reason__c = 'Data Breach'; opp.Closed_Won_Explanation__c = 'Test'; update opp; Opportunity oppLost = new Opportunity( Name = 'Test Opp', StageName = 'In Purchasing', CloseDate = dateToday, Amount = 1500, AccountId = acct.Id, LeadSource = 'Other'); insert oppLost; ID sysAdm = [SELECT Id FROM Profile WHERE Name = 'System Administrator'].Id; //user test data List<User> userList = new List<User>(); User u = new User(ProfileId = sysAdm,LastName = 'last',Email = 'testuser@test.com', Username = 'testuser@test.com' + System.currentTimeMillis(), CompanyName = 'TEST',Title = 'title',Alias = 'alias', TimeZoneSidKey = 'America/Los_Angeles',EmailEncodingKey = 'UTF-8', LanguageLocaleKey = 'en_US',LocaleSidKey = 'en_US'); userList.add(u); insert userList; PageReference pageRef = Page.mtaSearch; pageRef.getParameters().put('query', 'test'); Test.setCurrentPage(pageRef); //this is where i get the error mtaSearch doSearch=new mtaSearch(); doSearch.search(); } }
- Jean Grey 10
- June 04, 2018
- Like
- 0
Map of User Ids and Total Sales this Month
I have a trigger on Opp, after each insert/update I need to recalculate a user's sales for the month. I have the user set and I can do an aggregate query, but how do I connect these in the map?
Here is what I have so far, any help would be appreciated!
Here is what I have so far, any help would be appreciated!
//get total current count of opps oppList = [SELECT Id,Amount FROM Opportunity WHERE CloseDate = THIS_MONTH AND StageName = 'Closed–Won']; //add opps to set to get comm splits for(Opportunity o:oppList){ oppSet.add(o.Id); } //get aggregate of all commission splits csAgg = [SELECT SUM(Split_Amount__c),User__c FROM Commission_Split__c WHERE Opportunity__c IN :oppSet]; //get comm splits attached to this opp csList = [SELECT Id,Split_Amount__c,User__c,Opportunity__c FROM Commission_Split__c WHERE Opportunity__c IN :oppSet]; //get users for(Commission_Split__c c:csList){ userSet.add(c.User__r.Id); } //now I have my users in a Set, and aggregate results above, how do I connect? public Map<Id, AggregateResult> userSales = new Map<Id,AggregateResult>([SELECT SUM(Split_Amount__c) FROM Commission_Split__c WHERE User__c IN :userSet)]);
- Jean Grey 10
- June 02, 2018
- Like
- 0
How to clear batch class debug logs at a time
Hi,
I executed batch class and it is executed 60,000 records,batch size is 2 ,So 30,000 logs get created.I want to test it again.
Not able to clear the logs all becuase it is taking more time.
I deleted monitor user and again created user and even I opened the instace with other user in seperate browser still logs are showing.
Can any one suggest me how to delete these debug logs.
Thanks in advance,
I executed batch class and it is executed 60,000 records,batch size is 2 ,So 30,000 logs get created.I want to test it again.
Not able to clear the logs all becuase it is taking more time.
I deleted monitor user and again created user and even I opened the instace with other user in seperate browser still logs are showing.
Can any one suggest me how to delete these debug logs.
Thanks in advance,
- lakshmi.sf9
- November 12, 2014
- Like
- 0